Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial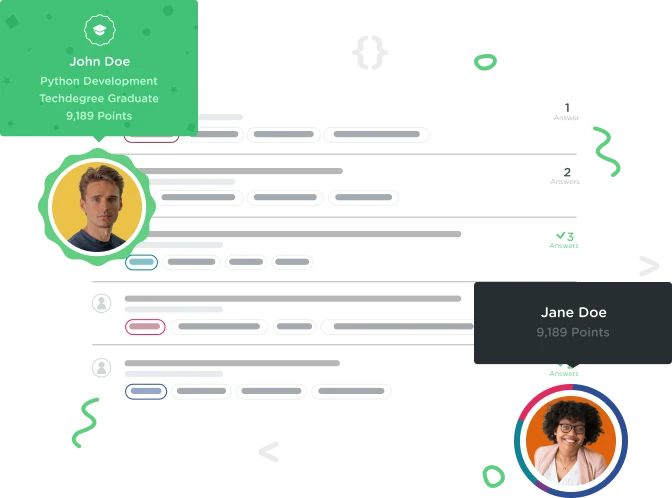
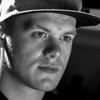
nick schubert
3,535 PointsI feel like there's a gap in what I should know by now
Hey there,
during the Python Collections course when the teacher changed from Craig to Kenneth, I started to feel like there is a big gap in knowledge that I have missed somehow.
Things were going great during Craig's lessons. However, when the teacher switched to Kenneth, I felt like I had become clueless and like there's some fundamental knowledge I had missed out on. For example: during the lessons with Craig everything was explained properly during the video then during the challenge it was challenging, but, I knew the general guidelines of what to do and figure it out. Now during Kenneth's lessons, I noticed that during the code challenges I have to do things that I had no prior knowledge of. I also find myself completely clueless on what to do, resulting in me searching the community forums for answers for every step of the way.
Now to the issue at hand, for this challenge I had the idea to do the following steps:
- make a new empty list to store the tuples into
- make a for loop in both iterables
- (this is where I get stuck) Figure out a way to get the first of both iterables and add them together into a tuple.
- return combo_list
I'm pretty sure I made a mistake in my steps list, I have looked up some community answers and even though I could use their answers to pass the test, I want to truly understand and build experience with what I'm doing.
Sorry for the wall of text, advice is greatly appreciated! I can't wait to tackle this problem and grow!
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(iter1, iter2):
combo_list = []
for iter in iter1, iter2:

Nicholas Wilky
3,973 PointsI totally agree with your thoughts on the Python Collections difficulty. I have had to google for every single coding challenge in dictionaries and tuples. Before that I could trial and error my way to passing the challenge. Have you continued on the beginning python track? Did it get better?
4 Answers
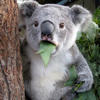
ursaminor
11,271 PointsHere's a solution that uses knowledge that you probably already have. Since they say to assume both iterables are the same length, you can loop over the length of one of them:
# this way you get the index, i, instead of an item from one of the iterables
for i in range(len(iter1)):
To create a tuple of items from the same index in both iterables, you can do:
(iter1[i], iter2[i])
To add it to the empty list, you can use append().
You'll need to put the whole thing above inside the parentheses, so you'll have double parentheses.
Putting that all together:
def combo(iter1, iter2):
result = []
for i in range(len(iter1)):
result.append((iter1[i], iter2[i]))
return result
Sounds like they combined the older lessons by Kenneth with the newer ones by Craig and there are some gaps. I'm sure it's a bit frustrating, but consider that when you're at a real job, you'll have to do things all the time that you don't know how to do and you have to do research. You'll be better prepared than other people who were given all the answers and never had to practice doing that!
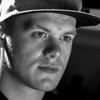
nick schubert
3,535 PointsThank you for the answer! I think I understand it now.
So, just to be clear:
#Since the iterables are both the same size, I can get the index by using either:
for i in range(len(iter1)):
# or
for index, iter in enumerate(iter1):
#Then I can append my list by using:
combo_list.append(iter1[i], iter2[i])
#or
combo_list.append((iter, iter2[index]))
Is this correct? Also, why is it that I have to use (iter,iter2[index]) instead of (iter1, iter2[index])?? I am a bit confused there.
Thanks again!
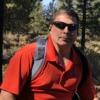
Mark Kastelic
8,147 PointsNick,
Since the challenge says we can assume equal lengths for the iterables, I chose to loop over just one of them while using enumerate to extract the integer index value along with each item. The index can then be used to retrieve the corresponding items from the second iterable while simultaneously combining them in a tuple which is appended to combo_list each time through the loop (see code below).
def combo(iter1, iter2):
combo_list = []
for index, iter in enumerate(iter1):
combo_list.append((iter, iter2[index]))
return combo_list
Once you study Python a little longer, you will eventually come across a handy function called zip( ) which does exactly what combo does:
>>> print(list(zip('abc', 'def')))
[('a', 'd'), ('b', 'e'), ('c', 'f')]
Try it!
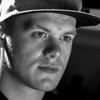
nick schubert
3,535 PointsAh, thank you so much, it works!
However, I would have never figured this out myself and I don't understand what is going on exactly. If I were to encounter a challenge similar to this I still wouldn't be able to know what to do. ( In fact, I still don't. )
for index, iter in enumerate(iter1):
combo_list.append((iter, iter2[index]))
Could you explain to me how these two lines work exactly? I'm familiar with the for loops, but I'm confused as to how it works in this particular instance combining the two iterables together.
Thanks again! I really appreciate the help!
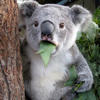
ursaminor
11,271 PointsIn terms of how to problem solve, you got the right idea by breaking the problem down into small steps. When there's a step you don't know how to do, see if you can break it down even further. For example, "Figure out a way to get the first of both iterables and add them together into a tuple" can be further broken down into:
- get the first item from first iterable
- get the first item from second iterable
- put both items into a tuple
When you're not sure at this point, read the documentation to refresh your memory, eg, how to create a tuple. You can always google, but you'll get better results after doing the above steps.
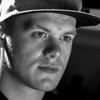
nick schubert
3,535 PointsThat's some great advice, thank you!
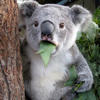
ursaminor
11,271 Points"Why is it that I have to use (iter, iter2[index]) instead of (iter1, iter2[index])?? "
The confusion stems from the name of the variable iter
. It would be more clear and accurate to say item
or something like that.
for index, item in enumerate(iter1):
combo_list.append((item, iter2[index]))
Good variable naming is really important and can help you as well as other developers reading your code.
When you don't know what something is in the code or what it does, try printing:
for index, iter in enumerate(iter1):
print(index, iter)
You'll see that iter
is a value from iter1.
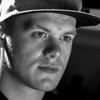
nick schubert
3,535 PointsIt all makes sense to me now, I can't thank you enough!
Mark Kastelic
8,147 PointsMark Kastelic
8,147 PointsP.S.: By placing iter1 and iter2 in the for statement separated by a comma, you are essentially making the for loop through a "list" of iterables (not the individual items in each). To see this, try running the code below: