Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial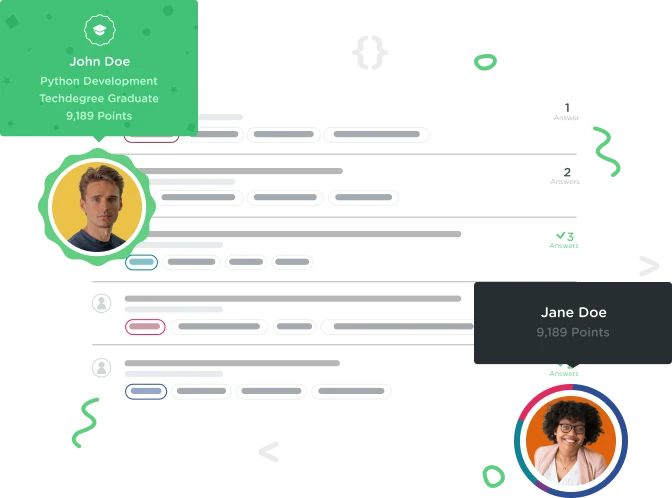

Kishan Gupta
6,567 PointsI finished the challenge but it still says i haven't
Main.java :
package com.teamtreehouse;
import java.io.IOException;
import java.util.List;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
Template tmpl = new Template(prompter.promptForStory());
List<String> validWords = prompter.run(tmpl);
String results = tmpl.render(validWords);
System.out.printf("Your TreeStory:%n%n%s", results);
}
}
Prompter.java :
package com.teamtreehouse;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public List<String> run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
return results;
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) throws IOException{
boolean isCensoredWord;
String word;
do {
System.out.printf("Enter %s : ", phrase);
word = mReader.readLine();
isCensoredWord = mCensoredWords.contains(word);
if(isCensoredWord) {
System.out.printf("%s is not allowed. Please try again. \n", word);
}
} while(isCensoredWord);
return word;
}
public String promptForStory() {
String story = "";
try {
System.out.print("Enter a story template : ");
story = mReader.readLine();
} catch (IOException ioe) {
System.out.println("Error with your input..");
ioe.printStackTrace();
}
return story;
}
}
So the code works properly and even censors the specified word but i am getting this error while submitting the code Bummer: Whoops looks like you didn't censor the words (2a). mCensoredWords contains 'dork', but it made it past somehow.
And It does censors dork.

liamthornback2
9,618 PointsI tried your code and it doesn't work for me either. And I even tested it in Intellij and It works perfectly...
I really hope someone answers this question so I can complete this course...

Zelemkhan Saydullaev
13,028 PointsSystem.out.printf("%s is not allowed. Please try again. \n", word);
the error is thrown because of this line. You are displaying the word that should be censored, if you make this more generic then the error goes away,
System.out.prinln(" That word is not allowed. Please try again.");
2 Answers

Jason Poole
3,123 PointsCraig Dennis tagging.

Daniel Phillips
26,940 PointsWhat if the user input a censored word with a capital letter in it?
Jason Poole
3,123 PointsJason Poole
3,123 PointsI am getting the same error as you, saying dork made it past even though dork does not make it past when i run my code. It's a bug by treehouse IMO