Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial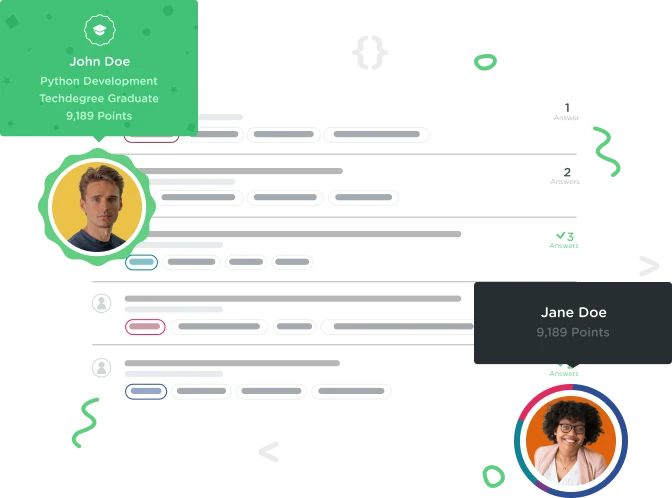
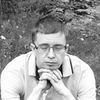
Henrik Granstroem
3,127 PointsI get 22 errors, about not convertable types in my workspace code. I cannot figure out what it can be.
The code is same as in the video, but still it complains about not convertable types. It also complains about the String[] which cannot be converted to string.
Pls help!
6 Answers

Anders Björkland
7,481 PointsHi Henrik!
Even though you follow along with the video, there may still be typos or statements missing that you might have overlooked. If you include your code and what the error message is then it is easier to see what can be done to resolve it.
With that said, a String[] is a different type from String. If you have declared a String variable and try to set a String-array to it, that would not be allowed. Rather, you would loop through the array or get a specific index on the array which would return a String which you can set to your String variable. Here are some examples:
Here is an example that is not allowed
public static void main(String[] args) {
String exampleString = args; // incompatible types, args is of type String[] and cannot be converted to String.
}
Here is an example which is allowed
public static void main(String[] args) {
// Looping through each String in the array args and add it to a String variable.
String exampleString = "";
for (String arg : args) {
exampleString += arg;
}
}
And here is an example of accessing a specific String variable in an array using index.
public static void main(String[] args) {
String exampleString = args[0]; // be aware, will return an ArrayIndexOutOfBoundsException if the array is empty.
}
Hope you figure out what's going on with your code. Please post your code and errors if you need more help.
Good luck!
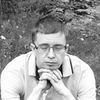
Henrik Granstroem
3,127 PointsHi!
Here I posting my code wich I still cant figure it what went wrong.
package com.teamtreehouse;
import java.util.Date;
public class Treet implements Comparable // Samma namn som filen {
private String mAuthor; private String mDescription; private Date mCreationDate;
public Treet(String Author, String Description, Date CreationDate) {
mAuthor = Author; // ?? = Måste vara lika som argumentlistan
mDescription = Description; // ?? = Måste vara lika som argumentlistan
mCreationDate = CreationDate; // ?? = Måste vara lika som argumentlistan
} @Override public String toString() {
return String.format("Treet: \"%s\" by %s on %s, mDescription, mAuthor, mCreationDate);
}
@Override public int compareTo(Object obj) {
Treet other = (Treet) obj;
if (equals(other))
{
return 0;
}
int dateCmp = mCreationDate.compareTo(other.mCreationDate);
if (dateCmp == 0)
{
return mDescription.compareTo(other.mDescription);
}
return dateCmp;
}
public String getAuthor() { return mAuthor; }
public String getDescription() { return mDescription; }
public Date getCreationDate() {
return mCreationDate;
}
public String[] getWords() {
return mDescription.toLowerCase().split("[^\\w#@']+");
}
}
Regards Henrik

Anders Björkland
7,481 PointsThanks. I will list a couple of things you can look at, in the end of my answer you will see how I would resolve them in code:
It appears like you have commented out the opening curly brace (that is { is in a comment and not a part of the code).
In your toString-method you use a String.format which has an opening quotation mark but not a closing one.
In regards to your parameters' name, name them as any variable, with an initial lowercase, String Author should be String author. It's not something the compiler wouldn't allow, but it's a formality issue.
I don't know how your Example.java looks like (or wherever your main-method is), but remember to import com.teamtreehouse.Treet
So here's how I solved your code:
package com.teamtreehouse;
import java.util.Date;
public class Treet implements Comparable { // the curly brace is now on the right side (that is to the left) of the comment
private String mAuthor;
private String mDescription;
private Date mCreationDate;
public Treet(String author, String description, Date creationDate) { // following the standard of camelCase where initial letters are lowercase
// The variables below are matched with the arguments of the the constructor. You could match the variable mAuthor
// with description, since they are both of type String, but that would just be confusing.
mAuthor = author;
mDescription = description;
mCreationDate = creationDate;
}
@Override
public String toString() {
// added a quotation mark to close the string below
return String.format("Treet: \"%s\" by %s on %s", mDescription, mAuthor, mCreationDate);
}
@Override
public int compareTo(Object obj) {
Treet2 other = (Treet2) obj;
if (equals(other)) {
return 0;
}
int dateCmp = mCreationDate.compareTo(other.mCreationDate);
if (dateCmp == 0) {
return mDescription.compareTo(other.mDescription);
}
return dateCmp;
}
public String getAuthor() {
return mAuthor;
}
public String getDescription() {
return mDescription;
}
public Date getCreationDate() {
return mCreationDate;
}
public String[] getWords() {
return mDescription.toLowerCase().split("[^\\w#@']+");
}
}
If you need help with your Example.java you can post that as well.
Good luck Henrik, and have fun. Any more questions, just ask.
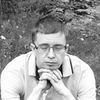
Henrik Granstroem
3,127 PointsAll the course have gone quiet well, till I hit into this errors. I have even sorted out earlier errors before. Just that this time I cant find the logical reasons for this errors since the code is matching the one Craig is doing in the video. I will look through the code with your added comments and try again.
Thanks for helping me! Henrik
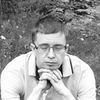
Henrik Granstroem
3,127 PointsAdding that doublequot solved all of it!
public String toString() {
return String.format("Treet: \"%s\" by %s on %s", mDescription, mAuthor, mCreationDate);
}

Anders Björkland
7,481 PointsExcellent. These small errors can be hard to detect. When you will start using an IDE (software on your computer) you will be warned as you type your code, where your error is and sometimes what you can do about it.
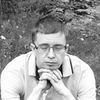
Henrik Granstroem
3,127 PointsYes, I have been trying to work with IDE little before I started here on treehouse. But I wanted to get some good teaching in the programming and get all I need to become what I want. :)

Anders Björkland
7,481 PointsNice, same boat here. ^^