Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial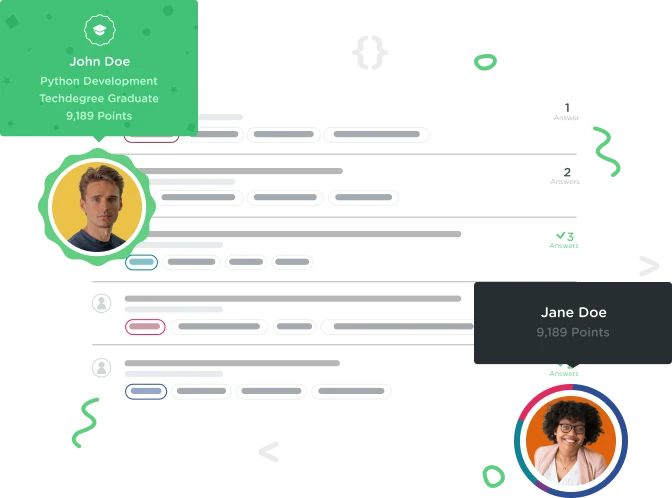

Simen Fossnes
3,226 PointsI get a System.ArgumentNullException on this when I shouldn't.
My code works. The only thing I canged that stopped it from working was adding a while loop in task 3. This created an error in task two, with no apparent change to the code I had in task 2 (a try catch statement with a FormatException).
Here is my code: using System;
namespace Treehouse.CodeChallenges { class Program { static void Main() {
int yays = -1;
while (true)
{
// asks the user for input
Console.Write("Enter the number of times to print \"Yay!\": ");
// takes input, and checks if it's a number or not
try
{
yays = Int32.Parse(Console.ReadLine());
}
catch (FormatException e)
{
Console.Write("You must enter a whole number.");
}
// checks for positive number
if (yays >= 0)
{
break;
}
else
{
Console.Write("You must enter a positive number.");
}
}
var counter = 0;
while (yays > counter)
{
Console.WriteLine("Yay!");
++counter;
}
}
}
}
The error is traced to the Int32.Parse bit of the code. Very strange, as I seem to get it running with no problem in visual studio on my laptop.
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
int yays = -1;
while (true)
{
// asks the user for input
Console.Write("Enter the number of times to print \"Yay!\": ");
// get input from the user
string inputString = Console.ReadLine();
// checks if it's a number or not
try
{
yays = Int32.Parse(inputString);
}
catch (FormatException e)
{
Console.Write("You must enter a whole number.", e);
}
// checks for positive number
if (yays >= 0)
{
break;
}
else
{
Console.Write("You must enter a positive number.");
}
}
// prints the amount of yay's to the console.
var counter = 0;
while (yays > counter)
{
Console.WriteLine("Yay!");
++counter;
}
}
}
}
1 Answer

Simen Fossnes
3,226 PointsOkey, think I found the answer. Looks like the program should be designed to fail if an exception is thrown; not continue. The code testing probably didn't work since the tests are assuming that the program should crash, and then ends up not doing that with a while loop. I also didn't have a way to exit the program, yet that shouldn't be a problem. A program like this would exit befor the user could comprehend all the yay's printed... which is not good UI.