Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial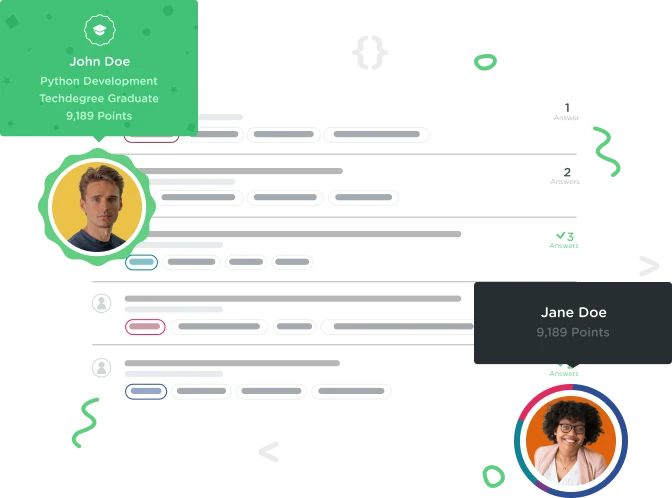
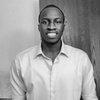
Modou Sawo
13,141 PointsI get a TypeError when I try to remove the list items using parentNode & event.target by clicking....
I get this message saying, "Uncaught TypeError: Cannot read property 'removeChild' of undefined" ==> pointing at the ul.removeChild(li);
line 15.
HTML code:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<button id="toggleList">Hide list</button>
<div class="list">
<p class="description">Things that are purple:</p>
<input type="text" class="description">
<button class="decription">Change list description</button>
<ul>
<li>grapes</li>
<li>amethyst</li>
<li>lavender</li>
<li>plums</li>
</ul>
<input type="text" class="addItemInput">
<button class="addItemButton">Add item</button>
<button class="removeItemButton">Remove last item</button>
</div>
<script src="app.js"></script>
</body>
</html>
Here's my JS code:
const toggleList = document.getElementById('toggleList');
const listDiv = document.querySelector('.list');
const descriptionInput = document.querySelector('input.description');
const descriptionP = document.querySelector('p.description');
const descriptionButton = document.querySelector('button.description');
const addItemInput = document.querySelector('input.addItemInput');
const addItemButton = document.querySelector('button.addItemButton');
const removeItemButton = document.querySelector('button.removeItemButton');
listDiv.addEventListener('click', (event) => {
if (event.target.tagName == 'LI') {
let li = event.target;
let ul = event.parentNode;
ul.removeChild(li);
}
});
1 Answer
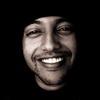
miikis
44,957 PointsHey Modou,
Your reference to the unordered list is incorrect. The unordered list is the parent node of the list item — not the parent node of the Event object. Try const ul = li.parentNode
. That'll make it work for ya.
Modou Sawo
13,141 PointsModou Sawo
13,141 PointsHi Mikis, Thanks man! It worked.
While here, can you please look at line 51, I get a "Uncaught TypeError: Cannot read property 'addEventListener' of null":
miikis
44,957 Pointsmiikis
44,957 PointsYou're welcome sir. You've probably figured it out by now but the reason that
addEventListener
isn't attaching todescriptionButton
is because you have a typo: in your HTML, you spelled thebutton
's class-name wrong. Fix that and it'll work ;)