Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial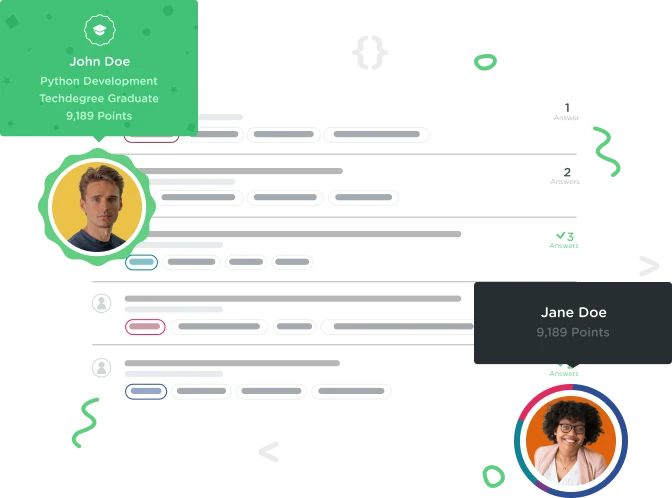

Kevin Gates
15,053 PointsI get an undefined error first, then I get the proper response (200) right afterward. Any ideas as to why?
app.js
// Problem: We need a simple way to look at a user's badge count and JavaScript points
// Solution: Use Node.js to connect to Treehouse's API to get profile information to print out
const profile = require('./profile.js');
const users = process.argv.slice(2)
users.forEach(profile.get);
profile.js
//Require https module
const https = require("https");
// Require http module for status codes
const http = require("http");
//Print Error Messages
function printError(error) {
console.error(error.message);
}
// Function to print message to console.
function printMessage(username, badgeCount, point) {
const message = `${username} has ${badgeCount} total badge(s) and ${point} points in JavaScript`;
console.log(message);
}
function get(username) {
try {
// Connect to the API URL (https://teamtreehouse.com/username.json)
const request = https.get(`https://teamtreehouse.com/${username}.json`, (response) => {
if (response.statusCode === 200 ) {
let body = "";
// Read the data
response.on('data', data => {
body += data.toString();
});
response.on('end', () => {
try {
// Parse the data
const profile = JSON.parse(body);
// Print the data
printMessage(username, profile.badges.length, profile.points.JavaScript);
} catch (error) {
printError(error);
}
});
} else {
const message = `There was an error getting the profile for ${username} (${http.STATUS_CODES[response.statusCode]})`;
const statusCodeError = new Error(message);
printError(statusCodeError);
}
}).on('error', printError(error))
} catch (error) {
printError(error);
}
}
module.exports.get = get;
the code in node that I run is
node app.js romerican
I get back:
error is not defined
romerican has 108 total badge(s) and 2844 points in JavaScript
I've test it, the area that is first showing the undefined error is the catch could in the main function get. What I don't understand is why it first fails, then works...without being called twice.
Any insight is appreciated.
1 Answer

Vitaly Khe
7,160 PointsYou have not passed error to a printError function in 44 string of your code. Pls include passing the error to it like :
}).on('error', error => printError(error))
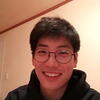
Seokhyun Wie
Full Stack JavaScript Techdegree Graduate 21,606 PointsThanks for your answer. It really helped.
Gari Merrifield
9,598 PointsGari Merrifield
9,598 PointsI would look closely at what is actually happening in your app.js. slice of 2, then a foreach, would suggest that it may be getting called twice. I haven't dug into your code deeply, but that is where I would start, and maybe log what is actually getting into your users const.