Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial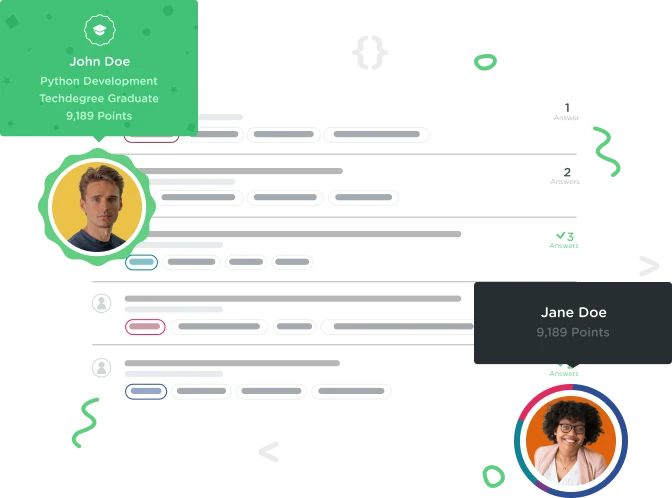

taha siddiqui
6,222 PointsI get my items duplicated from the List View Holder
When I open it at first, the list looks fine but then when I scroll down, there's duplicated items. So the items in their top most (unscrolled state) become the background as I scroll to get new items in the List View
This is my adapter
public class ListAdapter extends RecyclerView.Adapter {
@NonNull
@Override
public RecyclerView.ViewHolder onCreateViewHolder(@NonNull ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.list_item, parent, false);
return new ListViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull RecyclerView.ViewHolder holder, int position) {
//this is where we update the view and view group to reflect what position it is
//on the screen
((ListViewHolder) holder).bindView(position);
}
@Override
public int getItemCount() {
return Recipes.names.length;
}
//holds all our views
private class ListViewHolder extends RecyclerView.ViewHolder implements View.OnClickListener{
private TextView mTextView;
private ImageView mImageView;
public ListViewHolder(@NonNull View itemView) {
super(itemView);
mTextView = itemView.findViewById(R.id.itemText);
mImageView = itemView.findViewById(R.id.itemImage);
//ssets the item view's on click listener to the view group
itemView.setOnClickListener(this);
}
public void bindView(int position){
mTextView.setText(Recipes.names[position]);
mImageView.setImageResource(Recipes.resourceIds[position]);
}
@Override
public void onClick(View v) {
}
}
}
This is my List Fragment class
public class ListFragment extends Fragment {
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_list, container, false);
RecyclerView recyclerView = view.findViewById(R.id.listRecyclerView);
ListAdapter listAdapter = new ListAdapter();
//In Android development, any time we want to show a vertical list
// of scrollable items we will use a ListView which has data
// populated using an Adapter
recyclerView.setAdapter(listAdapter);
//layout manager is responsibe for determining where to place the views
//to make recycler view act like a vertical list, use linearManager class
RecyclerView.LayoutManager layoutManager = new LinearLayoutManager(getActivity());
recyclerView.setLayoutManager(layoutManager);
return view;
}
}
This is my main activity
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ListFragment fragment = new ListFragment();
//fragment manager helps us keep track of fragments, manage back stack,
//have access to fragment transaction API used to add/remove fragments
//remember to use the android.app package for this
FragmentManager fragmentManager = getFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
fragmentTransaction.add(R.id.placeHolder, fragment);
//transactions are like shared prefs where u need to commit
fragmentTransaction.commit();
}
}