Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial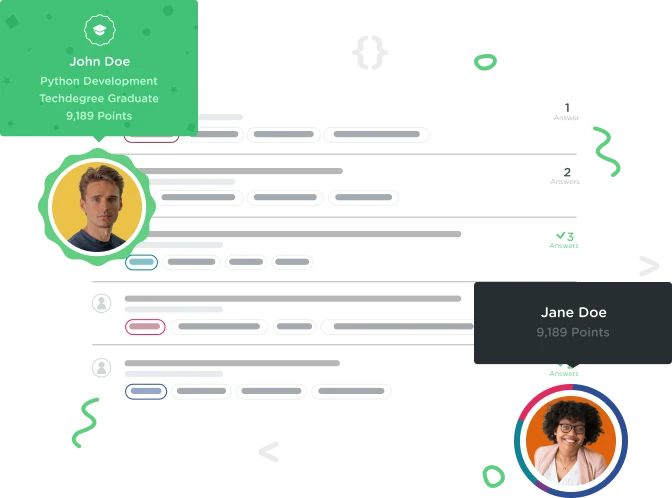

Donald Davis
10,588 PointsI got a little too brave and tried this. When I ran it through IDLE, it showed me removing str and bool, but not list.
I got a little too brave and tried this. When I ran it through IDLE, it showed me removing str and bool, but not list.
the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
# Your code goes below here
the_list.insert(0, the_list.pop(3))
for item in the_list:
if isinstance(item, str) or isinstance(item, bool) or isinstance(item, list):
the_list.remove(item)
1 Answer

Jason Anello
Courses Plus Student 94,610 PointsHi Donald,
You experienced what could happen when you are iterating over a list and modifying it at the same time.
When you get to the "a" and remove it, everything that comes after it moves down 1 index to take up the missing space.
On the next loop, you should be looking at the 2 but you're looking at the 3 instead since it moved to where the 2 used to be. You've skipped over the 2.
Eventually you get to the boolean value and you remove it. The list then moves down to occupy that space and you skip over it. The for loop ends because there are no more items.
It's recommended that you iterate over a copy of the list while you modify the original list to avoid this type of problem.
You can use slice notation to make a copy of the list like this: the_list[:]
Here's some output from the python shell:
>>> the_list = ["a", 2, 3, 1, False, [1, 2, 3]]
>>> the_list.insert(0, the_list.pop(3))
>>> for item in the_list[:]:
... if isinstance(item, str) or isinstance(item, bool) or isinstance(item, list):
... the_list.remove(item)
...
>>> the_list
[1, 2, 3]