Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial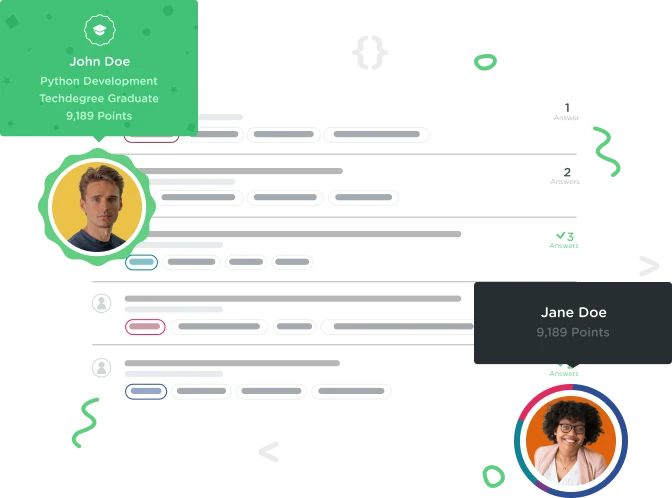

Eirin Joahna Pamela Gonzales
8,686 PointsI got an error: for-each not applicable to expression type
What does this mean?
package com.example;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
public class BlogPost implements Comparable<BlogPost>, Serializable {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
public int compareTo(BlogPost other) {
if (equals(other)) {
return 0;
}
return mCreationDate.compareTo(other.mCreationDate);
}
public String[] getWords() {
return mBody.split("\\s+");
}
public List<String> getExternalLinks() {
List<String> links = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
links.add(word);
}
}
return links;
}
public String getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mCategory;
}
public Date getCreationDate() {
return mCreationDate;
}
}
package com.example;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
import java.util.Map;
import java.util.HashMap;
public class Blog {
List<BlogPost> mPosts;
public Blog(List<BlogPost> posts) {
mPosts = posts;
}
public List<BlogPost> getPosts() {
return mPosts;
}
public Set<String> getAllAuthors() {
Set<String> authors = new TreeSet<>();
for (BlogPost post: mPosts) {
authors.add(post.getAuthor());
}
return authors;
}
public Map<String, Integer> getCategoryCounts() {
Map<String, Integer> allCategories = new HashMap<String, Integer>();
for (BlogPost post: mPosts) {
for (String category : post.getCategory()) {
Integer ctr = allCategories.get(category);
if (ctr == null) {
ctr = 0;
}
ctr++;
allCategories.put(category, ctr);
}
}
return allCategories;
}
}
2 Answers

Dan Johnson
40,533 PointsThe String
class is not an Iterable
in Java so it can't be used in a for-each loop. In other standard libraries for other languages where strings are iterables this would generally have a for-each pull out each character individually which isn't what you'd want to deal with in this case anyways.
With the nested for-each out of the way we can grab the category like this instead:
// Grab from each Post
String category = post.getCategory();

Eirin Joahna Pamela Gonzales
8,686 PointsThanks Dan! I already solved it! I removed the inner for loop then, I used that statement. Thanks! :)

Chirag jhumkhawala
1,212 PointsHad the same issue, worked out for me as well.!! thanks