Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial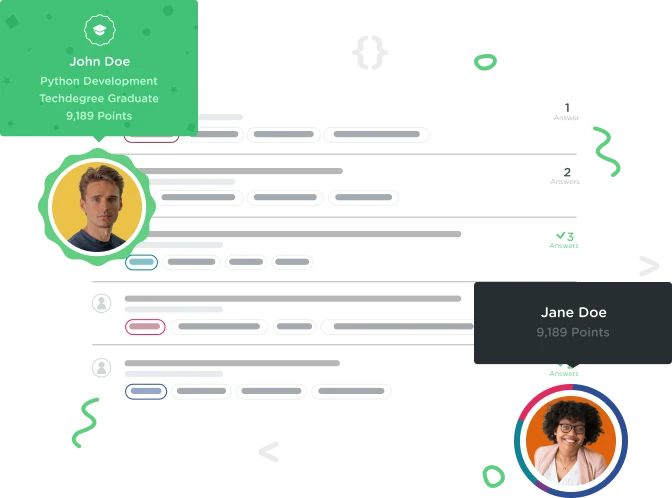

Chang Hyeon Lee
2,008 PointsI got invalid syntax error
I had a monster.py import random
from combat import Combat
COLORS = ['yellow', 'red', 'blue', 'green']
class Monster(Combat): min_hit_points = 1 max_hit_points = 1 min_experience = 1 max_experience = 1 weapon = 'sword' sound = 'roar'
def __init__ (self, **kwargs):
self.hit_points =random.randint(self.min_hit_points, self.max_hit_points)
self.experience =random.randint(self.min_experience, self.max_experience)
self.color = random.choice(COLORS)
for key, value in kwargs.items():
setattr(self, key, value)
def __str__(self):
return '{} {}, HP:{}, XP: {}'.format(self.color.title(),
self.__class__.__name__,
self.hit_points,
self.experience)
def battlecry(self):
return self.sound.upper()
class Goblin(Monster): max_hit_points = 3 max_experience = 2 sound = 'hob'
class Troll(Monster): max_hit_points = 5 min_hit_points = 3 min_experience = 2 max_experience = 6 sound = 'hows'
class Dragon(Monster): max_hit_points = 8 min_hit_points = 4 min_experience = 5 max_experience = 2 sound = 'grall'
character.py: from combat import Combat
class Character(Combat): experience = 0 hit_points = 10
def get_weapon(self):
weapon_choice = input("Weapon ([S]word, [A]xe, [B]ow): ").lower()
if weapon_choice in 'sab':
if weapon_choice == 's':
return 'sword'
elif weapon_choice == 'a':
return 'axe'
else:
return 'bow'
else:
return self.get_weapon()
def __init__(self, **kwargs):
self.name = input("Name: ")
self.weapon = self.get_weapon()
for key, value in kwargs.items():
setattr(self, key, value)
and combat.py: import random
class Combat: dodge_limit = 6 attack_limit = 6
def dodge(self): roll = random.randint(1, self.dodge_limit) retrun roll > 4
def attack(self): roll = random.randint(1, self.attack_limit) return roll > 4
and then I typed (from combat import Combat), I got syntax error message
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/home/treehouse/workspace/monster.py", line 3, in <module>
from combat import Combat
from combat import Combat
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "/home/treehouse/workspace/combat.py", line 10
retrun roll > 4
^
SyntaxError: invalid syntax
What did I wrong? and then if I see this error message, how can I fix this?
Thank you.
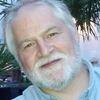
Jeff Muday
Treehouse Moderator 28,724 PointsOne error here is that you misspelled "return" as "retrun". I hope that's it since that is easy to fix.
Leonard Bode is right, that you would be better served by putting your python in a code block with syntax coloring. The keywords should be colored orange and misspelled keyword is in white.
return roll > 4 # this works
retrun roll > 4 # this does not work
Good luck in all your Treehouse courses! JM

Doc Collins
7,087 PointsYou typed: retrun roll > 4
It should have been: return roll > 4
leonardbode
Courses Plus Student 4,011 Pointsleonardbode
Courses Plus Student 4,011 PointsChang, when posting Python code in forums use the following syntax:
```python
(Your code goes here)
```
For example:
```python
COLORS = ['yellow', 'red', 'blue', 'green']
```
This would look like this:
COLORS = ['yellow', 'red', 'blue', 'green']
Please edit your question with this syntax and I will help you.