Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial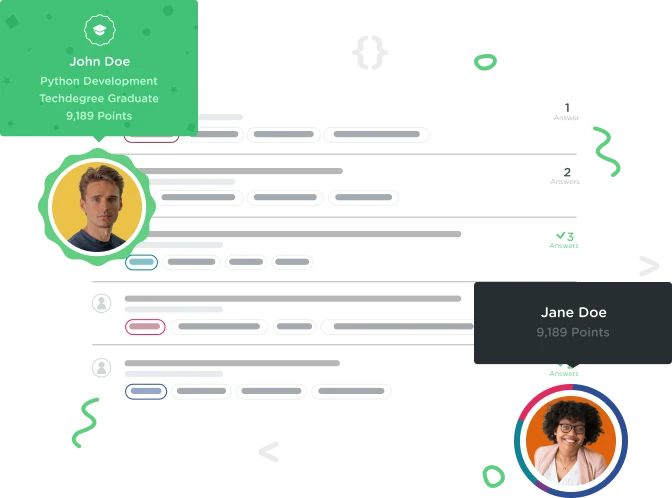

Blair Walker
12,672 PointsI got it to work perfectly, but instead of "and" I had "or". Why did this work but the "and" didn't?
name = input("Please enter your name: ")
number = int(input("Please enter a number: "))
print(name)
print(number)
is_fizz = (number % 3)
is_buzz = (number % 5)
if (is_fizz or is_buzz) == int(0):
print("is a FizzBuzz number.")
elif is_fizz == int(0):
print("is a Fizz number.")
#elif is_buzz == int(0):
#print("is a Buzz number.")
else:
#print("is neither a fizzy or a buzzy number")
1 Answer
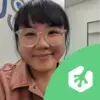
Rachel Johnson
Treehouse TeacherHey Blair Walker , thanks for posting in the community!
To understand what's happening, we must get into the weeds of and
, or
, and what they produce when we supply integers on either side of it.
-
and
will return the last number given.1 and 2 and 3
will return3
-
or
will return the first number given.1 or 2 or 3
will return1
With that knowledge, let's have a look at the conditional
Let's say the number inputted is 15.
-
is_fizz
will be 0 -
is_buzz
will also be 0 -
is_fizz or is_buzz
andis_fizz and is_buzz
will produce 0, so the conditional is true, and we get theFizzBuzz
output
Now let's say the number inputted is 5, which should not trigger FizzBuzz
-
is_fizz
will be 2 -
is_buzz
will be 0 -
is_fizz or is_buzz
will return 2, which in turn does NOT triggerFizzBuzz
-
is_fizz and is_buzz
will return 0, which DOES triggerFizzBuzz
So, we'll actually want to test if is_fizz
or is_buzz
themselves are equal to 0, rather than a combination of the two.
name = input("Please enter your name: ")
number = int(input("Please enter a number: "))
print(name)
print(number)
is_fizz = (number % 3)
is_buzz = (number % 5)
if (is_fizz == int(0) and is_buzz == int(0)):
print("is a FizzBuzz number.")
elif is_fizz == int(0):
print("is a Fizz number.")
elif is_buzz == int(0):
print("is a Buzz number.")
else:
print("is neither a fizzy or a buzzy number")
I hope this helps!
Blair Walker
12,672 PointsBlair Walker
12,672 PointsHi, thanks so much for your answer! I am a little confused still though about 'and, or' Let's take this for example:
if (is_fizz == int(0) and is_buzz == int(0)):
You said that 'and' will return the last number given. Would this mean for the above line of code, that only is_buzz is being returned? Maybe I'm confusing what 'return' means in this situation.
Rachel Johnson
Treehouse TeacherRachel Johnson
Treehouse TeacherHey Blair Walker !
and
will return the last number given if the elements are numbers. In your case,if (is_fizz == int(0) and is_buzz == int(0)):
is now comparing Booleans.true and true
will returntrue
true and false
,false and true
, andfalse and false
will all returnfalse
"return" in this context just means what the expression/statement will evaluate to. Ie
true and true
will returntrue
.