Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial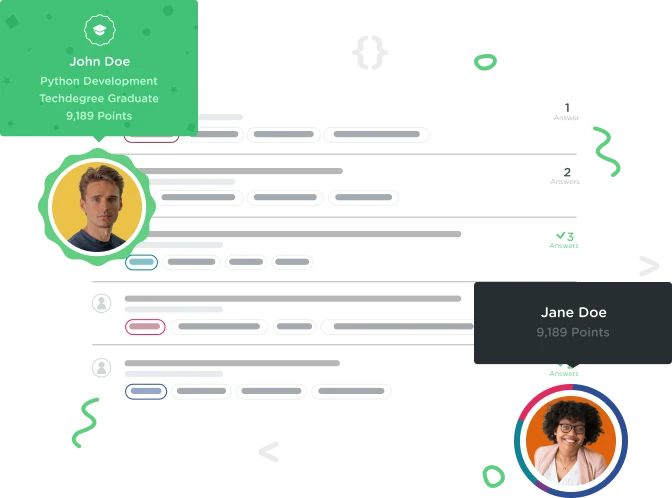

orange sky
Front End Web Development Techdegree Student 4,945 PointsI got "" on a prototype parameter
Hello,
I am not passing a name or mood to greet() in personPrototype, but in Firefox webconsole, when I type: console.log(Jim.greet['name']), I get "".
I thought the empty string only applied to when 'this' does not have a value; hence it takes on the Window global variable.
In the code below, my object called Jim has a name value called 'Jim'. Why would the Jim prototype greeting method return an empty space instead of undefined for Jim.greet['name']?
In fact the console shows undefined for Jim.greet['mood'], but it returns a "" for Jim.greet['name']. What does that mean? Cheers!!
<!DOCTYPE html>
<head>
</head>
<body>
<script>
var personPrototype={
name : "Anonymous",
greet : function(name, mood){
name = name || " You ";
mood = mood || " good ";
console.log("Hello " + name +
" I am " + this.name +
" and I am in a " + mood + " mood ! ");
}
};
function Person (name ){
this.name = name;
}
Person.prototype = personPrototype;
Jim = new Person("Jim");
//Please open up a console and type:
//Jim.greet['name'] then Jim.greet['mood']
</script>
</body>
</html>
5 Answers

Dino Paškvan
Courses Plus Student 44,108 PointsIn your scenario, you are not accessing the properties of the Jim object, you are accessing properties of the .greet()
method.
The method obviously doesn't have a mood
property, which is why you're getting undefined
for it.
But under the upcoming ECMAScript 6 standard of JavaScript (which has been partially implemented into some browsers), all functions have a name
property. That's what you are accessing through Jim.greet['name']
. Now, the greet
property references an anonymous (nameless) function, so that's why you get an empty string.
You could name your method and see that you'd get a different result:
var personPrototype={
name : "Anonymous",
greet : function greetingMethod(name, mood) { // notice that this function is actually a named function
name = name || " You ";
mood = mood || " good ";
console.log("Hello " + name + " I am " + this.name + " and I am in a " + mood + " mood ! ");
}
};
Now if you were to access the name
property, you would get the string "greetingMethod"
.

orange sky
Front End Web Development Techdegree Student 4,945 PointsHello Dino, hmm Yes, I understand that I am trying to access the properties of the greet method, but when I do. jim.greet(); my webconsole displays the placeholder values that we gave it with: name = name || 'you' and mood = mood || ' good'. Anyway, my webconsole shows exactly this:
Jim.greet(); undefined "Hello You I am Jim and I am in a good mood ! " toolTry.html:15 Jim.greet['name'] "" Jim.greet['mood'] undefined
In the setence, it prints 'Hello You....' so why doesnt it print 'you' (the placeholder value) when I try to access it individually with Jim.greet['mood']. The lecture video says that I should only get an empty tring if 'this.name' does not have a value when I create my object 'Jim'. I even find it peculiar that Jim.greet['mood'] returns undefined, when it should return, the placeholder value, 'good'.
thanks!!

Dino Paškvan
Courses Plus Student 44,108 PointsI think you misunderstood me. You are trying to access properties of a method that don't exist (mood
). Or properties which are something completely different than what you think they are (name
).
Basically, you are trying to access parameters of a function (method), outside of that function, but that's not how JavaScript (and most programming languages) works.
A function does not retain the values that were passed into it as arguments. It only has access to them during the execution of that particular function call.
Once the function does its job, you can't access those parameters. They are not properties.
Take this example:
function outputText(text) {
console.log("I got this text: " + text);
}
Now, when you call this function, it will output "I got this text: "
followed by the string that you've passed to it.
That string cannot be retrieved after the function is done logging the text.
outputText("This is a test"); // outputs "I got this text: This is a test
outputText['text']; // this is undefined
outputText.text; // as is this
The dot notation and the bracket notation are a way to access properties, not parameters. The parameters of a function are only available inside the function while it is being executed.
You could even set the text
property of a function to something, but that wouldn't have anything to do with the parameter of the function.
outputText['text'] = "Some string";
outputText['text']; // "Some string"
outputText("Some other string"); // I got this text: Some other string
Properties of functions and their parameters are completely unrelated.

orange sky
Front End Web Development Techdegree Student 4,945 PointsHello Dino, yes you are right, I misunderstood you; in fact I didnt know this. Ok, let me make sure I have the ABC on this right.
1)When I have an object literal, the reaon I can print,the value of the key is because the key is considered a property of that Object, right? Something like this:
var Jim ={name : 'jim', sport :'baseball'}; console.log(Jim.name) returns 'jim'
2) As for this below, I am tempted to call it an object because we use it as a prototype? You mentionned that parameters in a function take in the values that are passed, and once executed these parameters are no longer accessible. Wait, I think I am comparing apples and oranges. Ok, I just hit that brick wall again. The personPrototype and the object literal Jim have similar construct, why can't the console also display these values (Anonymous, You, good) Sorry for not totally getting it, but I am on the right tracks. Thanks for your help!!
var personPrototype={
name : "Anonymous",
greet : function(name, mood){
name = name || " You ";
mood = mood || " good ";
console.log("Hello " + name +
" I am " + this.name +
" and I am in a " + mood + " mood ! ");
}

Dino Paškvan
Courses Plus Student 44,108 PointspersonPrototype
is also an object, but its properties are name
and greet
, not name
and mood
.
name
and mood
are function parameters for the function (method) that greet
points at. You could take the function out of the object, so you'd see that its parameters have nothing to do with the object:
function universalGreet (name, mood) { // a universal greet function
name = name || " You ";
mood = mood || " good ";
console.log("Hello " + name + " I am " + this.name + " and I am in a " + mood + " mood ! ");
}
var personPrototype = {
name : "Anonymous",
greet : universalGreet // reference the universal greet function
}

orange sky
Front End Web Development Techdegree Student 4,945 Pointsgot it !!!!

orange sky
Front End Web Development Techdegree Student 4,945 PointsHello!
I will come back to this post as soon as I am done going over these lessons and trying them out in the console. There is something that I am unsure of. I will be back! Cheers!