Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial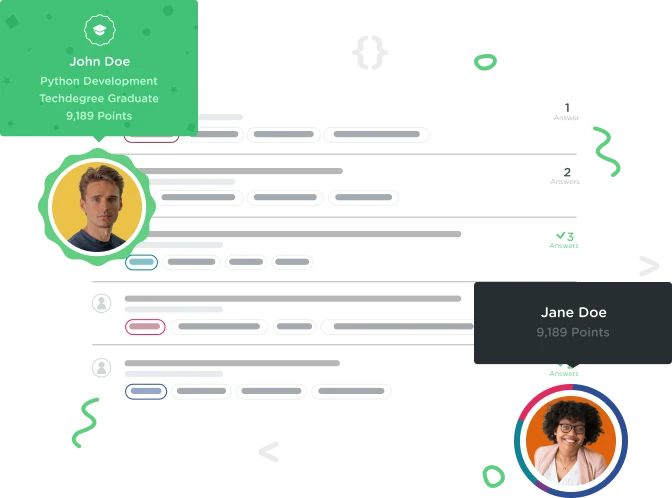

Louis Delgado
10,182 PointsI got stuck! how do I make it so that it outputs the correct info?
I need to figure out the best way to make this return the last else statement.
var money = 9;
var today = 'Friday'
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( money < 9 && 1 < money ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
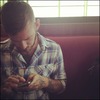
Erik McClintock
45,783 PointsLouis,
Based on the rules presented and the logic, we'll need to make two changes to our conditional checks in order to print out the final else if
block.
1) Our conditionals throughout are not checking for the appropriate combination of values. We only want to run a given code block if two conditions are met: a) we have X amount of money or greater, AND b) it is Friday. Currently, each conditional is checking OR between those two conditions, not AND.
var money = 9;
var today = 'Friday';
// notice that each if/else if check below is checking for a dollar amount OR the day of Friday (using the || operator)
if ( money >= 100 || today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 || today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 || today === 'Friday' ) {
alert("Time for a movie");
} else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
So firstly, we need to change the || operators to && operators, to make sure each check is looking for the both of those conditions to be true before allowing its code block to be run.
2) We want the final else if
block to run if, ultimately, the value in our money variable is not greater than or equal to the lowest possible amount (in this case, 10), but if the day is still indeed Friday. Currently, the final else if
condition is checking that it is NOT Friday. We need to check that it IS Friday.
// note that this final else if check is evaluating to true if it is NOT Friday (using the !== operator)
} else if ( today !== 'Friday' ) {
alert("It's Friday, but I don't have enough money to go out");
}
We need to switch that check from using the !== operator to using the === operator.
Once you have both of those changes in place, you'll be good to go!
Erik

Chase Marchione
155,055 Points1) You'll want to change all of the OR logic operators to be AND logic operators. (in other words, change the ||s to &&s). The reason why: the if and first 2 else if statements are too easily satisfied. They pass if you have enough money OR if the day is Friday, and what we need is for the tests to pass only if we have the money AND the day is Friday.
2) We should only expect to see "It's Friday, but I don't have enough money to go out" if the day is indeed Friday, but we have 10 or less stored in the money variable. Since we can logically deduce that we have 10 or less if we get to that test, we only need to check for the day.
var money = 9;
var today = 'Friday'
if ( money >= 100 && today === 'Friday' ) {
alert("Time to go to the theater");
} else if ( money >= 50 && today === 'Friday' ) {
alert("Time for a movie and dinner");
} else if ( money > 10 && today === 'Friday' ) {
alert("Time for a movie");
} else if ( today === 'Friday') {
alert("It's Friday, but I don't have enough money to go out");
} else {
alert("This isn't Friday. I need to stay home.");
}
Hope this helps!
Justin Light
10,478 PointsJustin Light
10,478 PointsA few things in this one, first you should use && in place of || in the first 2 else if statements. Also in the final else if statement you will need to check for Friday, you don't need to worry about the money as it has already been checked in the first few statements.