Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial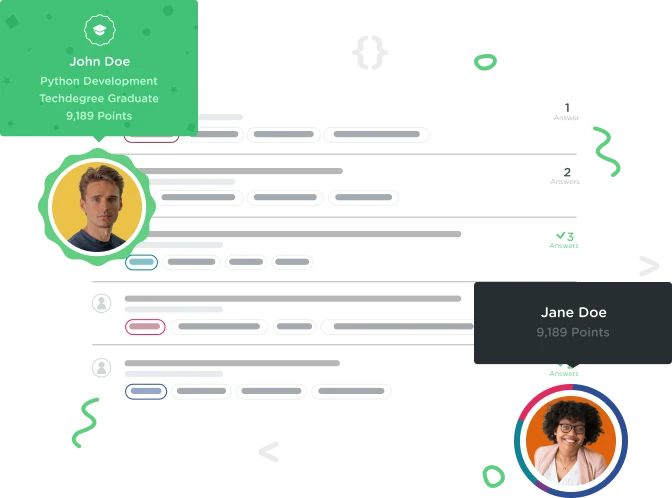
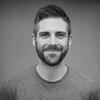
Daniel Cranney
11,609 PointsI got the right answer for numberOf503 challenge, but it feels like a cheat. How else could I do it?
So here's my solution for the numberOf503 challenge. It gives the right result (3), but it only really checks for a 5 at index 1, and a 3 at index 3. If the number at index 2 was different, it would still get added to my reduce result.
How else could I have done this, in a more reliable way?
const phoneNumbers = ["(503) 123-4567", "(646) 123-4567", "(503) 987-6543", "(503) 234-5678", "(212) 123-4567", "(416) 123-4567"];
let numberOf503;
// numberOf503 should be: 3
// Write your code below
numberOf503 = phoneNumbers.reduce( (count, number) => {
if(number[1] === '5' && number[3] === '3') {
return count + 1
}
return count;
}, 0);
console.log(numberOf503);
1 Answer
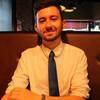
Cody Hansen
5,517 PointsThere are a few ways to answer this! If you're just looking for numbers that start with 503, you could use substrings or regular expressions!
With substrings, instead of looking for "5" at index 1 and "3" at index 3, you would just be looking for "(503)" at the start of the string. Like this!:
let numberOf503;
// numberOf503 should be: 3
// Write your code below
numberOf503 = phoneNumbers.reduce( (count, number) => {
if(number.substring(0,5) === "(503)") {
return count + 1;
}
return count;
}, 0);
This webpage goes over the method very thoroughly if you would like some extra reading: https://www.w3schools.com/jsref/jsref_substring.asp
With regular expressions, you're also looking for "503" at the beginning of the string by using a list of rules to follow. Like this!:
let numberOf503;
// numberOf503 should be: 3
// Write your code below
const regex = /\(?503.*/m;
numberOf503 = phoneNumbers.reduce((count, phone) => {
if(regex.test(phone)) {
return count += 1;
}
else {
return count;
}
}, 0);
I would go more into regular expressions, but they are a different beast of rules all on their own. There are courses here on Treehouse about them. I highly recommend them! They are very useful.
I hope this helps!
Daniel Cranney
11,609 PointsDaniel Cranney
11,609 PointsThanks Cody Hansen, I knew the solution would likely be a simple one but I didn't think to use substring in that way for some reason. Great solution, really helpful and well explained too!