Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial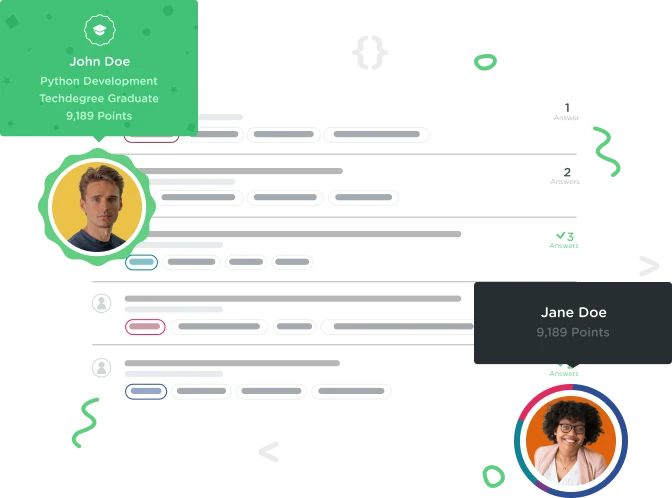
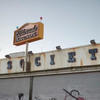
Vic A
5,452 Pointsi got this right, but..
i got this right but I wanted to know of a more concise way of writing it. I'm bad when it comes to short simple code
# EXAMPLES:
# move((1, 1, 10), (-1, 0)) => (0, 1, 10)
# move((0, 1, 10), (-1, 0)) => (0, 1, 5)
# move((0, 9, 5), (0, 1)) => (0, 9, 0)
def move(player, direction):
x, y, hp = player
xd, yd = direction
x, y = (x + xd, y + yd)
if x < 0:
hp -= 5
x += 1
wrong()
elif x > 4:
hp -= 5
x -= 1
wrong()
elif y < 0:
hp -=5
y += 1
wrong()
elif y > 4:
hp -= 5
y -= 1
return x, y, hp
4 Answers
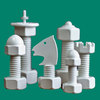
Steven Parker
231,169 PointsI'm a bit surprised that passed. There seem to be some errors:
- the challenge said the dimensions were 0 to 9, but you're limiting movement to 0 to 4
- the challenge didn't ask for a function named wrong to be called
But other than fixing these errors, you could make the code a bit more compact by assigning values in your tests similarly to how you assigned x and y:
hp, x = (hp - 5, x + 1)

Zach Waring
6,895 PointsHere is what i came up with.
def move(player,direction):
x,y,hp = player
xd,yd = direction
r = range(0,9)
new_x, new_y = (x + xd, y + yd)
if new_x not in r or new_y not in r:
hp -=5
return x,y,hp
return new_x, new_y, hp
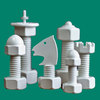
Steven Parker
231,169 PointsVery concise! Good job.

agzagi
2,399 PointsThis is awesome. Very nice answer! The best one I've have seen so far.
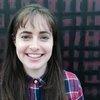
Lois Shedd
1,126 PointsDoes this address the issue of not letting the player walk into the walls? I may be wrong, but it looks like it returns new_x and new_y even if that means they would move past the walls.

agzagi
2,399 PointsDear Lois Shedd , as far as I can tell, this solution does address the issue of not allowing the player to move beyond the walls. Take a look at the "if": if the "if" conditions are met, then the function returns the original x and y, and subtracts 5 from the hp. This "return x,y,hp" is nested at the end of the "if" section.
By returning the original x and y you leave the player at the position he was before attempting the invalid move, while decreasing his hp.
Only if the "if" conditions are not met will the function return new_x and new_y, which is nested outside the "if" section.
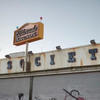
Vic A
5,452 PointsYea, it seems to work with 4 or 9. I added a prompt in a seperate ide i was working in and forgot to remove that when i copied it over. Ok great, thanks
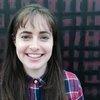
Lois Shedd
1,126 PointsI took a different approach by invalidating certain moves off the bat, for both x and y axes. Then all I had to do was check against this tuple to see whether the move was valid - if it was, I made it happen, and if not, I put the hurt on! Like so:
def move(player, direction):
x, y, hp = player
move_x, move_y = direction
invalid_moves = ((0, -1), (9, 1))
if (x, move_x) in invalid_moves:
hp -= 5
else:
x += move_x
if (y, move_y) in invalid_moves:
hp -= 5
else:
y += move_y
return x, y, hp
I don't know if it's actually that much more concise now that I'm looking at it, but I think perhaps it might be more readable ...
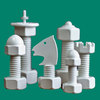
Steven Parker
231,169 PointsIf the area were not square, you'd need separate invalid_moves_x and invalid_moves_y.
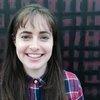
Lois Shedd
1,126 PointsTrue - good point. That would have changed my approach, I think, in terms of keeping things concise.