Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial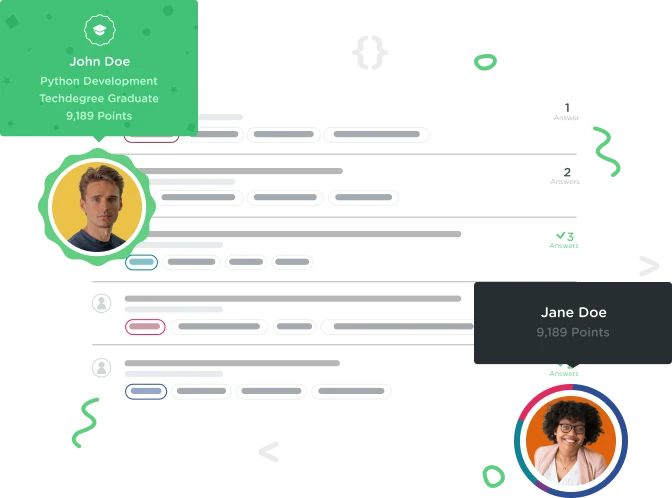

Bill Leatherman
1,451 PointsI had ChatGBT rewrite my code to include error handling.. This is what it gave.. Nice and clean
# Create an empty list to maintain the player names
players_names = []
# Function to handle adding players
def add_players():
while True:
add_players_input = input("Would you like to add players to the list? (Yes/No?) ").lower()
if add_players_input == "yes":
player_name = input("Enter the name of the player to add to the team: ")
players_names.append(player_name)
elif add_players_input == "no":
break
else:
print("Invalid input! Please enter 'Yes' or 'No'.")
# Function to safely select the goalkeeper
def select_goalkeeper():
while True:
try:
keeper = int(input(f"Please select the goalkeeper by entering the player's number (1-{len(players_names)}): "))
if 1 <= keeper <= len(players_names):
return keeper
else:
print(f"Please select a valid number between 1 and {len(players_names)}.")
except ValueError:
print("Invalid input! Please enter a number.")
# Call the function to add players
add_players()
# Print the number of players on the team
print(f"\nThere are {len(players_names)} players on the team")
# Print the player number and the player name
for i, player in enumerate(players_names, 1):
print(f"Player {i}: {player}")
# Safely select the goalkeeper
keeper = select_goalkeeper()
# Print the goalkeeper's name
print(f"The goalkeeper's name is {players_names[keeper - 1]}!")
1 Answer
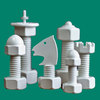
Steven Parker
230,946 PointsWhen posting code, always use Markdown formatting to preserve the code's appearance and retain special symbols. Check out this video about how to do code formatting.
An even better way to share code and make your issue easy to replicate is to make a snapshot of your workspace and post the link to it here. Here's a video that explains how to make a workspace snapshot.