Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial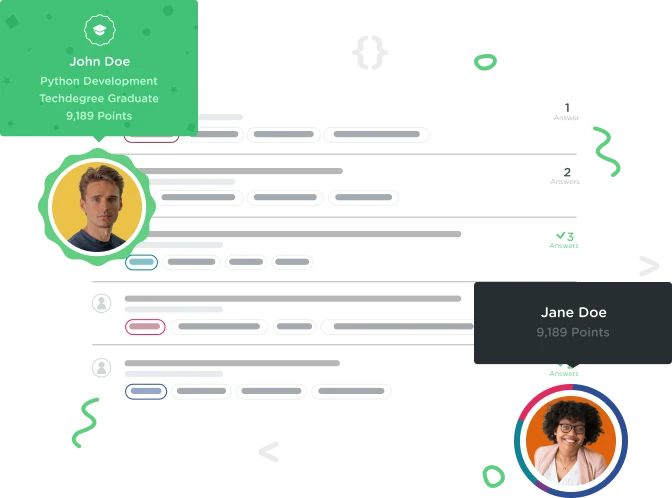
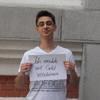
Placinta Alexandru
3,011 PointsI have 15 errors and i do not know why
This is the anntation Doc.java package com.teamtreehouse.docgen;
import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; import java.lang.annotation.Target; import java.lang.annotation.ElementType;
@Retention( value = RetentionPolicy.RUNTIME ) @Target( { ElementType.TYPE, ElementType.METHOD } ) public @interface Doc {
/** Description of class or method */
String desc() default "";
/** Description of parameters, if annotated element is a method & has parameters */
String[] params default {};
/** Description of return value, if annotated element is a method & isn't void */
String returnVal() default "";
}
This is my Main.java
package com.teamtreehouse.docgen;
import com.teamtreehouse.math.MathUtils; import java.lang.reflect.Method;
public class Main { public static void main(String[] args) { // TODO: Process the MathUtils class's annotations
Class<?> clazz = MathUtils.class;
Method[] methods = clazz.getMethods();
for ( Method m : methods )
{
System.out.printf( "names: %s%n", m.getName() );
}
}
}
This is the MathUtils.java
package com.teamtreehouse.math;
import java.awt.geom.Point2D; import com.teamtreehouse.docgen.Doc;
@Doc(
desc = "Utility class"
)
public class MathUtils {
private static final Double EPSILON = 0.0001;
@Doc(
desc = "Calculates the are of a triangle",
params = { "Coordinates of first vertex" },
returnVal = "Calculated are"
)
public static Double triangleArea(Point2D.Double a, Point2D.Double b, Point2D.Double c) {
return 0.0;
}
@Doc(
desc = "Calculates distance between given points",
params = { "Coordinates of one point", "Coordinates of another point" }
returnVal = ""
)
public static Double distance(Point2D.Double a, Point2D.Double b) {
return 0.0;
}
public static Double[] quadraticRoots(int a, int b, int c) {
return new Double[]{};
}
@Doc(
desc = "Displays the value of epsilon"
)
public static void epsilon() {
}
private static boolean arePointsClose(Point2D.Double a, Point2D.Double b) {
return false;
}
}
and these are the god damn errors..
src/com/teamtreehouse/math/MathUtils.java:24: error: ')' expected params = { "Coordinates of one point", "Coordinates of another point" } ^ src/com/teamtreehouse/math/MathUtils.java:25: error: <identifier> expected returnVal = "" ^ src/com/teamtreehouse/math/MathUtils.java:25: error: ';' expected returnVal = "" ^ src/com/teamtreehouse/docgen/Doc.java:18: error: illegal start of expression String[] params = default {}; ^ src/com/teamtreehouse/docgen/Doc.java:18: error: illegal start of type String[] params = default {}; ^ src/com/teamtreehouse/docgen/Doc.java:18: error: <identifier> expected String[] params = default {}; ^ src/com/teamtreehouse/docgen/Doc.java:18: error: = expected String[] params = default {}; ^ src/com/teamtreehouse/docgen/Doc.java:18: error: ';' expected String[] params = default {}; ^ src/com/teamtreehouse/docgen/Doc.java:21: error: <identifier> expected String returnVal() default ""; ^ src/com/teamtreehouse/math/MathUtils.java:25: error: cannot find symbol returnVal = "" ^ symbol: class returnVal location: class MathUtils src/com/teamtreehouse/docgen/Doc.java:21: error: cannot find symbol String returnVal() default ""; ^ symbol: class returnVal location: @interface Doc src/com/teamtreehouse/math/MathUtils.java:15: error: cannot find symbol params = { "Coordinates of first vertex" }, ^ symbol: method params() location: @interface Doc src/com/teamtreehouse/math/MathUtils.java:16: error: cannot find symbol returnVal = "Calculated are" ^ symbol: method returnVal() location: @interface Doc src/com/teamtreehouse/math/MathUtils.java:24: error: cannot find symbol params = { "Coordinates of one point", "Coordinates of another point" } ^ symbol: method params() location: @interface Doc src/com/teamtreehouse/math/MathUtils.java:22: error: annotation type not applicable to this kind of declaration @Doc( ^ 15 errors
If someone could help me i would be gratefull :(
2 Answers
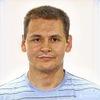
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsLooks like you forgot the comma after "params" argument of annotation:
@Doc(
desc = "Calculates distance between given points",
params = { "Coordinates of one point", "Coordinates of another point" }, // PUT comma here
returnVal = ""
)
That is why compiler says
error: ')' expected
Because it thinks that if there is no comma then parentheses should be closed.
Let me know if there other errors. And if possible format your code with markdown , take a look at cheatsheet:
https://teamtreehouse.com/community/cheatsheet
So that all your code is in that black window.
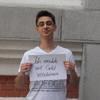
Placinta Alexandru
3,011 PointsI use Intellijidea, but yesterday i just wanted to use the workspace and all got messy and i got angry cause i was searching for my mistake for some time.
Placinta Alexandru
3,011 PointsPlacinta Alexandru
3,011 PointsThanks. That comma cost me 4 errors, but i still have another 11 that i do not know how to solve..
Placinta Alexandru
3,011 PointsPlacinta Alexandru
3,011 PointsLol. Sorry for wasting your time. I was too nervous yesterday and did not notice that my params in annotiation source folder was not declared as a function, i forgot () :))
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsAlexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsNo problem.
I suggest you use Intellijidea and check your code there.
Will save you a lot of time, since I saw you finished LDE course in Java :)