Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial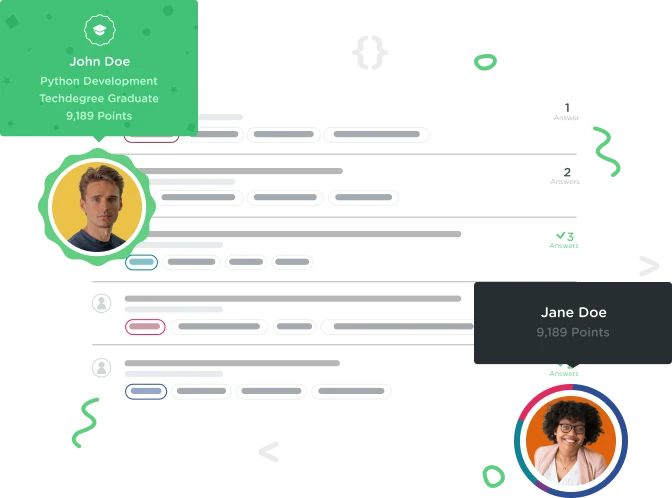
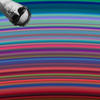
Gregorio Massara
3,544 PointsI have a constructor initializing all the private variables but I still get the Bummer error π€
Bummer error is not seeing my constructor. And also does not mention the title variable that is required in the instructions. if someone can double check my code would e great thanks!
package com.example;
import java.util.Date;
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public void blogPost() {
}
public void blogPost(String author, String title, String body, String category, Date creationDate) {
this.mAuthor = author;
this.mTitle = title;
this.mBody = body;
this.mCategory = category;
this.mCreationDate = creationDate;
}
public void blogPost(String author, String body, String category, Date creationDate) {
this.mAuthor = author;
this.mBody = body;
this.mCategory = category;
this.mCreationDate = creationDate;
}
public String getAuthor() {
return mAuthor;
}
public String getDate() {
return mCreationDate.toString();
}
public String getTitle() {
return mTitle;
}
public String getBody() {
return mBody;
}
public String getCategory() {
return mBody;
}
}
2 Answers

Lauren Moineau
9,483 PointsHi Gregorio. There are a few issues here:
- Constructors do not have a return type (so no void)
- The name of a constructor is the same as the name of the class (so it start with an upper-case letter)
- If you use the m convention (starting your field variable names with the prefix "m"), you do not need to use the "this" keyword in the constructor (or the setter, for that matter)
So putting it all together, your 2 options are:
Option #1
public class BlogPost {
private String mAuthor;
private String mTitle;
private String mBody;
private String mCategory;
private Date mCreationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
mAuthor = author;
mTitle = title;
mBody = body;
mCategory = category;
mCreationDate = creationDate;
}
}
Option #2
public class BlogPost {
private String author;
private String title;
private String body;
private String category;
private Date creationDate;
public BlogPost(String author, String title, String body, String category, Date creationDate) {
this.author = author;
this.title = title;
this.body = body;
this.category = category;
this.creationDate = creationDate;
}
}
Also, I'm not quite sure why you have so many constructors and getters as the exercise just seem to request one of each. But I guess you just wanted to practice :) Hope that helps. Happy coding!

Lauren Moineau
9,483 PointsYou're welcome Gregorio! :D It happens to all of us. We definitely have it easy with IntelliJ ^^
Gregorio Massara
3,544 PointsGregorio Massara
3,544 PointsThank You, Lauren ππΌπ it worked! Schoolboy error, so much harder without IntelliJ spotting these errors π