Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial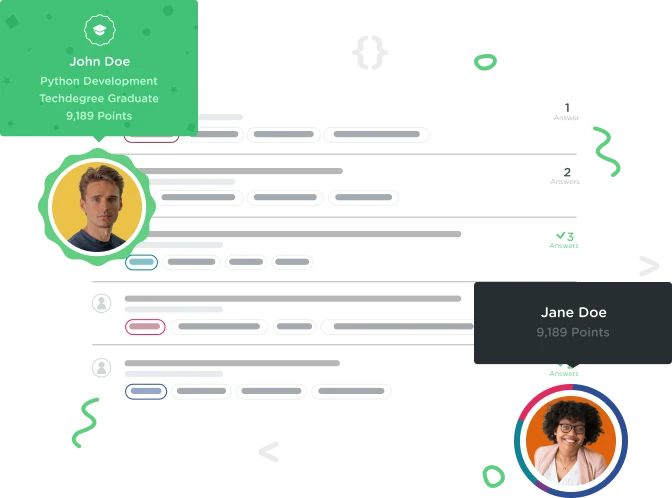
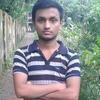
Niyamat Almass
8,176 Pointsi have a problem
i solve the challenge but there are some error in my example.java please solve the problem?
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getDescription() {
return mDescription;
}
public ForumPost(User author, String title, String description) {
}
}
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
public String getFirstName(){
return mFirstName;
}
public String getLastName(){
return mLastName;
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic) {
mTopic = topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
User author = new User("user", "scheu");
ForumPost post = new ForumPost(User, "Bomb", "boom!");
forum.addPost(post);
}
}
3 Answers

Ken Alger
Treehouse TeacherNiyamat;
- Replace the old
author
object with your name hard coded with the one that takesargs[0]
andargs[1]
. - Now that you have an
author
object put that in when you create yourForumPost
object. Currently you are usingniyamat
which is an undefined variable or object. Replace that withauthor
.
Ken

Ken Alger
Treehouse TeacherNiyamat;
The error you are having is in the User author = new User("user", "scheu");
line of code. The challenge is wanting to be able to utilize a name passed into it as described in the prompting code
System.out.println("first and last name are required. eg: java Example Craig Dennis");
When you create the new User object, author
you want to be able to pass into it the names given. So, in my case to run the program from the command line, I would type in java Example Ken Alger
and the Ken and Alger would get passed into the author
object for the firstName and lastName values. Make sense?
Post back if you are still stuck.
Ken
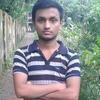
Niyamat Almass
8,176 PointsI have still error message .my example.java is
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
User author = new User("Niyamat", "Almass");
ForumPost post = new ForumPost(Niyamat, "Bomb", "boom!");
forum.addPost(post);
}
}
my error is
./Example.java:12: error: cannot find symbol
ForumPost post = new ForumPost(Niyamat, "Bomb", "boom!");
^
symbol: variable Niyamat
location: class Example
1 error
How to solve the error.What is the exactly code?

Ken Alger
Treehouse TeacherNiyamat;
That is a step in the right direction, but obviously not quite it, there are still a couple of issues.
Let's take another look at the User
object author
you have created. As I stated before, you want to be able to pass in a first and last name from the command line at run time. So if I type in the java Example Ken Alger
command, the User
object author
will take the arguments Ken
and Alger
. Similarly if you were to type in java Example Niyamat Almass
we need author
to take Niyamat
and Almass
. This should happen without having to alter the code.
How can we accomplish this? If you look at the main()
method for the Example
class you will notice that it takes a String [] args
as it's argument. What the heck does that mean? It means that it accepts an array (denoted by the square brackets) of arguments as a variable args
. This concept is touched on in the Hangman game in the course in which we do java Hangman someWordToSolve
and that game examines args[0]
. We need to do a similar thing here with this challenge.
Our User
object needs to be able to accept two arguments, args[0]
and args[1]
. This will "grab" the first two arguments after our java Example
statement in the command line. Since the constructor for the User
class accepts two arguments (first name and last name) we should be able to pass in the command line arguments into our model. We can do that during the creation of our User
object with
// set the firstName and lastName to the name passed in from the command line
User author = new User(args[0], args[1]);
The other issue you have in the above code is for the creation of your ForumPost
object. If you take a look at the constructor for that class, it should be set up as ForumPost(User author, String title, String description)
. Since we just discussed the creation of a User
object we should just pass in the author
object during the creation of our ForumPost
object.
Post back with additional questions or if you are still stuck.
Happy coding,
Ken
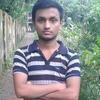
Niyamat Almass
8,176 Pointshey Ken Alger thank you.but i still don't get it.where i put the argument method (User author = new User(args[0], args[1]);)
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
User author = new User(args[0], args[1]);
User author = new User("Niyamat", "Almass");
ForumPost post = new ForumPost(niyamat, "Bomb", "boom!");
forum.addPost(post);
}
}
There is some error
./Example.java:11: error: variable author is already defined in method main(String[])
User author = new User("Niyamat", "Almass");
^
./Example.java:12: error: cannot find symbol
ForumPost post = new ForumPost(niyamat, "Bomb", "boom!");
^
symbol: variable niyamat
location: class Example
2 error
And please tell me the right code about this
User author = new User("Niyamat", "Almass");
ForumPost post = new ForumPost(niyamat, "Bomb", "boom!");
Niyamat Almass
8,176 PointsNiyamat Almass
8,176 PointsHey Ken Alger i got it!!!!!!!!!!!!!!!! .Thank you so much for your help.
Ken Alger
Treehouse TeacherKen Alger
Treehouse TeacherNiyamat;
Pleased that it worked out. This was a tough challenge with getting all of the various classes to interact with one another. Great job in sticking with it and getting it resolved.
Happy coding,
Ken