Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial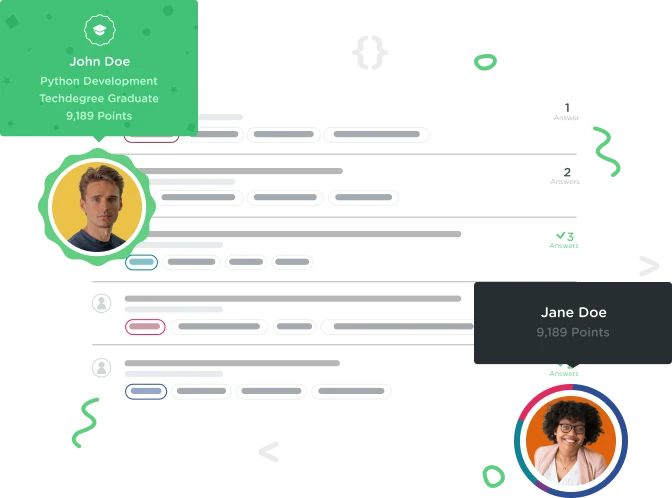

ohadyohay
867 PointsI have a problem in my code but I don't understand what is the problem
That is my solution for step number 4 of the practice named "Forum" from this course
public class Forum {
private String topic;
public Forum(String topic) {
this.topic = topic;
}
public String getTopic() {
return topic;
}
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
this.topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle() );
}
}
public class User {
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
public String getFirstName() {
return firstName;
}
public String getLastName() {
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
public ForumPost(User autor, String title, String description) {
this.author = author;
this.title = title;
this.description = description;
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
Forum forum = new Forum("Java");
User author = new User(args[0], args[1]);
ForumPost post = new ForumPost(author, "How to use arrays?", "Craig Dennis explains how to create and use arrays.");
forum.addPost(post);
}
}

ohadyohay
867 PointsThe error which I got is:
Beginning forum example
java.lang.NullPointerException
at Forum.addPost(Forum.java:17)
at Main.main(Main.java:14)
at JavaTester.run(JavaTester.java:185)
at JavaTester.main(JavaTester.java:45)
2 Answers

Leandro Botella Penalva
17,618 PointsHi ohadyohay,
Check your ForumPost constructor, it has a parameter called "autor" but inside the constructor you try to use it as "author".
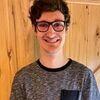
Trent Christofferson
18,846 PointsIt was very tricky, but I found it. In your forum post constructor, there is a typo in the parentheses. Currently it is User autor and it needs to be User author. It is null because you set this.author = author; but author does not exist because it is autor.
didnt see it was answered already, but hopefully you got it now :)
Trent Christofferson
18,846 PointsTrent Christofferson
18,846 Pointscan you post the error you are getting?