Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial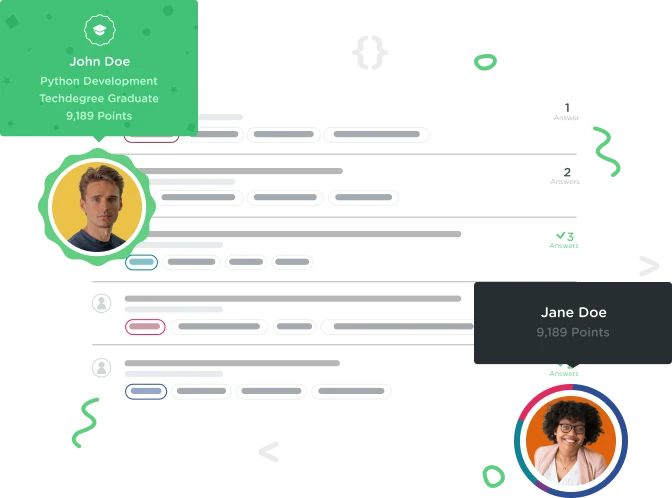
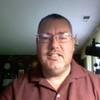
Michael Maitoza
3,805 PointsI have a question on the create a max() function exercise in JavaScript Basics. I am totally lost. Any help?
I'm not sure what I am doing wrong. Am I looking at this problem the wrong way? Any help would be appreciated.
function max(5, 10) {
if (5 > 10) {
return 5;
] else {
return 10;
}
}
2 Answers
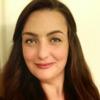
Jennifer Nordell
Treehouse TeacherHi there! Let's talk for a second about the purpose of a function. The idea behind having one at all is so that you can call that function, have it execute some code and then return a result or do some generic work that needs to be repeated. This will probably be based on things that you send in. You may want to run it once, but you may want it to run thousands of times and very likely the conditions will change over time.
Let me give you sort of a concrete example. Let's say that you hire an assistant and just about every workday you have the assistant go to the deli down the street and get you lunch. The very first time this is done, you give the assistant directions to the deli, explain how to charge it to the company, and give them your food and drink order. But the next time you ask the assistant to go to the deli, you don't want to have to explain the driving instructions nor the payment method again. That would be silly and you'd likely fire them if you had to do that every time. Instead, you'd simply call the goGetLunch()
function, and pass in your food and drink order for what you wanted that day. That very well may change from day to day.
In code, you might see something like:
// Monday's order
goGetLunch("ham sandwich", "large cafe latte");
// Tuesday's order
goGetLunch("chicken caesar salad", "large iced tea");
Then you would have the function the assistant is running return your food But the driving instructions and payment method never change so there's no reason to code those parts again.
Your code is hard-coding those values. It will always be the same result and the numbers will never change. This is equivalent to you never being able to change your lunch order with your assistant. What we're looking for is something really generic. We want the max value returned no matter which two numbers we send in. Take a look:
function max(a, b) { // when 2 numbers are sent here they will be assigned to a and b respectively
if(a > b) { // if a is greater than b
return a; // return a
} else { //otherwise
return b; // return b
}
}
alert(max(9, 30)); // We can call the max function and it will return the max number of whichever 2 numbers we send and print that in the alert.
Hope this helps clarify things!
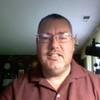
Michael Maitoza
3,805 PointsHere's the challenge: Create a new function named max which accepts two numbers as arguments (you can name the arguments, whatever you would like). The function should return the larger of the two numbers.
HINT: You'll need to use a conditional statement to test the 2 parameters to see which is the larger of the two.
Michael Maitoza
3,805 PointsMichael Maitoza
3,805 PointsThanks so much. The cleared things up so much for me. Thanks for your patience and well explained answer. I appreciate the help.