Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial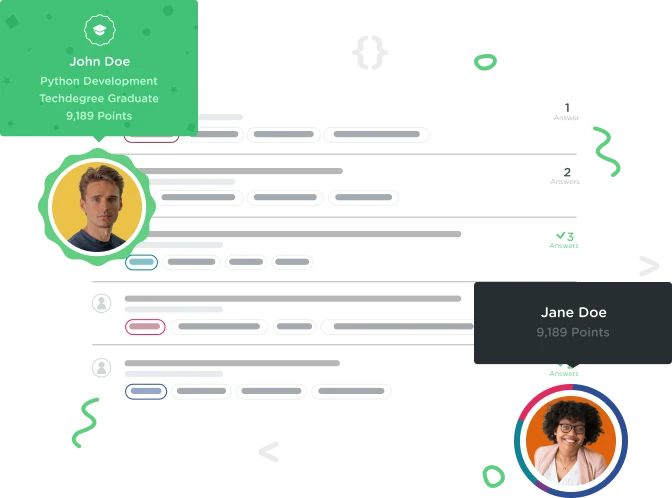
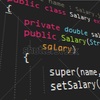
simon123123
1,291 PointsI have an error
package com.example.socialneworkshortcut;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
public class MainActivity extends Activity {
Button post, buttonimage, postimage;
EditText text;
ImageView image;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
post = (Button) findViewById(R.id.button1);
buttonimage = (Button) findViewById(R.id.button2);
postimage = (Button) findViewById(R.id.button3);
text = (EditText) findViewById(R.id.editText1);
image = (ImageView) findViewById(R.id.imageView1);
buttonimage.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent();
intent.setType("image/*");
intent.setAction(intent.ACTION_GET_CONTENT);
startActivityForResult(Intent.createChooser(intent,
"Where do you want your photo from?"), 1);
}
});
post.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
Intent shareIntent = new Intent();
shareIntent.setAction(Intent.ACTION_SEND);
shareIntent.setType("text/plain");
shareIntent.putExtra(Intent.EXTRA_TEXT, text.getText()
.toString());
startActivity(Intent.createChooser(shareIntent,
"Where do you want to share?"));
}
});
postimage.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
Intent shareIntent = new Intent();
shareIntent.setAction(Intent.ACTION_SEND);
shareIntent.setType("image/jpeg");
shareIntent.putExtra(Intent.EXTRA_STREAM, image);
startActivity(Intent.createChooser(shareIntent, "Where do you want to share?"));
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (resultCode == RESULT_OK) {
if (requestCode == 1) {
image.setImageURI(data.getData());
}
}
}
}
shareIntent.putExtra(Intent.EXTRA_STREAM, image);
This line gives me and error which says "The method putExtra(String, boolean) in the type Intent is not applicable for the arguments (String, ImageView)"
What am I doing wrong?
2 Answers

Ben Jakuben
Treehouse TeacherYour image
variable has the data type of ImageView
. The ImageView is just the container to display the image--it's a layout element. It's not the kind of thing you want to share via the intent. I'm assuming you want to share the image itself, right? Totally understandable mistake. :) If you change the name of your image
variable to imageView
instead, then the naming convention might help you keep them separate.
You're setting the ImageView with the following line:
image.setImageURI(data.getData());
To share an image via an intent like you're doing with EXTRA_STREAM
, you need to provide the URI to the image. So change that line to the following and I think you'll be set:
shareIntent.putExtra(Intent.EXTRA_STREAM, data.getData());
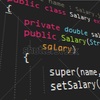
simon123123
1,291 Pointspostimage.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
Intent shareIntent = new Intent();
shareIntent.setAction(Intent.ACTION_SEND);
shareIntent.setType("image/jpeg");
shareIntent.putExtra(Intent.EXTRA_STREAM, data.getData());
startActivity(Intent.createChooser(shareIntent, "Where do you want to share?"));
}
});
Did you mean like this because if this still doesn't work