Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial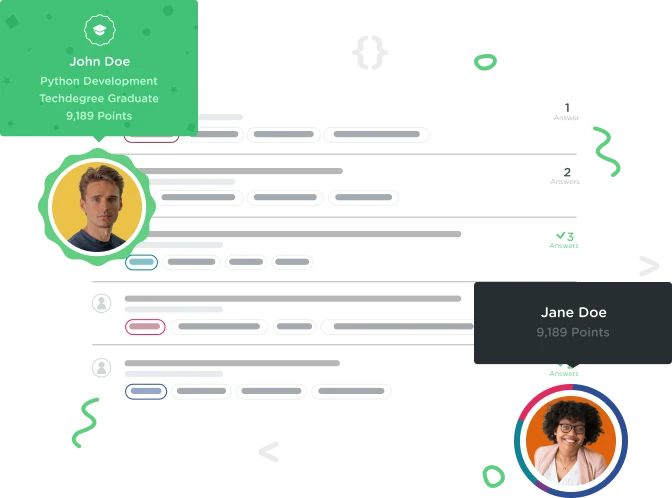

Daniel Horowitz
Courses Plus Student 7,305 PointsI have an odd issue, all of my links on the shirts4mike website are working, except 'shirts'.
When I click on 'shirts' I get a 403 forbidden error page pop up. It's only happening for this page. Why would that be? I downloaded the project files and have not edited anything other than what was covered on the video. I have all permissions on the folder and subfolders 777. I was thinking maybe it had something to do with the .htaccess rewrite rules, but again, I haven't changed anything and then why would it only be affecting the 'shirts' page. Does anyone have any ideas? I'll post my code, just in case anyone wants to see it.
This is: shirts.php
cd <?php
require_once("../inc/config.php");
require_once(ROOT_PATH . "inc/products.php");
// retrieve current page number from query string; set to 1 if blank
if (empty($_GET["pg"])) {
$current_page = 1;
} else {
$current_page = $_GET["pg"];
}
// set strings like "frog" to 0; remove decimals
$current_page = intval($current_page);
$total_products = get_products_count();
$products_per_page = 8;
$total_pages = ceil($total_products / $products_per_page);
// redirect too-large page numbers to the last page
if ($current_page > $total_pages) {
header("Location: ./?pg=" . $total_pages);
}
// redirect too-small page numbers (or strings converted to 0) to the first page
if ($current_page < 1) {
header("Location: ./");
}
// determine the start and end shirt for the current page; for example, on
// page 3 with 8 shirts per page, $start and $end would be 17 and 24
$start = (($current_page - 1) * $products_per_page) + 1;
$end = $current_page * $products_per_page;
if ($end > $total_products) {
$end = $total_products;
}
$products = get_products_subset($start,$end);
?><?php
$pageTitle = "Mike's Full Catalog of Shirts";
$section = "shirts";
include(ROOT_PATH . 'inc/header.php'); ?>
<div class="section shirts page">
<div class="wrapper">
<h1>Mike’s Full Catalog of Shirts</h1>
<?php include(ROOT_PATH . "inc/partial-list-navigation.html.php"); ?>
<ul class="products">
<?php
foreach($products as $product) {
include(ROOT_PATH . "inc/partial-product-list-view.html.php");
}
?>
</ul>
<?php include(ROOT_PATH . "inc/partial-list-navigation.html.php"); ?>
</div>
</div>
<?php include(ROOT_PATH . 'inc/footer.php') ?>
This is: .htaccess
RewriteEngine On
RewriteRule ^shirts/$ /shirts/shirts.php
RewriteRule ^shirts/([0-9]+)/$ /shirts/shirt.php?id=$1
RewriteRule ^receipt.php$ /receipt/ [R=301]
RewriteRule ^contact.php$ /contact/ [R=301]
RewriteRule ^shirts.php$ /shirts/ [R=301]
RewriteRule ^(shirts/[0-9]+)$ /$1/ [R=301]
RewriteCond %{QUERY_STRING} ^id=([0-9]+)$
RewriteRule ^shirt.php$ /shirts/%1/? [R=301]
This is: products.php
<?php
/*
* Returns the four most recent products, using the order of the elements in the array
* @return array a list of the last four products in the array;
the most recent product is the last one in the array
*/
function get_products_recent() {
$recent = array();
$all = get_products_all();
$total_products = count($all);
$position = 0;
foreach($all as $product) {
$position = $position + 1;
if ($total_products - $position < 4) {
$recent[] = $product;
}
}
return $recent;
}
/*
* Loops through all the products, looking for a search term in the product names
* @param string $s the search term
* @return array a list of the products that contain the search term in their name
*/
function get_products_search($s) {
$results = array();
$all = get_products_all();
foreach($all as $product) {
if (stripos($product["name"],$s) !== false) {
$results[] = $product;
}
}
return $results;
}
/*
* Counts the total number of products
* @return int the total number of products
*/
function get_products_count() {
return count(get_products_all());
}
/*
* Returns a specified subset of products, based on the values received,
* using the order of the elements in the array .
* @param int the position of the first product in the requested subset
* @param int the position of the last product in the requested subset
* @return array the list of products that correspond to the start and end positions
*/
function get_products_subset($positionStart, $positionEnd) {
$subset = array();
$all = get_products_all();
$position = 0;
foreach($all as $product) {
$position += 1;
if ($position >= $positionStart && $position <= $positionEnd) {
$subset[] = $product;
}
}
return $subset;
}
/*
* Returns the full list of products. This function contains the full list of products,
* and the other model functions first call this function.
* @return array the full list of products
*/
function get_products_all() {
// $products = array();
// $products[101] = array(
// "name" => "Logo Shirt, Red",
// "img" => "img/shirts/shirt-101.jpg",
// "price" => 18,
// "paypal" => "9P7DLECFD4LKE",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[102] = array(
// "name" => "Mike the Frog Shirt, Black",
// "img" => "img/shirts/shirt-102.jpg",
// "price" => 20,
// "paypal" => "SXKPTHN2EES3J",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[103] = array(
// "name" => "Mike the Frog Shirt, Blue",
// "img" => "img/shirts/shirt-103.jpg",
// "price" => 20,
// "paypal" => "7T8LK5WXT5Q9J",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[104] = array(
// "name" => "Logo Shirt, Green",
// "img" => "img/shirts/shirt-104.jpg",
// "price" => 18,
// "paypal" => "YKVL5F87E8PCS",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[105] = array(
// "name" => "Mike the Frog Shirt, Yellow",
// "img" => "img/shirts/shirt-105.jpg",
// "price" => 25,
// "paypal" => "4CLP2SCVYM288",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[106] = array(
// "name" => "Logo Shirt, Gray",
// "img" => "img/shirts/shirt-106.jpg",
// "price" => 20,
// "paypal" => "TNAZ2RGYYJ396",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[107] = array(
// "name" => "Logo Shirt, Teal",
// "img" => "img/shirts/shirt-107.jpg",
// "price" => 20,
// "paypal" => "S5FMPJN6Y2C32",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[108] = array(
// "name" => "Mike the Frog Shirt, Orange",
// "img" => "img/shirts/shirt-108.jpg",
// "price" => 25,
// "paypal" => "JMFK7P7VEHS44",
// "sizes" => array("Large","X-Large")
// );
// $products[109] = array(
// "name" => "Get Coding Shirt, Gray",
// "img" => "img/shirts/shirt-109.jpg",
// "price" => 20,
// "paypal" => "B5DAJHWHDA4RC",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[110] = array(
// "name" => "HTML5 Shirt, Orange",
// "img" => "img/shirts/shirt-110.jpg",
// "price" => 22,
// "paypal" => "6T2LVA8EDZR8L",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[111] = array(
// "name" => "CSS3 Shirt, Gray",
// "img" => "img/shirts/shirt-111.jpg",
// "price" => 22,
// "paypal" => "MA2WQGE2KCWDS",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[112] = array(
// "name" => "HTML5 Shirt, Blue",
// "img" => "img/shirts/shirt-112.jpg",
// "price" => 22,
// "paypal" => "FWR955VF5PALA",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[113] = array(
// "name" => "CSS3 Shirt, Black",
// "img" => "img/shirts/shirt-113.jpg",
// "price" => 22,
// "paypal" => "4ELH2M2FW7272",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[114] = array(
// "name" => "PHP Shirt, Yellow",
// "img" => "img/shirts/shirt-114.jpg",
// "price" => 24,
// "paypal" => "AT3XQ3ZVP2DZG",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[115] = array(
// "name" => "PHP Shirt, Purple",
// "img" => "img/shirts/shirt-115.jpg",
// "price" => 24,
// "paypal" => "LYESEKV9JWE3A",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[116] = array(
// "name" => "PHP Shirt, Green",
// "img" => "img/shirts/shirt-116.jpg",
// "price" => 24,
// "paypal" => "KT7MRRJUXZR34",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[117] = array(
// "name" => "Get Coding Shirt, Red",
// "img" => "img/shirts/shirt-117.jpg",
// "price" => 20,
// "paypal" => "5UXJG8PXRXFKE",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[118] = array(
// "name" => "Mike the Frog Shirt, Purple",
// "img" => "img/shirts/shirt-118.jpg",
// "price" => 25,
// "paypal" => "KHP8PYPDZZFTA",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[119] = array(
// "name" => "CSS3 Shirt, Purple",
// "img" => "img/shirts/shirt-119.jpg",
// "price" => 22,
// "paypal" => "BFJRFE24L93NW",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[120] = array(
// "name" => "HTML5 Shirt, Red",
// "img" => "img/shirts/shirt-120.jpg",
// "price" => 22,
// "paypal" => "RUVJSBR9FXXWQ",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[121] = array(
// "name" => "Get Coding Shirt, Blue",
// "img" => "img/shirts/shirt-121.jpg",
// "price" => 20,
// "paypal" => "PGN6ULGFZTXL4",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[122] = array(
// "name" => "PHP Shirt, Gray",
// "img" => "img/shirts/shirt-122.jpg",
// "price" => 24,
// "paypal" => "PYR4QH97W2TSJ",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[123] = array(
// "name" => "Mike the Frog Shirt, Green",
// "img" => "img/shirts/shirt-123.jpg",
// "price" => 25,
// "paypal" => "STDAUJJTSPT54",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[124] = array(
// "name" => "Logo Shirt, Yellow",
// "img" => "img/shirts/shirt-124.jpg",
// "price" => 20,
// "paypal" => "2R2U74KWU5RXG",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[125] = array(
// "name" => "CSS3 Shirt, Blue",
// "img" => "img/shirts/shirt-125.jpg",
// "price" => 22,
// "paypal" => "GJG7F8EW3XFAS",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[126] = array(
// "name" => "Doctype Shirt, Green",
// "img" => "img/shirts/shirt-126.jpg",
// "price" => 25,
// "paypal" => "QW2LFRYGU7L4Q",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[127] = array(
// "name" => "Logo Shirt, Purple",
// "img" => "img/shirts/shirt-127.jpg",
// "price" => 20,
// "paypal" => "GFV6QVRMJU7F8",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[128] = array(
// "name" => "Doctype Shirt, Purple",
// "img" => "img/shirts/shirt-128.jpg",
// "price" => 25,
// "paypal" => "BARQMHMB565PN",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[129] = array(
// "name" => "Get Coding Shirt, Green",
// "img" => "img/shirts/shirt-129.jpg",
// "price" => 20,
// "paypal" => "DH9GXABU3P8GS",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[130] = array(
// "name" => "HTML5 Shirt, Teal",
// "img" => "img/shirts/shirt-130.jpg",
// "price" => 22,
// "paypal" => "4LZ3EUVCBENE4",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[131] = array(
// "name" => "Logo Shirt, Orange",
// "img" => "img/shirts/shirt-131.jpg",
// "price" => 20,
// "paypal" => "7BNDYJBKWD364",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// $products[132] = array(
// "name" => "Mike the Frog Shirt, Red",
// "img" => "img/shirts/shirt-132.jpg",
// "price" => 25,
// "paypal" => "Y6EQRE445MYYW",
// "sizes" => array("Small","Medium","Large","X-Large")
// );
// when creating a new product, create it first in PayPal and
// then copy the product ID from PayPal to use here
// automated duplication to copy the product_id/sku from the array key
// and duplicate it into the product details inside the array
// foreach ($products as $product_id => $product) {
// $products[$product_id]["sku"] = $product_id;
// }
try {
$db = new PDO("mysql:host=localhost;dbname=shirts4mike;port=3306", "root", "hkh92304");
$db->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
$db->exec("SET NAMES 'utf8'");
} catch (Exception $e) {
echo "Could not connect to the database.";
exit;
}
try {
$results = $db->query("SELECT name, price, img, sku, paypal FROM products ORDER BY sku ASC");
} catch (Exception $e) {
echo "Data could not be retrieved from the database.";
exit;
}
$products = $results->fetchAll(PDO::FETCH_ASSOC);
//foreach ($products as $product_id => $product) { $products[$product_id]["sku"] = $product_id; }
return $products;
}
?>
This is: nginx.error.log for this local dev site (/var/log/nginx/registration.app-error.log error)
2015/06/11 13:27:30 [error] 13703#0: *2 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/"
2015/06/11 13:27:33 [error] 13703#0: *2 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/"
2015/06/11 13:27:40 [error] 13703#0: *2 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/"
2015/06/11 13:30:11 [error] 13797#0: *1 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/"
2015/06/11 13:30:12 [error] 13797#0: *1 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/"
2015/06/11 13:30:50 [error] 13797#0: *1 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/"
2015/06/11 13:30:52 [error] 13797#0: *1 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/"
2015/06/11 13:31:17 [error] 13797#0: *1 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/search/"
2015/06/11 13:33:01 [error] 13864#0: *1 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/"
2015/06/11 13:35:23 [error] 13864#0: *3 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/"
2015/06/11 13:35:26 [error] 13864#0: *3 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/"
2015/06/11 13:35:29 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/132/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/132/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/"
2015/06/11 13:35:34 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/30/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/30/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/132/"
2015/06/11 13:35:35 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/30/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/30/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/30/"
2015/06/11 13:35:37 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/30/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/30/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/30/"
2015/06/11 13:35:37 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/30/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/30/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/30/"
2015/06/11 13:35:37 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/30/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/30/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/30/"
2015/06/11 13:35:38 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/29/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/29/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/30/"
2015/06/11 13:35:38 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/29/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/29/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/29/"
2015/06/11 13:35:46 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/29/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/29/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/29/"
2015/06/11 13:35:47 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/132/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/132/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/29/"
2015/06/11 13:35:49 [error] 13864#0: *3 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/132/"
2015/06/11 13:35:55 [error] 13864#0: *3 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/search/"
2015/06/11 13:36:01 [error] 13864#0: *3 FastCGI sent in stderr: "Unable to open primary script: /var/www/shirts4mike/shirts.php (No such file or directory)" while reading response header from upstream, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts.php HTTP/1.1", upstream: "fastcgi://unix:/var/run/php5-fpm.sock:", host: "shirts4mike.com", referrer: "http://shirts4mike.com/"
2015/06/11 13:36:05 [error] 13864#0: *3 FastCGI sent in stderr: "Unable to open primary script: /var/www/shirts4mike/shirts.php (No such file or directory)" while reading response header from upstream, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts.php HTTP/1.1", upstream: "fastcgi://unix:/var/run/php5-fpm.sock:", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts.php"
2015/06/11 13:36:08 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/129/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/129/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts.php"
2015/06/11 13:36:10 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/130/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/130/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/129/"
2015/06/11 13:36:11 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/130/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/130/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/130/"
2015/06/11 13:36:12 [error] 13864#0: *3 "/var/www/shirts4mike/shirts/130/index.html" is not found (2: No such file or directory), client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/130/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/130/"
2015/06/11 13:36:25 [error] 13864#0: *3 FastCGI sent in stderr: "Unable to open primary script: /var/www/shirts4mike/shirts.php (No such file or directory)" while reading response header from upstream, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts.php HTTP/1.1", upstream: "fastcgi://unix:/var/run/php5-fpm.sock:", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/130/"
2015/06/11 13:39:07 [error] 13864#0: *33 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts.php"
2015/06/11 13:39:10 [error] 13864#0: *33 FastCGI sent in stderr: "Unable to open primary script: /var/www/shirts4mike/shirts.php (No such file or directory)" while reading response header from upstream, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts.php HTTP/1.1", upstream: "fastcgi://unix:/var/run/php5-fpm.sock:", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts/130/"
2015/06/11 13:39:12 [error] 13864#0: *33 directory index of "/var/www/shirts4mike/shirts/" is forbidden, client: 127.0.0.1, server: shirts4mike.com, request: "GET /shirts/ HTTP/1.1", host: "shirts4mike.com", referrer: "http://shirts4mike.com/shirts.php"
2 Answers
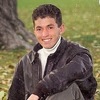
Tyrell Jentink
8,415 PointsFirst thing first... The "cd": Any text outside the
<?php ?>
will be passed to the browser as raw HTML; Without HTML tags, it will just be passed as text. SO, the "cd" should just appear at the top of the browser window, before all the rest of the code is run.
It is also PROBABLY a typo? It shouldn't be needed.
Now onto the forbidden error...
On the server, is shirts.php in the shirts/ folder? If not, the .htaccess rule is pointing to the wrong place.
You said the folders and subfolders had 777 permissions, but what about shirts.php itself? Is it 777 as well?
Finally, I've seen similar errors when SELinux is enabled on the server; If you are on a hosting service, this is unlikely; but if you are hosting it yourself, it may be worth looking into (On development machines, I just disable SELinux altogether).
I'm still not super confident in .htaccess... But I suspect the problem is in there somewhere. If the above doesn't help, we could try exploring a bit more in there.

Daniel Horowitz
Courses Plus Student 7,305 PointsI'm going to look into how to disable SELinux right now. Thank you. Yes, it is a local development server on my machine. No, the shirts.php is 777 as well, same user and group as the rest of the files and directories, and yes it is in the shirts/ directory. Thank you for your help, I'll let you know how disabling SELinux works.

Daniel Horowitz
Courses Plus Student 7,305 PointsSELinux and getenforce are both disabled and have been, so that can't be the problem.

Daniel Horowitz
Courses Plus Student 7,305 PointsAlso, if I click on any of the actual shirts on the home page, to view details, I get the hyper-link for the shirts page (i.e. http://shirts4mike.com/shirts/132/) but nothing happens. It does not change the page, it does nothing but show the link in the address bar.
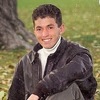
Tyrell Jentink
8,415 PointsFile permissions can be a sneaky beast; That wasn't the problem this time, but it's good to know those troubleshooting steps none the less. In the real world, you can probably defer to the System Administrator for such issues, but in our own development machines, it's good to know your way around.
As for the probable REAL problem, see my other answer ;)
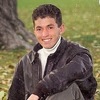
Tyrell Jentink
8,415 PointsI think I found it... In your .htaccess file, on the second rewrite rule and the last, you used "shirt.php" instead of "shirts.php."
Daniel Horowitz
Courses Plus Student 7,305 PointsDaniel Horowitz
Courses Plus Student 7,305 PointsAlso, what is the 'cd' at the beginning of the 'shirts.php' page?