Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial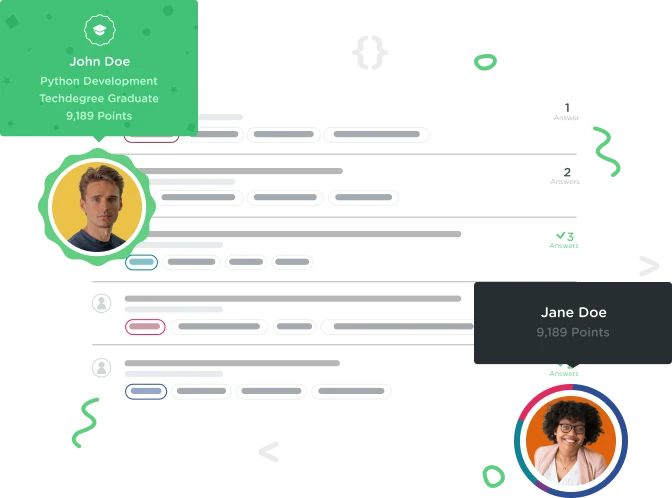

Russell Brookman
3,118 PointsI have been stuck on the For Loop for a really long time. I just can't make this thing count char's.
I have been stuck on this for a really long time. I can't make a for loop count char's. If you can show me how to do this I would really appreciate it. You can take a look at my code but by this point it is so screwed up I don't know where to go from here.
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public static void getTileCount(String mHand){
for (int numberOfTiles = 0; numberOfTiles < mHand.length; i++) {
if (mHand[numberOfTiles] > 0)
System.out.println("Number of " + (char) numberOfTiles + ": " + mHand[numberOfTiles]);
}
}}
5 Answers

Russell Brookman
3,118 PointsAlright, I'm going to ask someone else. Thanks anyway man.
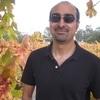
Kourosh Raeen
23,733 PointsHi Russell - A few points to coinsider:
The getTileCount() method shouldn't be static. It also shouldn't be void since it needs to return the tile count, which is an integer.
The parameter passed into the method should be a tile, which is a char.
Try using a fore each loop as the challenge suggests and loop through all the characters in mHand by turning it into an array of chars using the toCharArray() method:
for (char c : mHand.toCharArray()) {
}
Then inside the loop use an if statement to compare the current character c
with the tile passed into the method. If they are the same then increment by one a count variable that you've declared and initialized outside the loop. Once you're done with the loop return the count variable.
- There shouldn't be any printing to the screen. Just return the count.

Russell Brookman
3,118 PointsThanks. I think I got it.
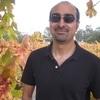
Kourosh Raeen
23,733 PointsThat's great! Good work!

Blake Larson
13,014 PointsHey Kourosh, appreciate the help but could you explain where the currentTile variable came from and how it worked in the for loop. Thanks.
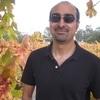
Kourosh Raeen
23,733 PointsHi Blake - In the for loop we are looping through an array of characters, mHand.toCharArray
, so the loop variable needs to be of type char and I called it currentTile because it is holding the tile in the current iteration of the loop, but you could call it anything. We are basically comparing the character passed into the method, called tile
, with all the characters in the mHand
string to count how many times that character appears in mHand
.
Hope this helps.

Russell Brookman
3,118 PointsI didn't get it. It won't accept my code. It looks right to me. Please tell me what I'm doing wrong?
public String getTileCount() { String numberOfTiles = ""; for (char tile : mHand.toCharArray()) { char tilesInHand = tile; if (mHand.indexOf(tile) >= 0) { tilesInHand = tile; } numberOfTiles += tilesInHand; } return numberOfTiles; }
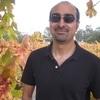
Kourosh Raeen
23,733 PointsHi Russell - These are changes you need to make to your code:
The getTileCount() method's job is to count the number of times a given tile appears in the string mHand
, so you need to pass that tile to the method. Also, since the count would be an integer the method should return an int:
public int getTileCount(char tile) {
}
You need an int variable to keep track of the number of times tile
appears in mHand
. Declare and initialize this variable to zero before the loop:
public int getTileCount(char tile) {
int count = 0;
for (char currentTile : mHand.toCharArray()) {
}
}
Inside the loop use an if statement to see if currentTile
is equal to tile
. If it is add one to count
:
public int getTileCount(char tile) {
int count = 0;
for (char currentTile : mHand.toCharArray()) {
if (c == tile) {
count++;
}
}
}
Finally, return count
after the for loop is done:
public int getTileCount(char tile) {
int count = 0;
for (char currentTile : mHand.toCharArray()) {
if (currentTile == tile) {
count++;
}
}
return count;
}

Russell Brookman
3,118 PointsI just wanted you to know that it worked. Thanks for your help.
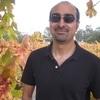
Kourosh Raeen
23,733 PointsMy pleasure! Happy coding!

Russell Brookman
3,118 PointsYou're the man buddy.