Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial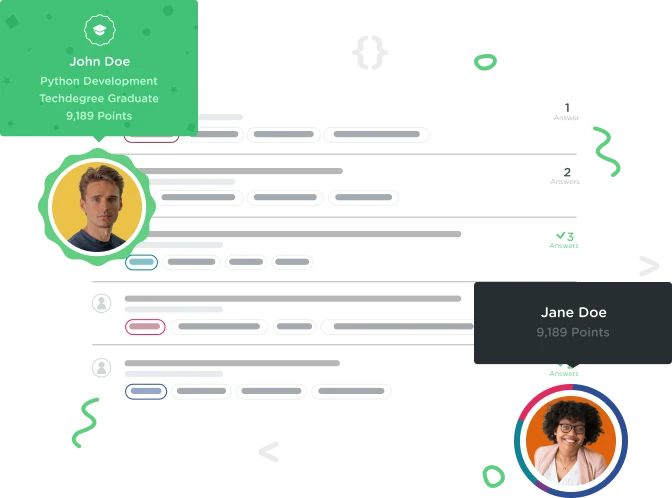

yoobin hong
6,645 PointsI have completed the extra challenge but I'm wondering why this else statement is acting the way it does
So my question is why is the program saying that the student is not in our directory even when the user types in the name of the student who is actually in the directory? I solved the problem looking through the previous asked questions and found the answer to the extra problem but I'm just curious as to why this is the case.
for(var i = 0; i < students.length; i++){ pupil = students[i] if (pupil.name.toUpperCase() === response ) { message += getReport(pupil); found = true; print(message); }else { alert("The student is not in our directory"); break; } }
This is my full script.
var students = [ {name: "Yoobin", track: "Javascript Full stack", achievements: 20, points: 5000 },
{name: "Sarah", track: "Front-end web", achievements: 10, points: 4000 },
{name: "David", track: "PHP", achievements: 50, points: 3000 },
{name: "Chris", track: "Wordpress", achievements: 60, points: 2000 },
{name: "Chris", track: "Ruby on rails", achievements: 100, points: 8000 } ]
var message = ''; var response; var pupil;
function print (message){ var div = document.getElementById('output') div.innerHTML = message; }
function getReport(pupil){ var report = "<h2> Student : " + pupil.name + "</h2>"; report += "<ul><li> Track : " + pupil.track + "</li>"; report += "<li>Achievements : " + pupil.achievements + "</li>"; report += "<li>Points : " + pupil.points + "</li></ul>"; return report; }
while(true){ response = prompt("Type in the name of the student you would like to view their stats on. Type QUIT if you would like to exit the prompt") response = response.toUpperCase();
if(response === null || response==="QUIT"){ break; } for(var i = 0; i < students.length; i++){ pupil = students[i] if (pupil.name.toUpperCase() === response ) { message += getReport(pupil); print(message); }else { alert("The student is not in our directory, Please search again"); break; } } }
2 Answers

andren
28,558 PointsThe core of the issue is that it is inside of a loop, the if
statement is evaluated for each student that is looped through.
I think it's easiest illustrated by an explanation of what happens behind the scenes when you run the program.
Let's say you type in the name "David", the for loop
will pull out the first student from the students array (Yoobin) and then check if that name matches the name you entered, since it does not the else
statement runs, since the else
statement contains a break
keyword the loop then ends right there.
That's the key thing to understand here, all of the students names gets run through that if
statement, which means that all of the students whose names do not match the one entered will cause the else
clause to trigger if it is placed within the loop. If you removed the break
keyword then you would see the alert pop up multiple times as the loop goes through the various student names.
A more robust way to implement a message like that would be to create a Boolean to track whether a student had been found, and then check that Boolean after the loop finished. Like this:
while (true) {
var wasFound = false; // Bool to track if student(s) was found
response = prompt("Type in the name of the student you would like to view their stats on. Type QUIT if you would like to exit the prompt")
response = response.toUpperCase();
if (response === null || response === "QUIT") {
break;
}
for (var i = 0; i < students.length; i++) {
pupil = students[i]
if (pupil.name.toUpperCase() === response) {
wasFound = true; // Set to true if found
message += getReport(pupil);
print(message);
}
}
if (!wasFound) { // Check if wasFound is false
alert("The student is not in our directory, Please search again");
}
}
That is just a quick solution of the top of my head, there are likely other more concise solutions as well.

yoobin hong
6,645 PointsOh!!!! Thank you for the explanation now I get why this code didn't work!! Thank you so much!!!!

Nathan Angulo
4,565 PointsThank you so much! I think I've finally grasped what's happening in the for loop now in this code. I appreciate it.
Nathan Angulo
4,565 PointsNathan Angulo
4,565 PointsI'm having trouble understanding the concept of for in this code. Could you elaborate what the [i] means after "students"?
andren
28,558 Pointsandren
28,558 PointsNathan Angulo: That is an example of a for loop.
A
for loop
takes three expressions within the parenthesis, the first is called the "initializing expression" and whatever you type here is executed once before the loop starts, the second is the "condition" which determines what causes the loop to run in the first place. And the third is called "increment Expression" and this is executed every time the loop has finished a run.In the code above
var i = 0
is the "initializing expression" so when the loop starts a variable calledi
is created and set to0
.i < students.length
is the "condition" so the loop will run as long asi
is less than the length of thestudents
array. Andi++
is the "increment Expression" so each time the the loop has ran it will incrementi
by one.When you place a number in a bracket after an array you tell JavaScript to pull out an item from the array at that index. So
students[0]
would pull out the item at index0
(which is the first item),students[1]
would pull out the item at index1
(which is the second item) and so on.So
students[i]
will pull out an item from the array. The first time the loop runsi
will be0
so the first student in the students array will be pulled out and assigned topupil
, the second time the loop runsi
will be1
(Sincei++
is used as the "increment Expression") so the second student will be pulled, the third timei
will be2
so the third student will be pulled out and so on.By the time the loop is finished (which happens when
i < students.length
is no longertrue
) all of the students will have been pulled out and run through the code within the loop.