Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial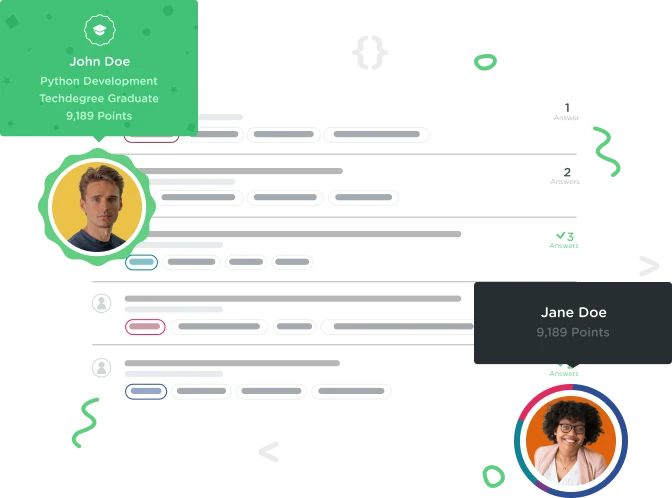

Jared Salwei
11,974 PointsI have created a new Class and I am calling that class to my main class to write the information to the display.
I have created a new Class and I am calling that class to my main class to write the information to the display. my question is I call the class three times one for each button. I also have to loop it three times in the new class. Can I shorten the code at all?
public MainPage()
{
this.InitializeComponent();
txtBoxRas.Text = "Rasmussen College";
txtBoxRas.FontSize = 14;
txtBoxRas.Foreground = new SolidColorBrush(Windows.UI.Colors.Red);
}
private void btnSubmit_Click(object sender, RoutedEventArgs e)
{
ProcessCalc();
}
private void ProcessCalc()
{
Int32 Var1 = Convert.ToInt32(txtBoxInput1.Text) + Convert.ToInt32(txtBoxInput2.Text);
txtBoxDisplay.Text = Convert.ToString(Var1);
}
async private void btnCourse1_Click(object sender, RoutedEventArgs e)
{
BL_PageContent.Cource1();
txtBoxCourse.Text = BL_PageContent.VarOutput;
var dialog = new MessageDialog(BL_PageContent.VarOutput);
await dialog.ShowAsync();
}
async private void btnCourse2_Click(object sender, RoutedEventArgs e)
{
BL_PageContent.Cource2();
txtBoxCourse.Text = BL_PageContent.VarOutput;
var dialog = new MessageDialog(BL_PageContent.VarOutput);
await dialog.ShowAsync();
}
async private void btnCourse3_Click(object sender, RoutedEventArgs e)
{
BL_PageContent.Cource3();
txtBoxCourse.Text = BL_PageContent.VarOutput;
var dialog = new MessageDialog(BL_PageContent.VarOutput);
await dialog.ShowAsync();
}
}
}
++++++++++++++++++++++ The other class++++++++++++++++++++
namespace App1
{
class BL_PageContent
{
public static string VarOutput { get; set; }
public static void Cource1()
{
VarOutput = "";
string[] names = new string[3] { "COP3488C", "UWP1", "This course is mobile app development." };
for (int i = 0; i < names.Length; i++)
{
VarOutput = VarOutput + names[i] + " ";
}
}
public static void Cource2()
{
VarOutput = "";
string[] names = new string[3] { "DOP3488B,", "UWC1,", "This course is Cloud Computing." };
for (int i = 0; i < names.Length; i++)
{
VarOutput = VarOutput + names[i] + " ";
}
}
public static void Cource3()
{
VarOutput = "";
string[] names = new string[3] { "BOP3589,", "UWP2,", "This course Computer Programming Java 1." };
for (int i = 0; i < names.Length; i++)
{
VarOutput = VarOutput + names[i] + " ";
}
}
}
}
Edited: Made the question more readable - Dane E. Parchment Jr. (Moderator)

Jared Salwei
11,974 PointsThis is not part of the course.
1 Answer

Olga Grijalba
6,086 PointsI see two parts that can be improved here:
-
The three buttons could have the same event, lets say the 3 of them call to "btnCourse_Click". Also, you would need to put a name/id to each one of the buttons so you will know through the sender which one of them invoked the method.
async private void btnCourse_Click(object sender, RoutedEventArgs e) { Button clickedButton = sender as Button; if (clickedButton.Name() == "Button1") BL_PageContent.Cource1(); else if (clickedButton.Name() == "Button2") BL_PageContent.Cource2(); else if (clickedButton.Name() == "Button3") BL_PageContent.Cource3(); txtBoxCourse.Text = BL_PageContent.VarOutput; var dialog = new MessageDialog(BL_PageContent.VarOutput); await dialog.ShowAsync(); }
-
On the other class, the 3 methods look almost the same. You could keep only one and send an array (that is what changes between them). This array should be send from the "btnCourseClick", so you can know which array iterate, so let's change that on the event. And I would declare the arrays as universal variables at the beggining. The final changes:
// global variables string[] names1 = new string[3] { "COP3488C", "UWP1", "This course is mobile app development." }; string[] names2 = new string[3] { "DOP3488B,", "UWC1,", "This course is Cloud Computing." }; string[] names3 = new string[3] { "BOP3589,", "UWP2,", "This course Computer Programming Java 1." }; async private void btnCourse_Click(object sender, RoutedEventArgs e) { Button clickedButton = sender as Button; if (clickedButton.Name() == "Button1") BL_PageContent.Cource1(names1); // Pass the corresponding array according to clicked button else if (clickedButton.Name() == "Button2") BL_PageContent.Cource2(names2); else if (clickedButton.Name() == "Button3") BL_PageContent.Cource3(names3); txtBoxCourse.Text = BL_PageContent.VarOutput; var dialog = new MessageDialog(BL_PageContent.VarOutput); await dialog.ShowAsync(); } // The other class, receiving the array to iterate public static void Cource1(string[] names) { VarOutput = ""; for (int i = 0; i < names.Length; i++) VarOutput = VarOutput + names[i] + " "; }
Steven Parker
231,275 PointsSteven Parker
231,275 PointsIs this part of a course? Could you provide a link to the course page you were working with?