Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial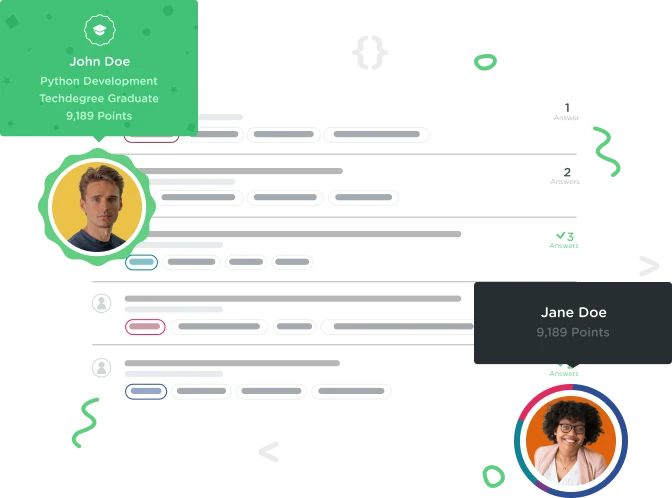

Hyeon Kwang Choi
5,522 PointsI have different structure of code for this challenge. I would like to hear any advice if my code has problems.
var students = [
{
name: 'Dave',
track: 'Front End Development',
achievements: 158,
points: 14730
},
{
name: 'Jody',
track: 'iOS Development with Swift',
achievements: '175',
points: '16375'
},
{
name: 'Jordan',
track: 'PHP Development',
achievements: '55',
points: '2025'
},
{
name: 'John',
track: 'Learn WordPress',
achievements: '40',
points: '1950'
},
{
name: 'Trish',
track: 'Rails Development',
achievements: '5',
points: '350'
}
];
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
var studentData = '';
for (var i = 0; i < students.length; i += 1) {
studentData += '<h1>' + students[i].name + '</h1>';
for(var key in students[i]) {
studentData += '<p>';
studentData += key + ': ' + students[i][key];
}
studentData += '</p>';
}
print(studentData);
I used a for-in loop to get each property names and values inside a for loop.
1 Answer
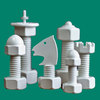
Steven Parker
230,274 PointsStudents missing data might not be as easily recognized this way.
If a data item was missing in a student record, the method shown in the example would still print out the keyword for the item and indicate it as "undefined". By iterating the existing keys instead, your output would just omit the missing key and data entirely.
Another minor difference is that the order of items returned from a for..in loop is not guaranteed, so even with no database errors the output order may not be the same.
Hyeon Kwang Choi
5,522 PointsHyeon Kwang Choi
5,522 PointsI updated my code with addition of students data.
and I don't understand why the order of items returned from a for..in loop is not guaranteed. could you explain it little bit further?
Steven Parker
230,274 PointsSteven Parker
230,274 PointsTry deleting one or more data lines from the students to see an example of what I was saying.
Hyeon Kwang Choi
5,522 PointsHyeon Kwang Choi
5,522 PointsI deleted two object literals and few data lines, but i don't see any problems as in order of items.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsWhat you should notice is that the categories (keys) will be missing from the output. But if you use the teacher's code from the video they would be shown with "undefined" as the value.