Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial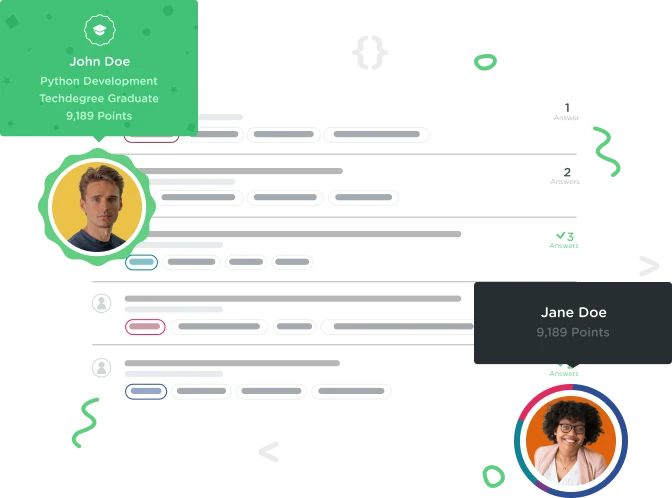

Shaun Kelly
5,648 PointsI have four questions that I really want to learn about with regards mainly to images!
QUESTION ONE: I have an app that is an image of a cartoon face and i'm trying to make it play different animations depending on which button you press. So the first button plays a certain animation then the second button plays a different animation etc. Here's my code but i'm having trouble starting the animation in the IBAction Button?
ViewController.h
#import <UIKit/UIKit.h>
@interface ViewController : UIViewController
- (IBAction)hatButton;
- (IBAction)winkButton;
- (IBAction)lickButton;
@property (strong, nonatomic) IBOutlet UIImageView *backgroundImageView;
@property (strong, nonatomic) NSArray *winkAnimation;
@property (strong, nonatomic) NSArray *lickAnimation;
@property (strong, nonatomic) NSArray *hatAnimation;
@end
ViewController.m
#import "ViewController.h"
#import "class.h"
@interface ViewController ()
@end
@implementation ViewController
- (void)viewDidLoad {
[super viewDidLoad];
//HAT ANIMATION
NSArray *hatAnimation = self.backgroundImageView.animationImages = [[NSArray alloc] initWithObjects:[UIImage imageNamed:@"h1"],
[UIImage imageNamed:@"h2"],
[UIImage imageNamed:@"h3"],
[UIImage imageNamed:@"h4"],
[UIImage imageNamed:@"h5"],
[UIImage imageNamed:@"h6"],
[UIImage imageNamed:@"h7"],
[UIImage imageNamed:@"h8"],
[UIImage imageNamed:@"h9"],
[UIImage imageNamed:@"h10"],
[UIImage imageNamed:@"h11"],
[UIImage imageNamed:@"h12"],
[UIImage imageNamed:@"h13"],
[UIImage imageNamed:@"h14"],
[UIImage imageNamed:@"h15"],
[UIImage imageNamed:@"h16"],
[UIImage imageNamed:@"h17"],
[UIImage imageNamed:@"h18"],nil];
self.backgroundImageView.animationDuration = 3.0f;
self.backgroundImageView.animationRepeatCount = 1;
//WINK ANIMATION
NSArray *winkAnimation = self.backgroundImageView.animationImages = [[NSArray alloc] initWithObjects:[UIImage imageNamed:@"w1"],
[UIImage imageNamed:@"w2"],
[UIImage imageNamed:@"w3"],
[UIImage imageNamed:@"w4"],
[UIImage imageNamed:@"w5"],
[UIImage imageNamed:@"w6"],
[UIImage imageNamed:@"w7"],
[UIImage imageNamed:@"w8"],
[UIImage imageNamed:@"w9"],
[UIImage imageNamed:@"w10"],
[UIImage imageNamed:@"w11"],
[UIImage imageNamed:@"w12"],
nil];
self.backgroundImageView.animationDuration = 3.0f;
self.backgroundImageView.animationRepeatCount = 1;
//LICK ANIMATION
NSArray *lickAnimation = self.backgroundImageView.animationImages = [[NSArray alloc] initWithObjects:[UIImage imageNamed:@"l1"],
[UIImage imageNamed:@"l2"],
[UIImage imageNamed:@"l3"],
[UIImage imageNamed:@"l4"],
[UIImage imageNamed:@"l5"],
[UIImage imageNamed:@"l6"],
[UIImage imageNamed:@"l7"],
[UIImage imageNamed:@"l8"],
[UIImage imageNamed:@"l9"],
[UIImage imageNamed:@"l10"],
[UIImage imageNamed:@"l11"],
[UIImage imageNamed:@"l12"],
[UIImage imageNamed:@"l13"],
[UIImage imageNamed:@"l14"],
[UIImage imageNamed:@"l15"],
[UIImage imageNamed:@"l16"],
[UIImage imageNamed:@"l17"],
[UIImage imageNamed:@"l18"],nil];
self.backgroundImageView.animationDuration = 3.0f;
self.backgroundImageView.animationRepeatCount = 1;
}
- (void)didReceiveMemoryWarning {
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (IBAction)hatButton {
[self.backgroundImageView startAnimating[self.hatAnimation]];
}
- (IBAction)winkButton {
[self.backgroundImageView startAnimating[self.winkAnimation]];
}
- (IBAction)lickButton {
[self.backgroundImageView startAnimating[self.lickAnimation]];
}
@end
QUESTION TWO: Can you create a circle button or a different shaped button rather than the default squared shaped button in Xcode 6? If so, how?
QUESTION THREE: Is it possible to create an image as a button itself ? So when you press on the image it acts as a button? The only possible way I would come across this is... Have the image under a invisible button. But then those are two separate things which I don't really want.
QUESTION FOUR: How would I download and import different objects in the object library for Xcode 6.
3 Answers

Stone Preston
42,016 Pointsyour problem is that in viewDidLoad you just keep reassigning the animation images property of your image view. if you want it to be a different animation each button press, you are going to need to reassign the animationImages property inside each IBAction.
you are also calling the startAnimating method incorrectly. it does not take any arguments.
below is the code for your hat animation. the other actions will look similar. you just need to set the imageView animation properties and then start the animation
- (IBAction)hatButton {
//HAT ANIMATION
self.backgroundImageView.animationImages = [[NSArray alloc] initWithObjects:[UIImage imageNamed:@"h1"],
[UIImage imageNamed:@"h2"],
[UIImage imageNamed:@"h3"],
[UIImage imageNamed:@"h4"],
[UIImage imageNamed:@"h5"],
[UIImage imageNamed:@"h6"],
[UIImage imageNamed:@"h7"],
[UIImage imageNamed:@"h8"],
[UIImage imageNamed:@"h9"],
[UIImage imageNamed:@"h10"],
[UIImage imageNamed:@"h11"],
[UIImage imageNamed:@"h12"],
[UIImage imageNamed:@"h13"],
[UIImage imageNamed:@"h14"],
[UIImage imageNamed:@"h15"],
[UIImage imageNamed:@"h16"],
[UIImage imageNamed:@"h17"],
[UIImage imageNamed:@"h18"],nil];
self.backgroundImageView.animationDuration = 3.0f;
self.backgroundImageView.animationRepeatCount = 1;
[self.backgroundImageView startAnimating];
}

Stone Preston
42,016 Pointsyour problem is that in viewDidLoad you just keep reassigning the animation images property of your image view. if you want it to be a different animation each button press, you are going to need to reassign the animationImages property inside each IBAction.
you are also calling the startAnimating method incorrectly. it does not take any arguments.
below is the code for your hat animation. the other actions will look similar. you just need to set the imageView animation properties and then start the animation
- (IBAction)hatButton {
//HAT ANIMATION
self.backgroundImageView.animationImages = [[NSArray alloc] initWithObjects:[UIImage imageNamed:@"h1"],
[UIImage imageNamed:@"h2"],
[UIImage imageNamed:@"h3"],
[UIImage imageNamed:@"h4"],
[UIImage imageNamed:@"h5"],
[UIImage imageNamed:@"h6"],
[UIImage imageNamed:@"h7"],
[UIImage imageNamed:@"h8"],
[UIImage imageNamed:@"h9"],
[UIImage imageNamed:@"h10"],
[UIImage imageNamed:@"h11"],
[UIImage imageNamed:@"h12"],
[UIImage imageNamed:@"h13"],
[UIImage imageNamed:@"h14"],
[UIImage imageNamed:@"h15"],
[UIImage imageNamed:@"h16"],
[UIImage imageNamed:@"h17"],
[UIImage imageNamed:@"h18"],nil];
self.backgroundImageView.animationDuration = 3.0f;
self.backgroundImageView.animationRepeatCount = 1;
[self.backgroundImageView startAnimating];
}
you can create square buttons with circles as background (the touch area will be square, but the button will look like a circle.
you can assign images to the background of buttons as well.

Shaun Kelly
5,648 Pointshey thanks so much! :D