Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial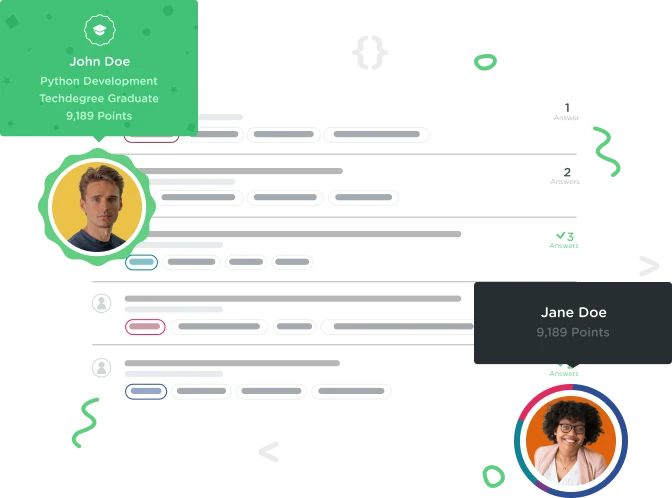
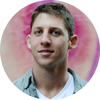
Robert Crocker
3,748 PointsI have gotten task 1 to work several different ways, but every time I get back to task three my task 1 no longer works.
I'm sure I'm removing the elements incorrectly, but it's hard to troubleshoot that task without understanding how task 1 mysteriously breaks.
I would appreciate someone helping me to understand where I'm going wrong here.
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<ul>
<li id="first">First Item</li>
<li id="second">Second Item</li>
<li id="third">Third Item</li>
</ul>
<script src="app.js"></script>
</body>
</html>
let myList;
let firstListItem;
myList = document.getElementsByTagName("ul")[0];
firstListItem = myList.querySelector("li");
myList.removeChild("li");
2 Answers
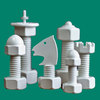
Steven Parker
229,744 PointsThe argument for removeChild is an element, not a selector string.
And in task 2, you have already selected the element and saved it. So for task 3 you could write:
myList.removeChild(firstListItem);
Using the wrong kind of argument causes a syntax error for the entire program, so the challenge says "Task 1 is no longer passing." because it checks task 1 again first.
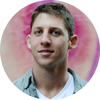
Robert Crocker
3,748 PointsHere I'm passing in an element, but task 1 breaks again. How does my code work for task 1 and 2, but not 3?
let myList; let firstListItem; myList = document.getElementsByTagName("ul")[0]; firstListItem = myList.querySelector("li"); myList.removeChild("li");
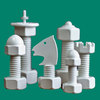
Steven Parker
229,744 Points"li" is a selector string.
It works as an argument to querySelector, but not for removeChild. Take another look at my answer for an example of a valid call to removeChild.
Robert Crocker
3,748 PointsRobert Crocker
3,748 PointsHere I'm passing in an element, but task 1 breaks again. How does my code work for task 1 and 2, but not 3?
let myList; let firstListItem;
myList = document.getElementsByTagName("ul")[0];
firstListItem = myList.querySelector("li");
myList.removeChild("li");
Kevin Korte
28,148 PointsKevin Korte
28,148 PointsHey Robert, take a good look again at what Steven is saying. Also, take note that is says "first list item element". You'll need to select your list item in a similar way you did your unordered list in step 1. Once you have the first list item in your variable, than call that in your removeChild method, not the string, like Steven showed.
If you do that, it'll pass you.