Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial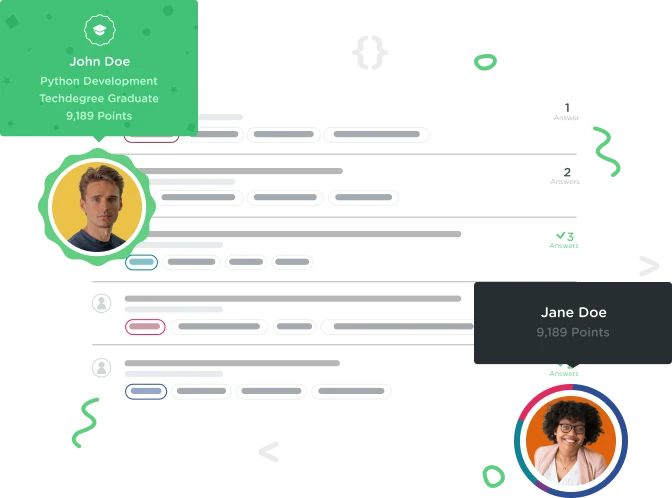

Darnell Cephus
4,208 PointsI have key and string parameters, why the error?
I have key and string parameters, why the error?
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends Activity {
public static final String EXTRA_YEAR = "EXTRA_YEAR";
public Button goButton;
public EditText yearField;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
goButton = (Button)findViewById(R.id.goButton);
yearField = (EditText)findViewById(R.id.yearField);
goButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Add your code in here!
Intent intent = new Intent(MainActivity.this, TimeTravelActivity.class);
intent.putExtra(EXTRA_YEAR, yearField.getText());
startActivity(intent);
}
});
}
}
import android.os.Bundle;
import android.view.View;
public class TimeTravelActivity extends Activity {
public String targetYear;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_time_travel);
}
}
2 Answers

Simon Coates
28,694 PointsFor any future users, the following seems to pass the challenge completely:
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class MainActivity extends Activity {
public Button goButton;
public EditText yearField;
public static final String EXTRA_YEAR = "EXTRA_YEAR";
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
goButton = (Button)findViewById(R.id.goButton);
yearField = (EditText)findViewById(R.id.yearField);
goButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(MainActivity.this,TimeTravelActivity.class);
String year = new String();
year = yearField.getText().toString();
intent.putExtra(EXTRA_YEAR, year);
startActivity(intent);
}
});
}
}
and
import android.os.Bundle;
import android.view.View;
public class TimeTravelActivity extends Activity {
public String targetYear;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_time_travel);
targetYear = getIntent().getStringExtra(MainActivity.EXTRA_YEAR );
}
}

Seth Kroger
56,415 PointsIt's one of those tricky points of Android, but EditText.getText() doesn't quite give you a String. It gives you something called an Editable and you have to use toString() on it to get a true String.

Darnell Cephus
4,208 PointsI'm trying to get use to thinking logically about this...but this was the correct answer. Correct me if I'm wrong Seth lol
..... goButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Add your code in here!
Intent intent = new Intent(MainActivity.this,TimeTravelActivity.class);
String year = new String();
year = yearField.getText().toString();
intent.putExtra(EXTRA_YEAR, year);
startActivity(intent);
}
});
}