Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial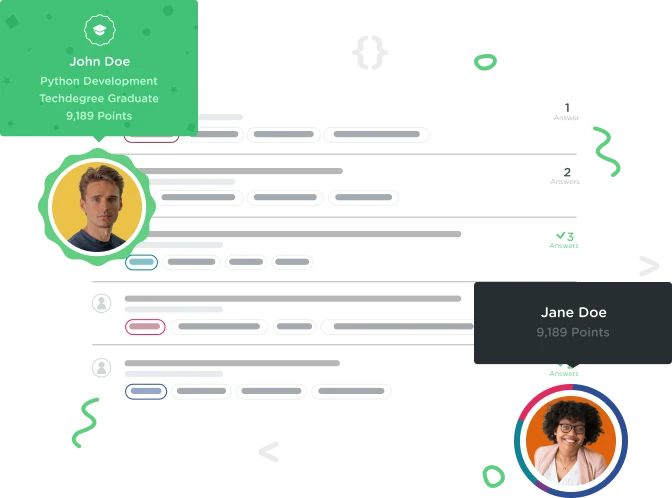
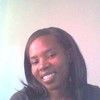
Colletah Mavunduse
5,414 Pointsi have modified the foreach loop but still its saying its wrong
The array below contains a list of books. Each element in the array has the book’s title as its value and the ISBN as its key. Right now, the page is only displaying the book titles in the browser. In this code challenge, we will modify the page to also display each book’s ISBN. First, we need to make the keys from the books array accessible inside the foreach loop. Modify the foreach command so that, as it loops through the books, it loads the ISBN for each book into a working variable called $isbn.
<?php
$books["978-0743261690"] = "Gilgamesh";
$books["978-0060931957"] = "The Odyssey";
$books["978-0192840509"] = "Aesop's Fables";
$books["978-0520227040"] = "Mahabharta";
$books["978-0393320978"] = "Beowulf";
?><html>
<head>
<title>Five Great Books</title>
</head>
<body>
<h1>Five Great Books</h1>
<ul>
<?php foreach($books as $book=>$isbn) { ?>
<li><?php echo $book . " " . "$isbn"; ?></li>
<?php } ?>
</ul>
</body>
</html>
3 Answers
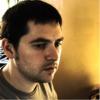
geoffrey
28,736 PointsYou were close, but what you need to do is this to access the keys of your associative array.
<?php
$books["978-0743261690"] = "Gilgamesh";
$books["978-0060931957"] = "The Odyssey";
$books["978-0192840509"] = "Aesop's Fables";
$books["978-0520227040"] = "Mahabharta";
$books["978-0393320978"] = "Beowulf";
?>
<html>
<head>
<title>Five Great Books</title>
</head>
<body>
<h1>Five Great Books</h1>
<ul>
<?php foreach($books as $isbn => $book) { ?>
<li><?php echo $book." ".$isbn; ?></li>
<?php } ?>
</ul>
</body>
</html>
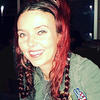
Juliette Tworsey
Front End Web Development Techdegree Graduate 32,425 PointsThis is what worked for me:
<?php foreach($books as $isbn=>$book) { ?> <li><?php echo $book. " " .$book[$isbn]; ?></li> <?php } ?>
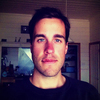
Sam Donald
36,305 PointsYou're all close but it asks for the $isbn
to display in parentheses, like so
<?php foreach($books as $isbn => $book) { ?>
<li><?php echo $book." "."(".$isbn.")"; ?></li>
<?php } ?>
Which will output Gilgamesh (978-0743261690)
Colletah Mavunduse
5,414 PointsColletah Mavunduse
5,414 Points<li><?php echo $book . " " . "($isbn)"; ?></li>