Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial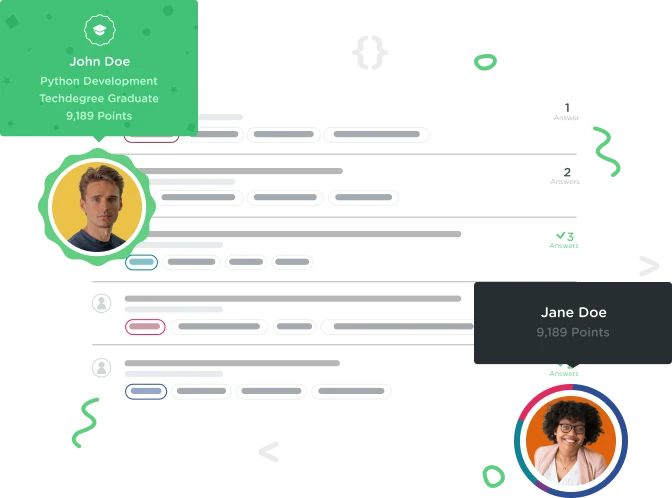

christian elam
6,443 PointsI have no clue how to figure this out!!??
I am really confused and have no idea how to complete this challenge could you help me please!!?????
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! Im a machine!")
}
}
// Enter your code below

christian elam
6,443 PointsAnd by the way does anyone know how to skip a ending challenge such as this because other challenges that are in the middle of your courses and classes you can just click the next one but since this is on the end you can't skip it or atleast I dont know how
1 Answer
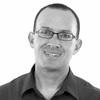
David Papandrew
8,386 PointsHi Christian,
Here's the code:
class Robot: Machine {
override func move(direction: String) {
switch direction {
case "Up": self.location.y += 1
case "Down": self.location.y -= 1
case "Right": self.location.x += 1
case "Left": self.location.x -= 1
default: print("Do nothing") // Or you could put a break clause per the instructions
}
}
}
Some explanations:
1) The first line defines a subclass of Machine
2) The 2nd line creates a class method "move" that overrides the move method of the Machine parent class (remember that due to inheritance, we need to "override" the move function if we want the subclass to have its own behavior).
3) We use a switch statement to check for all the move inputs (which we receive as a parameter "direction")
4) Up/Down directions will change our y coordinate. As you recall, the Machine class defines these.
5) Right/Left changes the x coordinate for the object
Hope that helps.

christian elam
6,443 PointsTHANK YOU SO MUCH!!!! I didn't realize I had to make separate classes for that. THANK YOU FOR HELPING ME I REALLY APPRECIATE IT!!!!!!!!!
christian elam
6,443 Pointschristian elam
6,443 PointsHere are the directions below
In the editor you've been provided with two classes - Point to represent a coordinate point and Machine. The machine has a move method that doesn't do anything because most machines are motionless.
Your task is to subclass Machine and create a new class named Robot. In the Robot class, override the move method and provide the following implementation. If you enter the string "Up" the y coordinate of the Robot's location increases by 1. "Down" decreases it by 1. If you enter "Left", the x coordinate of the location property decreases by 1 while "Right" increases it by 1.
Note: If you use a switch statement you can use the break statement in the default clause to exit the current iteration.