Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial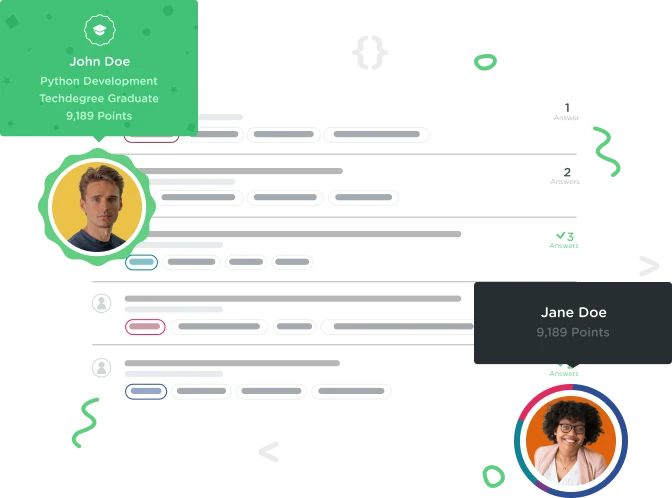

Bethany Sell
9,511 PointsI have no errors coming up in the preview window, and my code works in the playground. Am I overcomplicating this?
Is this correct, have I added too much to this, or did I not understand the question?
struct Tag {
let name: String
init(name: String){
self.name = name
}
}
struct Post {
var title: String
var author: String
var tag: Tag
init(title: String, author: String, name: String){
self.title = title
self.author = author
self.tag = Tag(name: name)
}
func description() ->String{
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let firstPost = Post(title: "iOSDevelopment", author: "Apple", name: "swift")
let postDescription = firstPost.description()
1 Answer
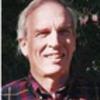
jcorum
71,830 PointsWhen I run your code through the challenge it passes Task 1 but fails Task 2 with a misleading error message: "Make sure you're declaring an instance method named description that returns a String".
When I test your code in Xcode it works fine. Which has to be frustrating.
I've found that the Treehouse editor is very picky sometimes. In particular, that code that works in Xcode isn't accepted by the editor for some reason or another. It's hard to guess in your case, but here's code that works:
struct Tag {
let name: String
}
struct Post {
let title: String
let author: String
let tag: Tag
func description() -> String {
return "\(title) by \(author). Filed under \(tag.name)"
}
}
let tag = Tag(name: "swift")
let firstPost = Post(title: "iOS Development", author: "Apple", tag: tag)
let postDescription = firstPost.description()
Note that you did not need to write initializers for either Tag or Post. The default ones work fine. And that may have been enough to jinx the editor.
It may be expecting a Tag in this line of code:
let firstPost = Post(title: "iOSDevelopment", author: "Apple", name: "swift")
Yours works, but you give it a String, rather than a Tag.