Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial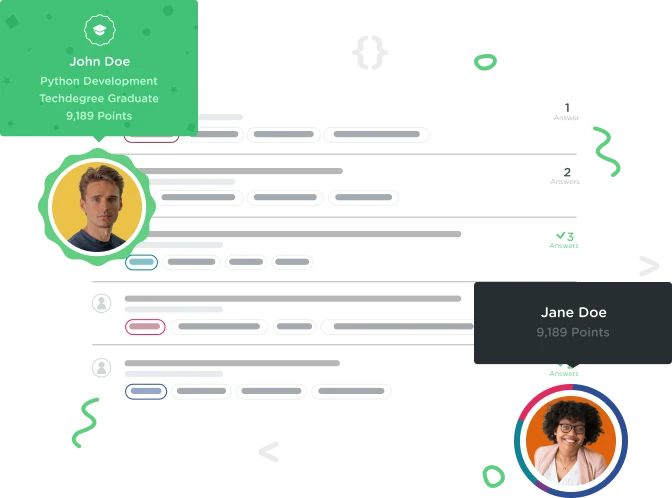

Leo Marco Corpuz
18,975 PointsI have no idea how to do the last part of this challenge.
I have no idea how to do this part of the challenge. I'm lost. I've tried to solve this but it doesn't make any sense.
public class Forum {
private String topic;
// TODO: add a constructor that accepts a topic and sets the private field topic
public Forum(String topic){
this.topic=topic;
}
public String getTopic() {
return topic;
}
public void addPost(ForumPost post) {
System.out.printf("A new post in %s topic from %s %s about %s is available",
topic,
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle()
);
}
}
public class User {
// TODO: add private fields for firstName and lastName
private String firstName;
private String lastName;
public User(String firstName, String lastName) {
// TODO: set and add the private fields
this.firstName=firstName;
this.lastName=lastName;
}
// TODO: add getters for firstName and lastName
public String getFirstName(){
return firstName;
}
public String getLastName(){
return lastName;
}
}
public class ForumPost {
private User author;
private String title;
private String description;
// TODO: add a constructor that accepts the author, title and description
private ForumPost(User author,String title,String description){
this.author=author;
this.title=title;
this.description=description;
}
public User getAuthor() {
return author;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
User author = new User();
// TODO: initialize the forum post with the user created above and a title and description of your choice
this.lastName=lastName;
ForumPost post = new ForumPost();
forum.addPost(post);
}
}
2 Answers
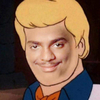
Daniel Turato
Java Web Development Techdegree Graduate 30,124 PointsHere's what I got for Main.java, this should work:
public class Main {
public static void main(String[] args) {
System.out.println("Beginning forum example");
if (args.length < 2) {
System.out.println("Usage: java Main <first name> <last name>");
System.err.println("<first name> and <last name> are required");
System.exit(1);
}
Forum forum = new Forum("Java");
// TODO: pass in the first name and last name that are in the args parameter
User author = new User(args[0], args[1]);
// TODO: initialize the forum post with the user created above and a title and description of your choice
ForumPost post = new ForumPost(author, "TestTitle", "TestDesc");
forum.addPost(post);
}
}
When passing arg's in java through the Main method, it puts it into a String array. In this case, the firstName and lastName are passed into the 1st and 2nd elements of the string array. To access them, all you have to do is go args[?] where the ? represents the specific index you want. Hope this helps
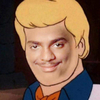
Daniel Turato
Java Web Development Techdegree Graduate 30,124 PointsWell the first thing they did was this:
post.getAuthor();
What this does is access the Forum Post object and then calls the getAuthor() method. This method then returns a User object which is the user who created the post. From there you can do this:
getFirstName();
This will then return the first name of the User using the getFirstName() method.

Leo Marco Corpuz
18,975 PointsThanks!
Leo Marco Corpuz
18,975 PointsLeo Marco Corpuz
18,975 PointsThanks!
But can you explain this line of code from the Forum class: post.getAuthor().getFirstName(), post.getAuthor().getLastName() How is the getFirstName and getLastName method being accessed without the User class and I don't understand how they're combining different methods through dot notation.