Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial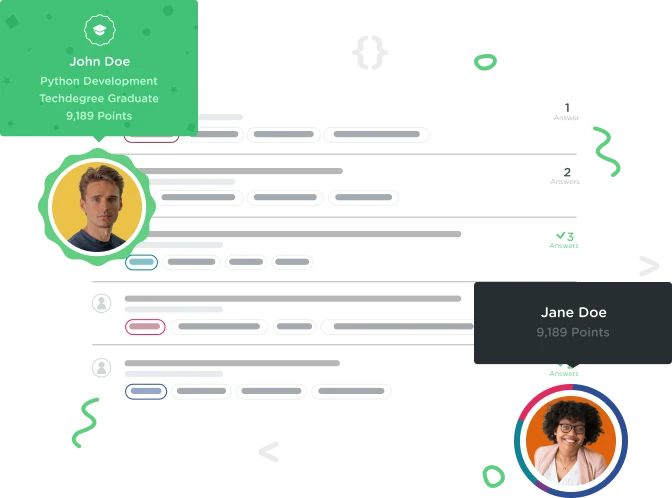

Marko Borenovic
1,813 PointsI have no idea how to do this
I really have no idea how to do this challenge
# The dictionary will look something like:
# {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
# 'Kenneth Love': ['Python Basics', 'Python Collections']}
#
# Each key will be a Teacher and the value will be a list of courses.
#
# Your code goes below here.
def num_teachers(teachers):
count = 0
for amount in teachers.keys():
count += 1
return count
def num_courses(courses):
count = 0
for value in courses.values():
count += len(value)
return count
def courses(list_courses):
names = []
for value in list_courses.values():
names.extend(value)
return names
def most_courses(most):
for value in len(most.values()):
print (keys(max(value)))
3 Answers

Noë Snaterse
Courses Plus Student 5,581 PointsIt depends a little whether you are using Python2 or Python3 but here is some pseudocode to get you started.
teachers = {'Andrew Chalkley': ['jQuery Basics', 'Node.js Basics'],
'Kenneth Love': ['Python Basics', 'Python Collections']}
for name, courses in teachers.iteritems(): # python2
num = len(courses)
print name, num
for name, courses in teachers.items(): # python3
num = len(courses)
print name, num
Then just keep track of the teacher which has the highest num
.

Noë Snaterse
Courses Plus Student 5,581 PointsWhat is the question? That code is that given or did you write is yourself?

Marko Borenovic
1,813 PointsCreate a function named most_courses that takes our good ol' teacher dictionary.
most_courses should return the name of the teacher with the most courses. You might need to hold onto some sort of max count variable.
This is the question. It is fourth step out of 5
Marko Borenovic
1,813 PointsMarko Borenovic
1,813 PointsThank you very much