Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial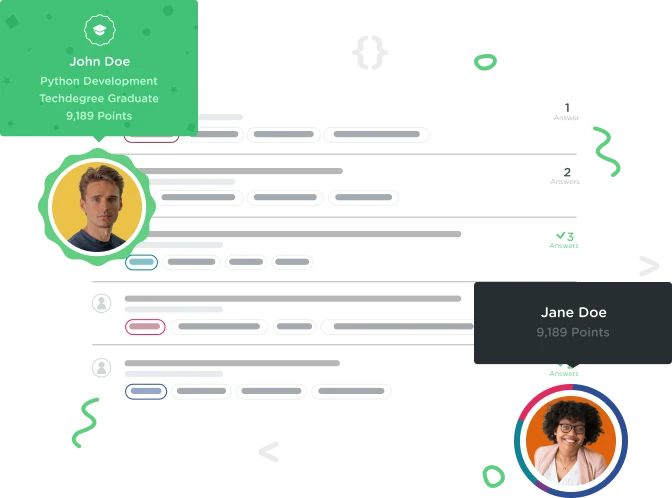

Hussein Amr
2,461 PointsI have no idea how to solve this
any ideas?
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
5 Answers
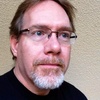
Chris Freeman
Treehouse Moderator 68,441 PointsHere are the steps:
- Create a function named combo that takes two ordered iterables:
def combo(iter1, iter2):
- Create a list of tuples, where each tuple contains one item from each iterable argument
- This will, perhaps, need a loop to gather the items from both iterables and create the tuples
- Return the list of tuples
Comments in the code give an example of the call and return values:
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
Post back if you need more help. Good luck!!

Hussein Amr
2,461 PointsChris Freeman so how do i use a as an index to b after i enumerate it?
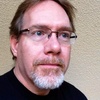
Chris Freeman
Treehouse Moderator 68,441 PointsAs mentioned in the docs, enumerate will return an index and an item from a
:
>>> seasons = ['Spring', 'Summer', 'Fall', 'Winter']
>>> list(enumerate(seasons))
[(0, 'Spring'), (1, 'Summer'), (2, 'Fall'), (3, 'Winter')]
# example usage
>>> for index, season in enumerate(seasons):
... print(index, season)
...
0 Spring
1 Summer
2 Fall
3 Winter
So, index
could be used to reference b
, as in, b[index]

Hussein Amr
2,461 PointsChris Freeman ok so i understood what you're saying but I can't really apply it the right way
def combo(a, b):
list = []
for index, letter in enumerate(a):
print(index, letter)
list_cont = list.append((a , b[index]))
return list_cont
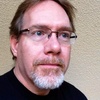
Chris Freeman
Treehouse Moderator 68,441 PointsGetting closer.
- The print statement is not needed. (I used it as an example only)
- don't use
list
as a variable name. It is a built-in object type. By assigning tolist
you make it a local object which can mess up other usages oflist()
. -
append()
operates on a list in-place and returns "None", solist_cont
would only beNone
. Remove the assignment and only use the append method directly on <what every your rename `list` to>. - indent the append to be inside the for loop
- the first argument to the append should be
letter
and not the full lista
. - return <what every you rename `list` to>
That should do it. Get trying!!

Hussein Amr
2,461 PointsChris Freeman I finally got it Chris! Thank you very much. I'm really disappointed though because i wasn't even close to the answer and it usually takes me a whole lot of time to get through code challenges :/

Hussein Amr
2,461 PointsChris Freeman I don't get how the b[index] thing worked. can you further explain it?
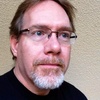
Chris Freeman
Treehouse Moderator 68,441 Pointsb
is the second iterable so b[index]
uses the index to look up the corresponding item in b
that was in a
.

sage georgi
2,005 PointsMy question is why they blocked the zip method dangit
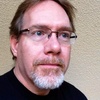
Chris Freeman
Treehouse Moderator 68,441 PointsYes. Using zip
makes the solution too easy. The point of the exercise is to learn to iterate over lists.
Hussein Amr
2,461 PointsHussein Amr
2,461 PointsChris Freeman take a look at my code and tell me what's wrong
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsYou cannot have two
in
statements in the for loop.One solution involves using
enumerate(a)
to get an index and one item froma
, then use the index to get the matching item from b usingb[index]
. See more aboutenumerate
in the docs.