Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial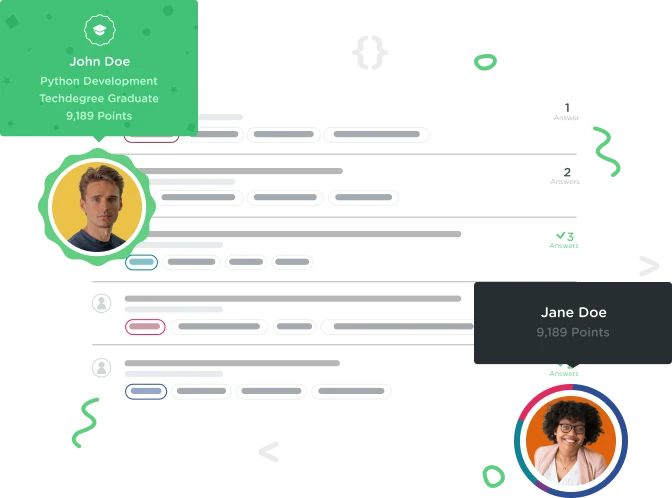

Ryan Anderson
2,920 PointsI have no idea what's being asked here.
Here's what I've done based on the instructions, but I have absolutely no idea what the question being asked here is. Some direction would be greatly appreciated.
struct RGBColor {
let red: Double
let green: Double
let blue: Double
let alpha: Double
let description: String
// Add your code below
init (red: Double, green: Double, blue: Double, alpha: Double, description: String) {
self.red = 86.0
self.green = 191.0
self.blue = 131.0
self.alpha = 1.0
self.description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}
}

Ryan Anderson
2,920 PointsI presumed there would be a link to the challenge created by default. The question is in reference to this one: https://teamtreehouse.com/library/objectoriented-swift-20/complex-data-structures/custom-initializers
4 Answers
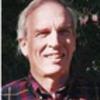
jcorum
71,830 PointsRyan, thanks for the link. You're very close. The only issue is that description is to be calculated inside the initializer using the passed in values for red, blue, green and alpha, rather than being passed in itself as a formal parameter:
init(red: Double, green: Double, blue: Double, alpha: Double) {
self.red = red
self.green = green
self.blue = blue
self.alpha = alpha
description = "red: \(red), green: \(green), blue: \(blue), alpha: \(alpha)"
}

Ryan Anderson
2,920 PointsThanks for this! That was my original solution but I didn't format description properly. I think I'm just a bit hazy on the point of init methods, so I'll do a bit of reading on that.
Why does description not need a "self" reference?
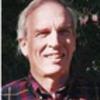
jcorum
71,830 Pointsself is used to disambiguate the local variable (formal parameter) red from the member variable (stored property) red. If you write red = red the compiler will complain. If you write self.red = red it doesn't, because it knows you want the member variable set to the passed in value. In the case of description there's no ambiguity. You're just saying assign a value to the member variable. Think of self as a pronoun that means "my," then self.red = red means my red should be assigned the passed in value red.

Ryan Anderson
2,920 PointsSo, if I follow correctly...
We init description because we don't pass it through the arguments for the struct, but technically speaking, nothing would change here if red was not init'ed unless it was assigned a value previously in the code?
This seems like a really simple concept, but for some reason I'm having a lot of trouble wrapping my brain around it, even after discussing it at length with my programmer friends.
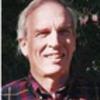
jcorum
71,830 PointsThe job of the initializer is to make sure all stored properties (member properties or instance properties in other programming languages) have values (are initialized).
Since description has to have a value, it can be given a value in one of three ways:
You can give it a value when you declare it:
let description: String = "...some text"
You can pass in a value for it via the initializer:
init(.... description: String)
Or you can compute the value inside the initializer:
description = "red: (red), green: (green), blue: (blue), alpha: (alpha)"
In this case you want the description to change depending on what values are passed in for red, green, blue and alpha, so you have to go the third way.

Ryan Anderson
2,920 PointsThanks! It's starting to become clear.
jcorum
71,830 Pointsjcorum
71,830 PointsRyan, it's difficult to help if we don't know what you are supposed to be doing. The best way is to include the link to the challenge. Absent that, copy in the instructions.