Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial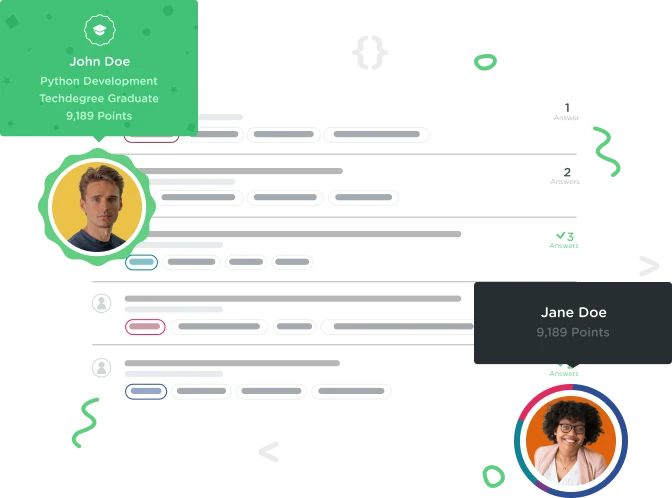
Trevor Wood
17,828 PointsI have no idea whats going on here
I'm pretty lost here. Can someone explain this code challenge question to me as if I were a small child?
Also, what is the end goal of this challenge? What are we trying to achieve with this code?
Thanks in advance for whomever answers :)
import Foundation
enum UIBarButtonStyle {
case Done
case Plain
case Bordered
}
class UIBarButtonItem {
var title: String?
let style: UIBarButtonStyle
var target: AnyObject?
var action: Selector
init(title: String?, style: UIBarButtonStyle, target: AnyObject?, action: Selector) {
self.title = title
self.style = style
self.target = target
self.action = action
}
}
enum Button {
case Done(String)
case Edit(String)
func toUIBarButtonItem() -> UIBarButtonItem{
}
}
let done = Button.Done("Done")
let doneButton = Button.Done("Done")
2 Answers
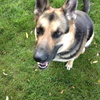
Eric Hodgins
29,207 PointsFrom the big picture this challenge I think is trying to show you how powerful Swift enums are. This task specifically is trying to accomplish a way to make a UIBarButtonItem easily through an enum method.
Looks like you got the first part of the challenge. But this is how I accomplished the second part:
enum Button {
case Done(String)
case Edit(String)
func toUIBarButtonItem() -> UIBarButtonItem {
switch self {
case .Done(let title):
return UIBarButtonItem(title: title, style: .Done, target: nil, action: nil)
case .Edit(let title):
return UIBarButtonItem(title: title, style: .Plain, target: nil, action: nil)
}
}
}
let done = Button.Done("Done")
let doneButton = done.toUIBarButtonItem()
With that func in the enum you can now easily make a UIBarButtonItem from any other Button enums that you make. Whether they're case Done or Edit...or any other custom cases made. Does that all make sense? If anythings unclear feel free to ask!
Ryan Doyle
8,587 PointsThat was so helpful! This took me so long to figure out just because I kept trying to figure out that in the world anything was actually making in the first place. I think once the challenges get this complex, it's much more helpful to know what's actually going on. Had the instruction just stated that the code was to take a IUButton and make it more friendly by converting it to an easier named Enum, this would have been so much easier. It also kind of relies on the fact that you'll just assume the string is a title.

psculthorpe
3,151 PointsTotally agree - I feel like a simple and less abstract explanation of the problem would have made me attempt to tackle it in a different way - thanks Eric Hodgins :)