Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial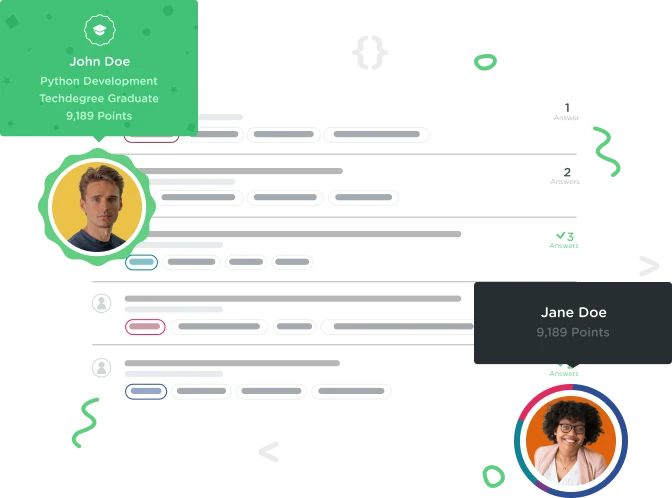

Tojo Alex
Courses Plus Student 13,331 PointsI have one tiny mistake though I'm not sure where it is
I have tried multiple times but it gives me the same error every time and sometimes I receive multiple errors.
namespace Treehouse.CodeChallenges
{
public class Frog
{
private int _numFliesEaten;
public int GetNumFliesEaten()
{
_numFliesEaten;
}
public int SetNumFliesEaten()
{
set
{
_numFliesEaten = value;
}
}
}
}
1 Answer

andren
28,558 PointsThere are actually a couple of issues:
- In your
GetNumFliesEaten
method you have forgotten to include thereturn
keyword when returning the_numFliesEaten
field. - The
SetNumFliesEaten
method should have a return type ofvoid
notint
, since it does not actually return any value. - Since
SetNumFliesEaten
is just a normal setter method (you are not dealing with a property here) you should not use theset
keyword. And you also cannot referencevalue
without defining it as a parameter first.
If you fix those issues like this:
namespace Treehouse.CodeChallenges
{
class Frog
{
private int _numFliesEaten;
public int GetNumFliesEaten()
{
// return keyword added
return _numFliesEaten;
}
// void set as return type and value added as parameter
public void SetNumFliesEaten(int value)
{
// set keyword and block removed
_numFliesEaten = value;
}
}
}
Then your code will pass.