Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial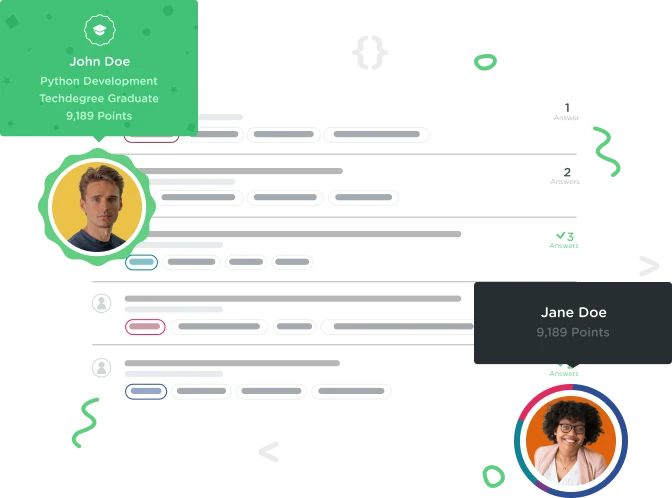

Angelica Islas
4,082 PointsI have questions about the curly brackets from the example. It doesn't look like they are all closed to me.
let correctGuess = false; const number = 6; const guess = prompt('Guess a number between 1 and 10.');
if ( +guess === number ) {
correctGuess = true;
} else if ( +guess < number ) {
const guessMore = prompt(Try again. The number is higher than ${guess}
);
if ( +guessMore === number ) {
correctGuess = true;
}
} else if ( +guess > number ) {
const guessLess = prompt(Try again. The number is lower than ${guess}
);
if ( +guessLess === number ) {
correctGuess = true;
}
}
if ( correctGuess ) {
console.log("You guessed the number!");
} else {
console.log(Sorry. The number was ${number}.
);
}
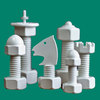
Steven Parker
231,269 PointsMark, a leading "+
" is one way of converting a string (like what comes in from "prompt
") into a number. And I think this particular example game is intended to allow only 2 guesses by design.
And technically, you can have more than one statement on a line, but it's not usually considered good practice.
1 Answer
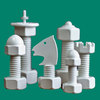
Steven Parker
231,269 PointsOne technique that helps visualize proper bracketing is to indent lines based on nesting level. Your code formatted in this way (and shown here using Markdown formatting) looks like this:
let correctGuess = false;
const number = 6;
const guess = prompt("Guess a number between 1 and 10.");
if (+guess === number) {
correctGuess = true;
} else if (+guess < number) {
const guessMore = prompt(`Try again. The number is higher than ${guess}`);
if (+guessMore === number) {
correctGuess = true;
}
} else if (+guess > number) {
const guessLess = prompt(`Try again. The number is lower than ${guess}`);
if (+guessLess === number) {
correctGuess = true;
}
}
if (correctGuess) {
console.log("You guessed the number!");
} else {
console.log(`Sorry. The number was ${number}.`);
}
The code itself seems fine and works correctly.
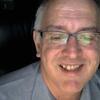
Mark Casavantes
2,880 PointsThank you Steven, I learn a lot from you every time you post and I appreciate learning from you.
Mark Casavantes
2,880 PointsMark Casavantes
2,880 PointsHello Angelica,
It looks like you put more than one line of code together. Every ";" should end a line. I see some double }} in your code. Eliminate one of the }. back ticks are needed on statements that have ${guess} or ${number}. Why is there a + sign in front of some variables? Change const to let for variable guess. A const variable cannot change once assigned. Instead of having variables guessMore and guessLess, just have a variable guess. Use guess < number for guessLess and guess > number for guessMore. You will need a loop to let people guess more than once. I do not know if I caught all of what you need to fix, but I hope I have helped you.