Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial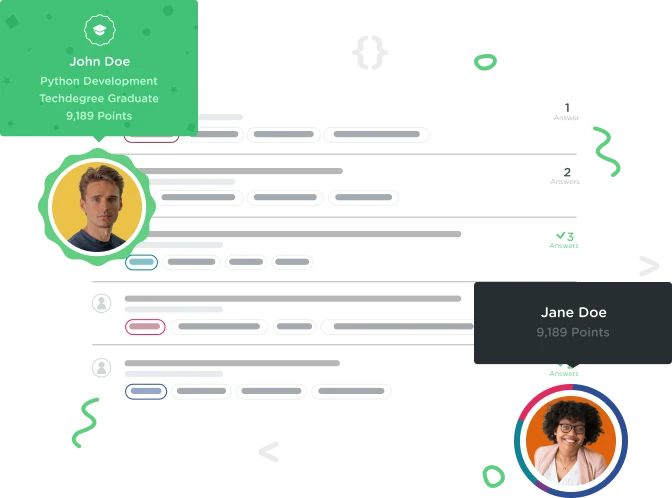

Sanketh Shanbhag
1,560 PointsI have tested this code; But it aint being accepted here ?
def disemvowel(word): words = list(word.lower()) print(words) for eachword in words: if eachword in ['a','e','i','o','u']: while True: try: words.remove(eachword) except: break word = str(words) return word
def disemvowel(word):
words = list(word.lower())
print(words)
for eachword in words:
if eachword in ['a','e','i','o','u']:
while True:
try:
words.remove(eachword)
except:
break
word = str(words)
return word
2 Answers
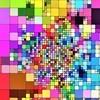
james south
Front End Web Development Techdegree Graduate 33,271 Pointsstr casting a list doesn't turn the list into a word. use the join method. also you have a print statement in your code, it needs to be removed unless the challenge asks you to print.

Mike Wagner
23,559 PointsOkay, so I've taken your code and run it through my interpreter. The first issue I see is that by the end of your for
loop you essentially are returning a list of letters, not a disemvoweled word. So the first thing you'd need to do is join the list together in one way or another. Another problem I potentially see is that you're casting word
to lower when you split it into a list, which means you're essentially modifying a word (e.g. "Treehouse") to always return lowercase, so you should move the .lower()
down into your if-in
check.
Also, it's worth noting that if you pass in a multiword string like
disemvowel("A quick brown fox jumps over the lazy dog")
your remove()
seems to fall apart and you will wind up with at least 1 vowel (in the example string it leaves the 'i' behind). It doesn't appear to be related to the try/except, as the problem persists even if the try/except is removed, but I can't certain. I'm not entirely sure if the Challenge passes in multiword iterables or not, but the challenge doesn't appear to like some combination of the remove()
or the join()
My brain can't seem to wrap around what the problem is, maybe someone with a bit more Python experience can explain the problem better.
As a solution I would, instead, create an empty string variable, then reverse your if-in
check to an if-not in
. If the letter in question is not in your list of vowels, a simple concatenation (i.e. newString += eachWord
using your variable name) and then returning the newString
at the end should get the job done.
I really have no idea why, but almost all of the problems I have seen people have with this challenge relate to using a list to break the string apart and then rejoining it together. I don't think I have seen a successful variation of doing this, but my knowledge is limited in this area, so I would love to find an answer.