Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial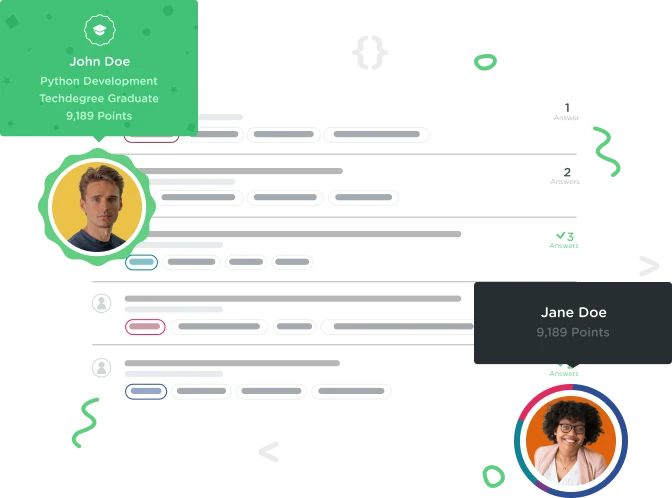

Yitzchak Zeitler
5,952 PointsI have the following challenge, so please help me complete it: https://w.trhou.se/eet5w1l847
I'm using the bootcamp.js. Ignore the var students at the top.
Thanks for your help!!
4 Answers

Yitzchak Zeitler
5,952 PointsThat's basically it. I did end up figuring it out, and my issue was that I was not calling the function to run.
Except for the sake of DRY:
var bowl = ["jelly-bean", "m&m", "m&m", "chocolate", "m&m", "jelly-bean", "m&m", "m&m", "jelly-bean"]
var jbOccur = 0; var mOccur = 0; var chocOccur = 0;
function occurrence() {
for( var i = 0; i < bowl.length; i += 1) {
if (bowl[i] === "jelly-bean") {jbOccur += 1;}
if (bowl[i] === "m&m") {mOccur += 1;}
if (bowl[i] === "chocolate") {chocOccur += 1;}
}}
occurrence();
console.log(jbOccur); console.log(mOccur); console.log(chocOccur);

NiKole Maxwell
11,083 PointsOHHHH!! Lol I'm sorry. I came up with the following after you updated more info.
My answer
Declarations
var bowl = ["jelly-bean", "m&m", "m&m", "chocolate",
"m&m", "jelly-bean", "m&m", "m&m", "jelly-bean"]
var jbOccur = 0;
var mOccur = 0;
var chocOccur = 0;
Start your function and for-loop
function occurrence() {
for ( var i = 0; i < bowl.length; i ++) {
// "++" and remove 1 to add +1 to the loop count
Deal with the Jellybeans first
if (bowl[i] === "jelly-bean") {
/*changed your bowl.length (a count of items in the bowl array)
TO analyze if the current item of the bowl array = the word, "jelly-bean" */
jbOccur += 1; //do this if bowl[i] is the same as this word ie "jelly-bean"
console.log('jbOccur + 1 now ', jbOccur); // log it out so you see it
Iterate through m&ms, still looping
} else if (bowl[i] === "m&m") { //continue the script
//if not "jellybean", then if its m&m add one to "m&m"
mOccur += 1; //add to m&m count
console.log('mOccur + 1 now ', mOccur); //log it out
Home stretch, accounting for chocolate
} else if (bowl[i] === "chocolate") {
//if not "m&m", then if "chocolate" + one
chocOccur += 1; //add to chocolate count
console.log('chocOccur + 1 now ', chocOccur); //log it out
}
}
}
Run it
occurrence (); //run the function
/*/print my totals /*/
console.log(' Total: jellybeans', jbOccur);
console.log(' Total: m&m\'s', mOccur);
console.log(' Total: chocolate', chocOccur);
I had to go but this got me interested, I actually made the start to a game with the first round of answers I was going to give you. This is what happens when you mix candy and code :O!

NiKole Maxwell
11,083 PointsUpdated to improve markdown quality of my answer.

NiKole Maxwell
11,083 PointsI don't have enough time atm to fully help with this one but I can tell you that your index.html file is missing the quiz.js script.
Add this to your html, based on the quick look I had, you should add it before the bootcamp.js to allow the entry field (question) to come first.
<script src="js/quiz.js"></script>
I'll check back in later if I can and someone else hasn't already answered.

Yitzchak Zeitler
5,952 PointsI'm not using quiz.js whatsoever.
Only concerned with the bootcamp.js
Yitzchak Zeitler
5,952 PointsYitzchak Zeitler
5,952 Points