Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial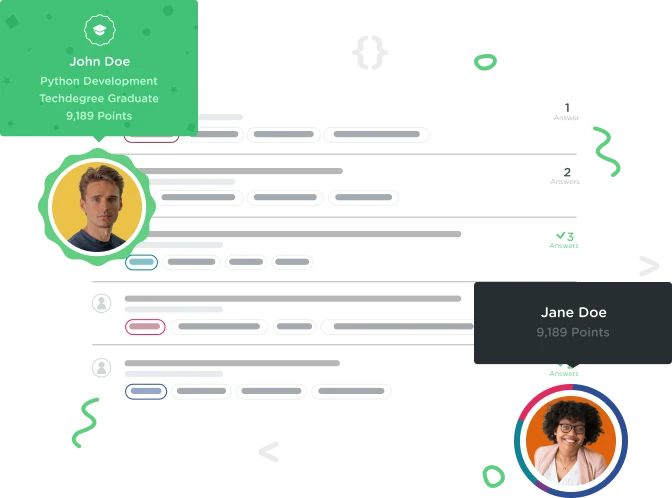

Braveo Yuriy
Courses Plus Student 5,763 PointsI haven't followed the Guil's actions. Do I need to get back and follow him, so that I can solve the task?
I haven't followed the Guil's actions. Do I need to get back and follow him, so that I can solve the task?
1 Answer

Tiffany Kwan
5,893 PointsI had the same problem since I like to take notes instead of following along as I watch. I went back and pieced together my notes for the html and js. Here is the bare bones of what you will need to try the challenge.
index.html
<!DOCTYPE html>
<html>
<head>
<title>JavaScript and the DOM</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">JavaScript and the DOM</h1>
<p>Making a web page interactive</p>
<div class="list">
<p>Things that are purple:</p>
<ul class="listUl">
<li>
grapes
<button class="down">Down</button>
<button class="up">Up</button>
<button class="remove">Remove</button>
</li>
<li>
peanuts
<button class="down">Down</button>
<button class="up">Up</button>
<button class="remove">Remove</button>
</li>
<li>
lavender
<button class="down">Down</button>
<button class="up">Up</button>
<button class="remove">Remove</button>
</li>
<li>
plums
<button class="down">Down</button>
<button class="up">Up</button>
<button class="remove">Remove</button>
</li>
</ul>
<button class="removeItemButton">Remove last item</button>
</div>
<script src="app.js"></script>
</body>
</html>
app.js
const listDiv = document.querySelector('.list');
const listUl = document.querySelector('ul');
const removeItemButton = document.querySelector('button.removeItemButton');
listUl.addEventListener('click', (event) => {
if (event.target.tagName == 'BUTTON') {
let li = event.target.parentNode;
let ul = li.parentNode;
if (event.target.className == 'remove') {
ul.removeChild(li); //Removes button's parent node (which is a list item)
}
if (event.target.className == 'up') {
let prevLi = li.previousElementSibling;
if(prevLi) {
ul.insertBefore(li, prevLi); //Moves the list item up
}
}
}
})
//Removes last item on the list
removeItemButton.addEventListener ('click', () => {
let ul = document.querySelectorAll('ul')[0];
let li = document.querySelector('li:last-child');
ul.removeChild(li);
})