Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial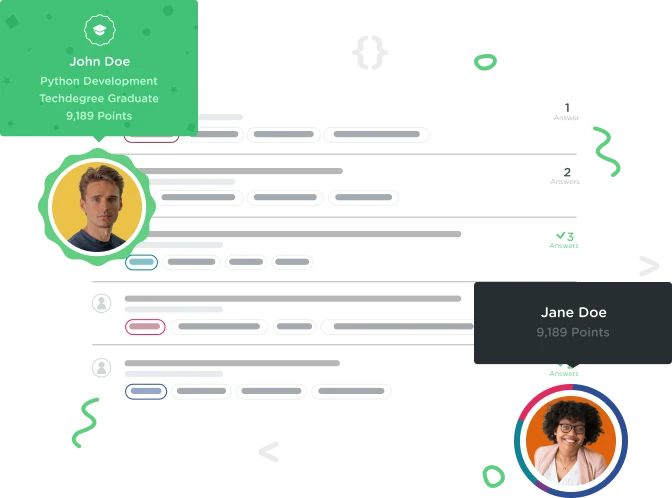
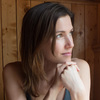
Rachel Miller
4,804 PointsI having trouble getting my code to work. Any thoughts would be greatly appreciated. Thanks!
//The below explains what I want the program to do...
//If the priority is high, and money is there, item can be bought
//If there are no more high priority items and money is available, then medium priority items can be bought
//If there are no more medium priority items and money is available, then low priority items can be bought
var amountToSpend = 8000;
var data = [("bed", 2000, "high"), ("faucet", 200, "low"), ("table", 2000, "medium"),("chair", 800, "high")];
//The for loops order the priority from high to low but fail to pull in all the data and spit it out to the input function. Any ideas on how to do this?
for(var i = 0; i< data.length; i++) {
if(data[i] === "high") {
console.log(data[i]);
}
}
for(var i = 0; i< data.length; i++) {
if(data[i] === "medium") {
console.log(data[i]);
}
}
for(var i = 0; i< data.length; i++) {
if(data[i] === "low") {
console.log(data[i]);
}
}
function input(item, amount, priority) {
if(amountToSpend >=0 && amount <= amountToSpend) {
if (priority === "high") {
amountToSpend -= amount;
console.log(item);
}
else if (priority === "medium") {
amountToSpend -= amount;
console.log(item);
}
else if (priority === "low") {
amountToSpend -= amount;
console.log(item);
}
else {
console.log("error");
}
}
return "Amount Left $" + amountToSpend;
}
3 Answers
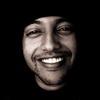
miikis
44,957 PointsHi Rachel,
What you're looking to do is best done with a sorting algorithm. One of the most efficient sorting algorithms is the MergeSort algorithm — another one is the Quick Sort algorithm. I used MergeSort here. This way it'll run faster and your code will be more succinct. Anyway, here's one way to do what you're trying to do:
var items = [
{
name : "bed",
cost : 2000,
priority : 1 //"high"
},
{
name : "faucet",
cost : 200,
priority : 3 //"low"
},
{
name : "table",
cost : 2000,
priority : 2 //"medium"
},
{
name : "chair",
cost : 800,
priority : 3 //"high"
}
];
function merge(left, right) {
var result = [],
lLen = left.length,
rLen = right.length,
l = 0,
r = 0;
while(l < lLen && r < rLen){
if(left[l].priority < right[r].priority) {
result.push(left[l++]);
}
else {
result.push(right[r++]);
}
}
//remaining part needs to be addred to the result
return result.concat(left.slice(l)).concat(right.slice(r));
}
function mergeSort(arr) {
var len = arr.length;
if(len <2)
return arr;
var mid = Math.floor(len/2),
left = arr.slice(0,mid),
right =arr.slice(mid);
//send left and right to the mergeSort to broke it down into pieces
//then merge those
return merge(mergeSort(left),mergeSort(right));
}
var whatShouldIBuy = function(possiblePurchases, cashInPocket) {
var theVerdict = "You should buy ", i;
var rankedPurchases = mergeSort(possiblePurchases).reverse();
var cashLeft = cashInPocket;
var shoppingList = [];
for(i=0; i<rankedPurchases.length; i++) {
if(rankedPurchases[i].cost <= cashLeft && cashLeft > 0) {
cashLeft -= rankedPurchases[i].cost;
shoppingList.push("the " + rankedPurchases[i].name);
}
}
if(shoppingList.length <= 0) {
return "Dude, you're broke. Go make some money!";
}
return theVerdict + shoppingList.join(" and ") + ". That will leave you with $" + cashLeft + " to keep your pocket warm!";
};
//console.log(whatShouldIBuy(items, 100)); //returns 'Dude, you're broke. Go make some money!'
//console.log(whatShouldIBuy(items, 500)); //returns 'You should buy the faucet. That will leave you with $300 to keep your pocket warm!'
//console.log(whatShouldIBuy(items, 1000)); //returns 'You should buy the faucet and the chair. That will leave you with $0 to keep your pocket warm!'
//console.log(whatShouldIBuy(items, 1500)); //returns 'You should buy the faucet and the chair. That will leave you with $500 to keep your pocket warm!'
//console.log(whatShouldIBuy(items, 3000)); //returns 'You should buy the faucet and the chair and the table. That will leave you with $0 to keep your pocket warm!'
//console.log(whatShouldIBuy(items, 5000)); //returns 'You should buy the faucet and the chair and the table and the bed. That will leave you with $0 to keep your pocket warm!'
To see it in action, simply call the whatShouldIBuy() function and give it an array of objects (your list of possible purchases) as the first argument and an Integer (the amount of cash in your pocket) as the second argument. Then, look in your browser's javascript console :)
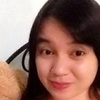
vanessa
3,455 PointsHope this helps..
var data1 = [
{saleItem : 'bed', price: 2000, priority : 1}, // 1 is high, 2 is medium, 3 is low priority
{saleItem : 'faucet', price: 200, priority : 3},
{saleItem : 'table', price: 2000, priority : 2},
{saleItem : 'chair', price: 800, priority : 1},
];
var totalData = data1.length - 1;
var canPurchase = true;
var listOfPurchaseable = [];
var customerPocket = prompt('How much is your budget?');
var rAmount = parseInt(customerPocket);
var output;
function print(message) { // print message
document.write(message);
}
// sort data by price descending & save to byPrice variable
var byPrice = data1.slice(0);
byPrice.sort(function(a,b) {
return b.price - a.price;
});
var lowestPricePossible = parseInt(byPrice[totalData].price);
// sort data by priority high to low & save to byPriority variable
var byPriority = data1.slice(0);
byPriority.sort(function(a,b) {
return a.priority - b.priority;
});
function getPossiblePurchase(customerPocket) {
if(parseInt(customerPocket) < parseInt(lowestPricePossible)) {
canPurchase = false;
}else {
for ( j=0; j < byPriority.length; j+=1) {
if(parseInt(customerPocket) >= parseFloat(byPriority[j].price)) {
listOfPurchaseable.push([byPriority[j].saleItem, byPriority[j].price, byPriority[j].priority]);
customerPocket -= parseFloat(byPriority[j].price);
byPriority.splice(j,1);
return customerPocket;
}
} // for ends
} //else ends
canPurchase = false;
return customerPocket;
} // getPossiblePurchase ends
// MAIN LOOP
while (canPurchase) { // loop while customerPocket is greater than lowest possible purchase item
if (rAmount >= lowestPricePossible) {
console.log(customerPocket);
rAmount = getPossiblePurchase(rAmount);
} else {
canPurchase = false;
}
}
// print list of possible purchase
output = ' Your budget is : ' + customerPocket + '<br>';
if (listOfPurchaseable.length > 0) {
for ( x=0; x < listOfPurchaseable.length; x++ ){
output += 'Item : ' + listOfPurchaseable[x][0] + '<br>';
output += 'Price : ' + listOfPurchaseable[x][1] + '<br>';
output += 'Priority : ' + listOfPurchaseable[x][2] + '<br><br>';
}
output += 'Remaining Amount : ' + rAmount;
} else {
output += ' Sorry your budget is too low ';
}
print(output);
/**
sample result
Your budget is : 4590
Item : bed
Price : 2000
Priority : 1
Item : chair
Price : 800
Priority : 1
Item : faucet
Price : 200
Priority : 3
Remaining Amount : 1590
**/
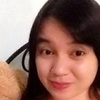
vanessa
3,455 Pointsi will try this!
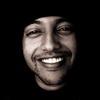
miikis
44,957 PointsHaha do it! Let me know what you think.
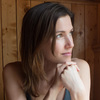
Rachel Miller
4,804 PointsThanks so much for taking the time to answer this. Lots of great ideas and new concepts to try to figure out :)!