Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial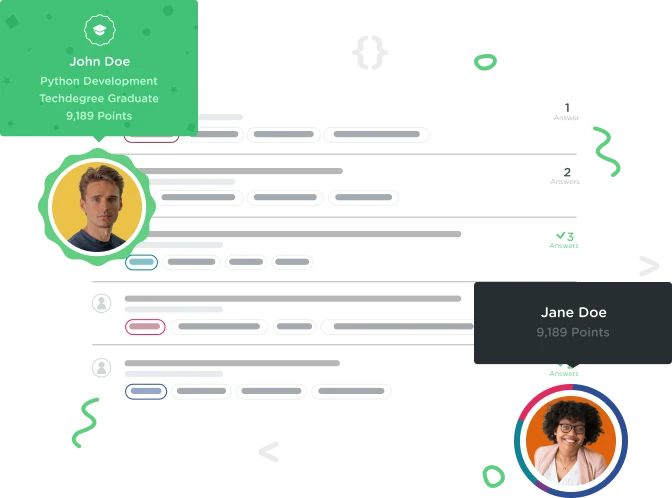

Kyle Baker
1,884 PointsI hoping someone can walk me through each step of the "ArrayLists" challenge.
The Question:
Let's add a method that returns a list of all links in the body of the document. All words that start with http should be considered links. Name it getExternalLinks.
NOTE: Remember to import the needed classes from java.util. HINT: We already made the getWords method, we should use it!
The Answer:
import java.util.ArrayList;
import java.util.List;
public List<String> getExternalLinks() {
List<String> results = new ArrayList<String>();
for (String word : getWords()) {
if (word.startsWith("http")) {
results.add(word);
}
}
return results;
}
I don't fully understand this:
List<String> results = new ArrayList<String>();
Why is String being put into angle brackets next to List and ArrayList? Why are they being set equal to each other? Why does List<String> have to be before results. I guess I understand close to none of it.
As for this:
for (String word : getWords())
Is it saying for each 'word' it's going to do this getWords(), which means it's just going to separate the words? How does that work if 'word' isn't set to anything? How does it know what the words are? As you can see I'm pretty confused...
1 Answer

Cameron Lasky
109 PointsList<String> results = new ArrayList<String>();
Why is String being put into angle brackets next to List and ArrayList?
Java is a strongly typed language meaning that the different objects and variables you use all need to be defined with the specific type they are. In this example you are declaring that you have a List that contains String objects (List<String>). This is how we declare a new list we always need to define the type of list we are creating. For example if I have List<Integer> I have a List that contains Integers.
List is a generic type there are multiple types of lists in java one introduced in this example is the ArrayList. So when you define you have a List of Strings you then need to define what kind of list you have (ArrayList). Once again when you specify that you have an ArrayList you need to define the type of object in your ArrayList hence the <String> after. (Note: In later versions of Java the second <String> after the ArrayList is not required all you need is <> eg: ArrayList<>())
Why are they being set equal to each other? Why does List have to be before results. I guess I understand close to none of it.
You are not setting them equal to each other. The = operator does not denote equality in this instance it denotes an assignment. So what you are doing here is creating a new List of Strings and calling it results. You are then assigning the specific type of List (ArrayList) to your results variable. You then should be able to reference your newly created List object (results) later on in your code by using the results variable.
This is all just the syntax involved in declaring a new ArrayList.
for (String word : getWords())
Is it saying for each 'word' it's going to do this getWords(), which means it's just going to separate the words? How does that work if 'word' isn't set to anything? How does it know what the words are?
It appears that the getWords() function is going to return a List of Strings. You can think of this list as follows: [red, blue, green, yellow, black] they are all separate words in the list so no separating is going on. However you are kind of on the right track here. What this will do is for each word in your list of words you'll then loop. Each word in the list in turn will then be stored in the word variable. (eg. First time through loop word = red, Second time through loop word = blue, Third time through loop word = green....)
Kyle Baker
1,884 PointsKyle Baker
1,884 PointsGotcha! This helps a lot! Thanks for your patience.
This also helped me understand the 'type parameters' or the word in the angle brackets < >:
Type parameters are analogous to the ordinary parameters used in methods or constructors. Much like a method has formal value parameters that describe the kinds of values it operates on, a generic declaration has formal type parameters. When a method is invoked, actual arguments are substituted for the formal parameters, and the method body is evaluated. When a generic declaration is invoked, the actual type arguments are substituted for the formal type parameters.