Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial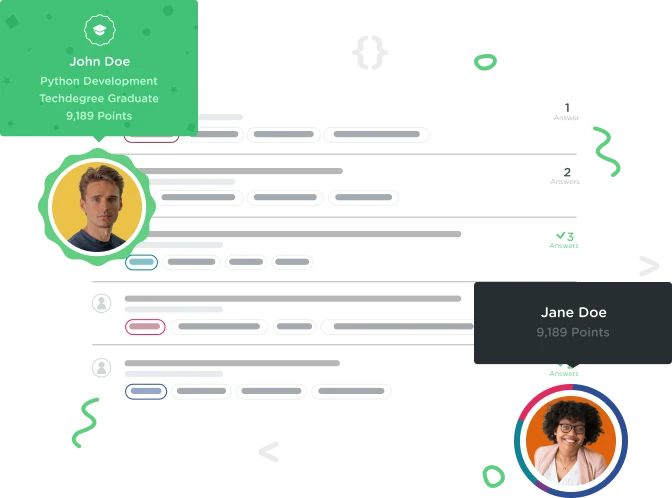
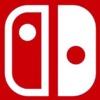
Cross Ender
9,567 PointsI just can't figure this out, either.
Trying to get the CapitilismHand.reroll() class method to work, but it won't. Please, I really want to finish this course, and not being able to figure out the last possible moment feels like torture.
from dice import D6
class Hand(list):
def __init__(self, size=0, die_class=None, *args, **kwargs):
if not die_class:
raise ValueError("You must provide a die class")
super().__init__()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand):
def __init__(self):
super().__init__(size=2, die_class=D6)
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
@property
def doubles(self):
double = False
for dicty in self._sets.items():
if dicty[1] == 2:
double = True
return double
@classmethod
def reroll(cls, size, die_class):
hand = []
for _ in range(size):
hand.append(die_class)
return cls(hand)
3 Answers

James Shi
8,942 PointsA class method's first argument is the class the method belongs to. In this case, cls is CapitalismHand, not Hand. The reroll method should return a new instance of the CapitalismHand class, so it doesn't need any arguments.
@classmethod
def reroll(cls):
return cls()

David Welsh
9,298 PointsI had a lot of issues on this as well, and was directed to this stack overflow answer which broke it down for me. At first I thought, nah, no way it's that easy. Just don't overcomplicate it. https://stackoverflow.com/questions/13260557/create-new-class-instance-from-class-method

Matthew Earlywine
3,508 PointsWhy is this not passing the challenge? '''Python from dice import D6
class Hand(list): def init(self, size=0, die_class=None, *args, **kwargs): if not die_class: raise ValueError("You must provide a die class") super().init()
for _ in range(size):
self.append(die_class())
self.sort()
def _by_value(self, value):
dice = []
for die in self:
if die == value:
dice.append(die)
return dice
class CapitalismHand(Hand): def init(self, *args, **kwargs): super().init(size=2, die_class=D6, *args, **kwargs)
@property
def doubles(self):
if self[0] == self[1]:
return True
@classmetod
def reroll(cls):
return (cls)
@property
def ones(self):
return self._by_value(1)
@property
def twos(self):
return self._by_value(2)
@property
def threes(self):
return self._by_value(3)
@property
def fours(self):
return self._by_value(4)
@property
def fives(self):
return self._by_value(5)
@property
def sixes(self):
return self._by_value(6)
@property
def _sets(self):
return {
1: len(self.ones),
2: len(self.twos),
3: len(self.threes),
4: len(self.fours),
5: len(self.fives),
6: len(self.sixes)
}
'''

Steven Hender
5,830 PointsThe above code has a typo on the "@classmetod" line It should be "@classmethod" Note the missing "h"