Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial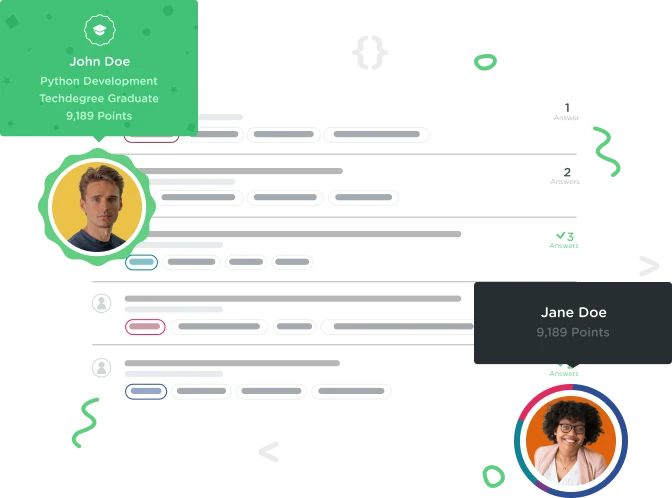
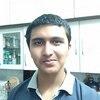
Aditya Sheode
1,291 PointsI just dont understand how the two different load methods are working
public void load () { load(MAX_PEZ); }
public void load (int pezAmount) { mPezCount += pezAmount; }
How exactly is the original method referring to the new one please help!
19 Answers
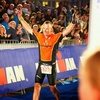
Steve Hunter
57,712 PointsOK. You can use the method with the parameter like this:
load(1); // add one to mPezCount
load(2); // add 2
load(MAX_PEZ); // guess what ;)
The method that takes no parameter does the same job as the last line above. But it does it inside the method. The job of that method is to fill up the PEZ dispenser, i.e. add MAX_PEZ tomPezCount
.
Because you might want to do this a lot, the method without a parameter creates a shortcut to do it. So rather than typing:
load(MAX_PEZ);
You can just use:
load();
Inside the non-parametered method, you'll see the call to the parameter method:
public void load(){
load(MAX_PEZ);
}
So, the method calls the last line of the code I started with but does that inside the method meaning you can just use load()
to max the PEZ out, rather than load(MAX_PEZ)
.
Better?
Steve.
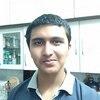
Aditya Sheode
1,291 PointsOHHH so .. public void load () { load(MAX_PEZ); // This is referring to the second method?? i didnt know that simple brackets played such a huge role..
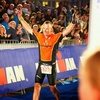
Steve Hunter
57,712 PointsYes, two methods:
// no parameters
public void load(){
load(MAX_PEZ);
}
// with a parameter
public void load(int aParam){
mPezCount += aParam;
}
So the first method doesn't take a parameter but uses the second method which does take a parameter, defaulting its value to MAX_PEZ
.
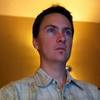
ryanjones6
13,797 PointsFirst Question:
Yes the Java Compiler knows if a method is Overloaded. If you don't understand Overloading. Use this site: http://beginnersbook.com/2013/05/method-overloading/ - This site explains Java in simple terms. Plus the examples are great.
Second Question:
A parameter is placed within the parenthesis of a method:
public void load () { ... } // no parameters
public void load (int pezAmount) { ... } // int pezAmount is a parameter
An argument is the syntax within the body(scope) of the method
public void load () {
//this is an argument, it is calling the second overloaded method.
load(MAX_PEZ);
}
public void load (int pezAmount) {
// this is an argument the uses the parameter pezAmount
mPezCount += pezAmount;
}
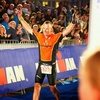
Steve Hunter
57,712 PointsEmoji cheat sheet - here
Steve.
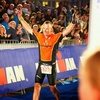
Steve Hunter
57,712 PointsHi Aditya,
There are two methods. One takes a parameter, the other does not.
The one that takes a parameter allows you to pass in a value. This value is added to mPezCount
.
The method that takes no parameter defaults the value passed to the method above. So, rather than adding a user-defined amount to mPezCount
, it uses MAX_PEZ. To do this, it passes MAX_PEZ as the parameter to the first method.
So, the second method uses the first by passing the constant, MAX_PEZ, as the parameter in the first method.
Make sense?
Steve.
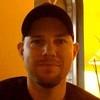
Jeremy Hill
29,567 PointsAditya,
I understand that this can be a little confusing for beginners so I'm going to back up just a little.
A method is a set of instructions for the computer to run when the method is called. The method can be given any name with some restrictions. The parameters are the variables inside of the parenthesis in your method heading like this:
public void addNumbers(int num1, int num2);
The variables inside the parenthesis (parameters) are presumed to be used somewhere within the method code block (between the curly braces - '{}'). That means whatever the variables are will be passed in when the method gets called: this is information needed for the method to operate. Just like when you make a phone call- in order for the connection to be made a number has to passed in. So with the method I created above you can see that it will add numbers because of the name ("addNumbers") and if you notice the two variables in the parenthesis that tells me that the method needs two integers to complete its operation- which means that this method relies on information from somewhere- either a user or another part of the program.
So if I have the method: public void load() This tells me that it doesn't return anything (because of return type - "void") and it doesn't take any parameters (nothing inside of the parenthesis). This means that the method already has everything it needs to operate. Inside the method code block curly braces ("{}") it has the set of instructions to operate. The load() method may just need to increment the pez count by one each time it is called or they may want the pez to be topped off each time the method is called.
In this case the first load() method that doesn't take a parameter basically tops of the pez (fills it up to max). The second load() method that does take a parameter: public void load(int amount) allows the user/program to add in a specific amount that is different than the initial max amount.
Why is it done this way you might ask? Well say you don't have enough pez to fill up the entire container but you might have say four pieces; you can add the four pieces instead of adding max. If you don't have max and you try to fill it up anyway it could throw an error of some sort. So this other method (the one with the parameter) allows us to pass in the specific amount.
Method signatures allows us to create the same type of method using different variables that are to be passed in. To give you an extensive idea of method signatures I will do a few more:
public void addNumbers(){
}
public void addNumbers(int x){
}
public void addNumbers(int x, int y){
}
public void addNumbers(String name, int x, y){
}
All these are legal to use because they all have different signatures (number of variables in the parenthesis).
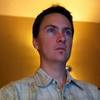
ryanjones6
13,797 PointsYes, those methods all work, except the last method you forgot to declare the data type for y, but that is just a typo.
public void addNumbers(String name, int x, y){ } // missing data type declaration for parameter y
public void addNumbers(String name, int x, int y){ }// data type for parameter y set to int
Besides TeamTreehouse's Workspace, have you tried using and IDE such as: Netbeans, IntelliJ, or Eclipse?
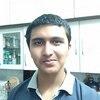
Aditya Sheode
1,291 Pointsso do you mean that by just calling the methods the compiler clubs them? and btw what is the difference between a parameter and an argument?
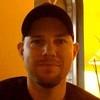
Jeremy Hill
29,567 PointsAs long as you have varying arguments the compiler will know which one is being referred to.
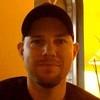
Jeremy Hill
29,567 PointsI never replied to your parameter/argument question: Parameters are basically the variables needed in the parenthesis in your method heading. Arguments are what is passed in when the method is called. They are basically the same thing in that they are what allows information to be passed in to your method for execution. So if I give the load() method one parameter like so: load(int x), this means that when the method is called the user/program will have to pass in exactly one argument in order for the method to run. If you only have one load() method and it has parameters and you try calling the method without the corresponding number of arguments your compiler will throw some errors at you. I hope this make sense.
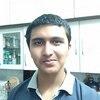
Aditya Sheode
1,291 Pointsokay and my second question please... (how do you use emojis in here anyway?)
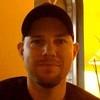
Jeremy Hill
29,567 PointsI'm not sure, I usually don't use them; maybe someone can enlighten us both on that one.
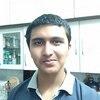
Aditya Sheode
1,291 Points steve hunter
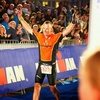
Steve Hunter
57,712 Points
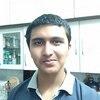
Aditya Sheode
1,291 Pointsi still am not getting his code how does max pez refrence to the second load method??? please help
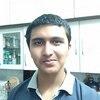
Aditya Sheode
1,291 Pointsplease explain it me as if im 5.. im going over the concept again...
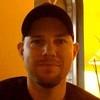
Jeremy Hill
29,567 PointsYes it should have the type int next to it, my bad. If you are asking me I have all three IDEs.
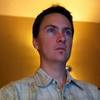
ryanjones6
13,797 PointsYes, I was asking which and if you had installed any IDE. I found best way to learn about Overloading is to test out the concept in an IDE. I prefer Netbeans and IntelliJ. Try following the tutorials on the site BeginnersBook - http://beginnersbook.com/2013/05/method-overloading/ -- After you complete a sample. Break it up by Createing a class called Main with the content from public static void main(String[] args)
public class Main{
public static void main(String[] args){
}
}
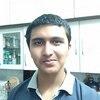
Aditya Sheode
1,291 PointsHello Guys I cant thank you all enough! i'm very happy that i have such excellent classmates... AGain thank you for taking the pains to explain this to me..
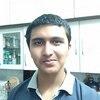
Aditya Sheode
1,291 PointsJeremy Thank you very much your detailed explaination made it very clear.. Craig tends to you know just say things and just move on quickly you know.. but All of you I just cant thank you enough!
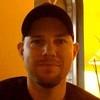
Jeremy Hill
29,567 PointsYou are very welcome. I understand he does move through it fairly quickly. I took Java programming in college so I already have some prior knowledge in it.
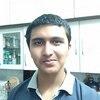
Aditya Sheode
1,291 PointsI'm definitely gonna try another ide.. My net connection refreshes on the 9th august which ide would you recommend for me? NetBeans?
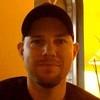
Jeremy Hill
29,567 PointsI do like netbeans but my favorite is Eclipse, though I am warming up to IntelliJ idea.
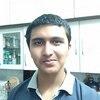
Aditya Sheode
1,291 PointsYeah but eclipse is like really big isn't it?
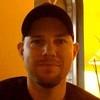
Jeremy Hill
29,567 PointsYou mean like it takes up a lo of memory?
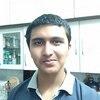
Aditya Sheode
1,291 Pointsyes :( it hogs memory like popcorn
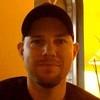
Jeremy Hill
29,567 PointsI'm not sure how much more it uses but it runs fine on my desktop.
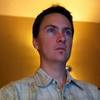
ryanjones6
13,797 PointsIt's good to know at least Eclipse and Netbeans. Both can be found within the industry if your focus is to become a Java Developer. I prefer Netbeans over Eclipse because I found it easier to import files. IntelliJ is good to know because Android Studio built their IDE using the JetBrains (intelliJ) framework.
Jeremy Hill
29,567 PointsJeremy Hill
29,567 PointsIt looks like the first load() method signature takes zero parameters and inside its code block calls the load() method that does take an argument and passes in the max amount of pez.
It appears that this lesson is teaching you about method signatures; you can have multiple methods with the same name as long as the arguments are different. And in this example they have it set up where one of the load() methods calls the other load() method (with different arguments).
So they have two methods at work here, both of which have the same name but different arguments:
The first load() method doesn't take any parameters and basically has it set to load the pez dispenser full; it's kind of like the default. The second load() method allows the user/program to pass in a specific number of pez pieces to load. instead of writing a whole new method to do a max load they just call the original load() method and pass in the max value.