Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial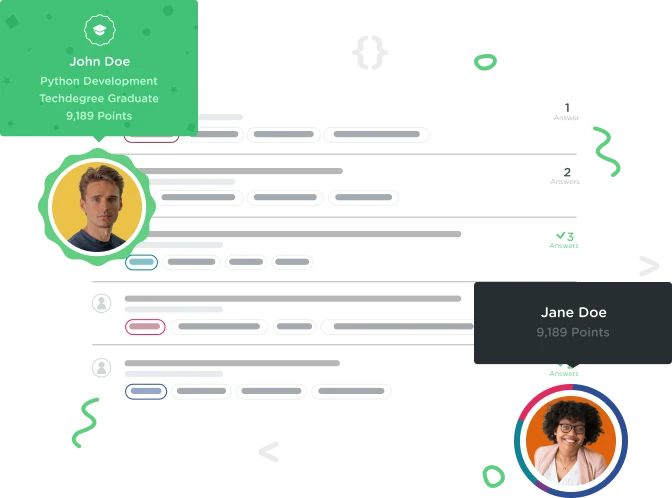

Graham O Connor
6,551 Pointsi just want to know where is the mistake in this code
<!DOCTYPE html> <html> <head> <title>Treetunes</title> <link rel="stylesheet" href="style2.css"/> </head>
<body>
<div>
<h1>Treetunes</h1>
<ol id="playlist">
</ol>
<button id="play">Play</button>
<button id="next">Next</button>
<button id="stop">Stop</button>
</div>
<script src = "playlist1.js" ></script>
<script src = "media.js"></script>
<script src = "movie.js"></script>
<script src ="song.js"></script>
<script src ="app1.js"></script>
</body>
</html>
function Playlist() { this.songs = []; this.nowPlayingIndex = 0
}
Playlist.prototype.add = function(song) { this.songs.push(song); };
Playlist.prototype.play = function() { var currentSong = this.songs[this.nowPlayingIndex]; currentSong.play();
};
Playlist.prototype.stop = function(){ var currentSong = this.songs[this.nowPlayingIndex]; currentSong.stop();
};
Playlist.prototype.next = function() { this.stop(); this.nowPlayingIndex++; if(this.nowPlayingIndex === this.songs.length){ this.nowPlayngindex = 0; }
this.play();
};
Playlist.prototype.renderInElement = function(list) { list.innerHTML = "";
for(var i = 0; i<this.songs.length; i++){
list.innerHTML += this.songs[i].toHTML();
}
};
function Media(title, duration ) { this.title = title; this.duration = duration; this.isPlaying = false; }
Media.prototype.play = function() {
this.isPlaying = true;
};
Media.prototype.stop = function() {
this.isPlaying = false;
};
function Song(title, artist ,duration ) { Mecia.call(this, title, duration); this.artist = artist;
}
Song.prototype = Object.create(Media.prototype);
Song.prototype.toHTML = function() { var htmlString = '<li'; if(this.isPlaying){
htmlString +='class="current"';
}
htmlString +='>'; htmlstring +=this.title; htmlString += '-'; htmlString +=this.artist; htnlString +='<span class="duration">'; htmlString += this.duration; htmlString += '</span></li>';
};
var hereComesTheSun = new Song("Here comes the sun", "The Beatles", "2:54"); var walkingOnSunshine = new Song("Walking on sunshine","Katrina and the Waves","3:43");
var manOfSteel = new Movie("Man of Steel", 2013, "2:30:00");
playlist.add(hereComesTheSun); playlist.add(walkingOnSunShine);
playlist.add(manOfSteel);
var playListElement = document.getElementById("playlist");
playlist.renderInElement(playListElement);
var playButton = document.getElementById("play"); playButton.onclick = function(){
playlist.play();
playlist.renderInElement(playListElement);
} var nextButton = document.getElementById("next"); nextButton.onclick = function(){
playlist.next();
playlist.renderInElement(playListElement);
} var stopButton = document.getElementById("stop"); stopButton.onclick = function(){
playlist.stop();
playlist.renderInElement(playListElement);
}
function Movie(title, artist ,duration ) { Mecia.call(this, title, duration); this.artist = artist;
}
Movie.prototype = Object.create(Media.prototype);
Movie.prototype.toHTML = function() { var htmlString = '<li'; if(this.isPlaying){
htmlString +='class="current"';
}
htmlString +='>'; htmlstring +=this.title; htmlString += ' ('; htmlString +=this.year; htmlString += ') '; htnlString +='<span class="duration">'; htmlString += this.duration; htmlString += '</span></li>';
};
1 Answer

Simon Coates
28,694 PointshtnlString ? I don't know if that's the only error. People will be more inclined to debug if you post a workspace snapshot (see https://teamtreehouse.com/library/previews-and-snapshots ) or use the markdown syntax so code displays properly. Another spelling mistake is nowPlayngindex.
It may also punish you for walkingOnSunShine as opposed to walkingOnSunshine. I think case may matter in javascript.
you also seem to have a reference to Mecia not Media.
with the movie,
this.artist = artist;
should probably be
this.year = year;
You also need to change the movie function definition so that the method expects year.