Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial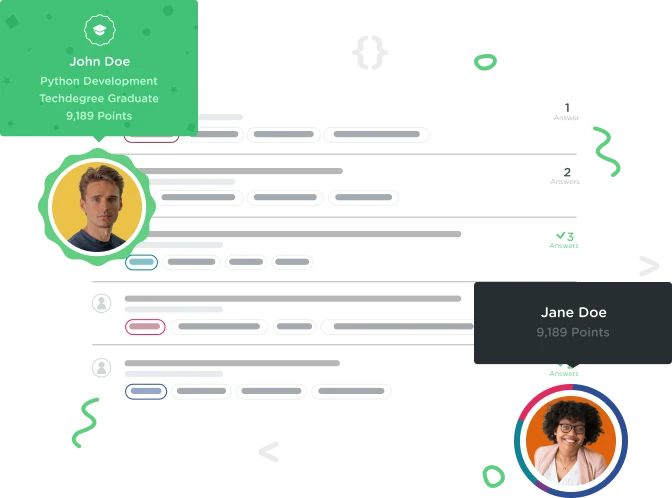

Colin Senfelds
1,427 PointsI keep experiencing an odd "Bummer" message in Swift 2.0 Collections and Control Flow.
The problem appears to be in challenge task 2 of 4. On task 1, the code works without issue and takes me to task 2, where if I enter incorrect code, the system will inform me that I made an error. However, when the code appears to be correct and I submit it for checking, I am given the message that task 1 is no longer passing. If I go back, change nothing (or only remove ": [Int]”), then resubmit, I am again granted access to task 2. As a note, I am only experiencing issues through the Team TreeHouse site. Xcode has no issue returning the final array. Am I just not writing this code in the best way or am I misinterpreting the dirtections? Thanks for you help! Colin
// Enter your code below
var arrayOfInts: [Int] = [1,
3,
5,
7,
11,
13]
arrayOfInts.append(17)
arrayOfInts += [19,
23]
1 Answer
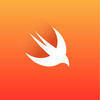
Steven Deutsch
21,046 PointsHey Colin Senfelds,
The challenge wants you to start with a total of 6 items in your array. In task 2 you need to add two more items to the array, using two different methods. The first item you will add with the append() method. The second item you will add by concatenating an array. I completed the code challenge and broke down my code step by step so you can see what the challenge is asking. The reason why your code wasn't passing was possibly because you were adding too many values with your array concatenation or you had more than 6 items in the original array.
// Enter your code below
// Create an array of 6 Ints
var arrayOfInts = [1, 2, 4, 5, 7, 10]
// Add item to array using append() method
arrayOfInts.append(43)
// Add item to the array by concatenating an array
arrayOfInts += [27]
/* Retrieve item 5 from index, and assign result
to a constant named value */
/* Array indexes start at 0 so to access the
5th item we use an index of 4 */
let value = arrayOfInts[4]
/* Remove the 6th item from the array.
We can do this using removeAtIndex()
The removeAtIndex returns the removed value,
so we can assign the value to a variable/constant */
let discardedValue = arrayOfInts.removeAtIndex(5)
Good Luck!
Colin Senfelds
1,427 PointsColin Senfelds
1,427 PointsThanks Steven! It turns out the problem all along was that, in task 2, the system wanted me to concatinate an array containing specifically only 1 integer. I suppose the "Bummer" message explaining that task 1 didn't pass really was a bug. Task 2 is where my problem code was.