Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial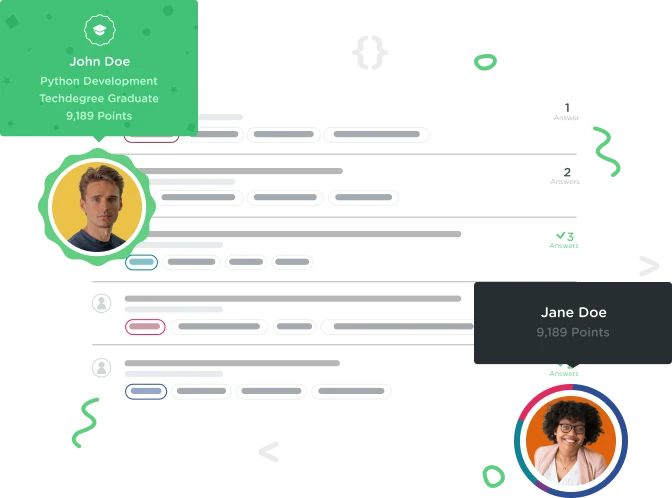
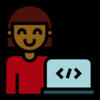
Renee Rooche
2,469 PointsI keep getting 3 out 5 when I answered everything correctly
/*
- Store correct answers
- When quiz begins, no answers are correct */ let correct = 0;
// 2. Store the rank of a player let rank;
// 3. Select the <main> HTML element const main = document.querySelector('main');
/*
- Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers / let question1 = prompt("What is the last letter of the alphabet?"); if ( question1.toLowerCase() === "z") { correct += 1; } let question2 = prompt("What is the color of the sky"); if ( question2.toLowerCase() === "blue") { correct += 1; } let question3 = prompt("Where is Six Flags located?"); if ( question3.toUpperCase() === "New Jersey" ) { correct += 1; } let question4 = prompt("What is the speed limit in NY?"); if ( question4 === 55) { correct += 1; } let question5 = prompt("What is the capitol of Jamaica?"); if (question4.toUpperCase() === "KINGSTON") { correct +=1; } /
- Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown */ if (correct === 5) { rank = "Gold"; } else if (correct = 3 || 4) { rank = "Silver"; } else if (correct = 1 || 2) { rank = "Bronze"; } else { rank = "No Crown"; }
// 6. Output results to the <main> element
main.innerHTML =
<h2>You got ${correct} out of 5.</h2>
<p> Crown earned : <strong>${rank}</strong></p>
;
1 Answer
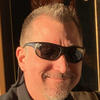
Peter Vann
36,427 PointsHi Renee!
else if (correct = 3 || 4)
Won't work.
First you need == (equaility operator) or === (strict equality operator) not =
(= is the assignment operator)
Second, you are using || incorrectly.
You would need to do something more like this:
else if (correct === 3 || correct === 4)
And
else if (correct === 1 || correct === 2)
But I still think it's better to use > for 3/4 and 1/2 like this:
if (correct === 5) {
rank = "Gold";
} else if (correct >= 3) {
rank = "Silver";
} else if (correct >= 1) {
rank = "Bronze";
} else {
rank = "No Crown";
}
Or
if (correct === 5) {
rank = "Gold";
} else if (correct > 2 {
rank = "Silver";
} else if (correct > 0) {
rank = "Bronze";
} else {
rank = "No Crown";
}
Just my opinion...
Your way, once corrected, should work just fine, though (and actually is more readable and more helpful to see what the real intended logic is, to be honest)...
I hope that helps.
Stay safe and happy coding!
Renee Rooche
2,469 PointsRenee Rooche
2,469 PointsThank you! That makes a lot of sense.